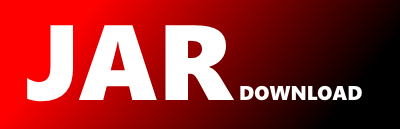
aws.sdk.kotlin.services.codebuild.model.StartBuildBatchRequest.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of codebuild Show documentation
Show all versions of codebuild Show documentation
The AWS SDK for Kotlin client for CodeBuild
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.codebuild.model
class StartBuildBatchRequest private constructor(builder: Builder) {
/**
* An array of ProjectArtifacts objects that contains information about the
* build output artifact overrides for the build project.
*/
val artifactsOverride: aws.sdk.kotlin.services.codebuild.model.ProjectArtifacts? = builder.artifactsOverride
/**
* A BuildBatchConfigOverride object that contains batch build configuration
* overrides.
*/
val buildBatchConfigOverride: aws.sdk.kotlin.services.codebuild.model.ProjectBuildBatchConfig? = builder.buildBatchConfigOverride
/**
* Overrides the build timeout specified in the batch build project.
*/
val buildTimeoutInMinutesOverride: kotlin.Int? = builder.buildTimeoutInMinutesOverride
/**
* A buildspec file declaration that overrides, for this build only, the latest one
* already defined in the build project.
* If this value is set, it can be either an inline buildspec definition, the path to an
* alternate buildspec file relative to the value of the built-in
* CODEBUILD_SRC_DIR environment variable, or the path to an S3 bucket.
* The bucket must be in the same Amazon Web Services Region as the build project. Specify the buildspec
* file using its ARN (for example,
* arn:aws:s3:::my-codebuild-sample2/buildspec.yml). If this value is not
* provided or is set to an empty string, the source code must contain a buildspec file in
* its root directory. For more information, see Buildspec File Name and Storage Location.
*/
val buildspecOverride: kotlin.String? = builder.buildspecOverride
/**
* A ProjectCache object that specifies cache overrides.
*/
val cacheOverride: aws.sdk.kotlin.services.codebuild.model.ProjectCache? = builder.cacheOverride
/**
* The name of a certificate for this batch build that overrides the one specified in the batch build
* project.
*/
val certificateOverride: kotlin.String? = builder.certificateOverride
/**
* The name of a compute type for this batch build that overrides the one specified in the
* batch build project.
*/
val computeTypeOverride: aws.sdk.kotlin.services.codebuild.model.ComputeType? = builder.computeTypeOverride
/**
* Specifies if session debugging is enabled for this batch build. For more information, see
* Viewing a running build in Session Manager. Batch session debugging is not supported for matrix batch builds.
*/
val debugSessionEnabled: kotlin.Boolean? = builder.debugSessionEnabled
/**
* The Key Management Service customer master key (CMK) that overrides the one specified in the batch build
* project. The CMK key encrypts the build output artifacts.
* You can use a cross-account KMS key to encrypt the build output artifacts if your
* service role has permission to that key.
* You can specify either the Amazon Resource Name (ARN) of the CMK or, if available, the CMK's alias (using
* the format alias/).
*/
val encryptionKeyOverride: kotlin.String? = builder.encryptionKeyOverride
/**
* A container type for this batch build that overrides the one specified in the batch build
* project.
*/
val environmentTypeOverride: aws.sdk.kotlin.services.codebuild.model.EnvironmentType? = builder.environmentTypeOverride
/**
* An array of EnvironmentVariable objects that override, or add to, the
* environment variables defined in the batch build project.
*/
val environmentVariablesOverride: List? = builder.environmentVariablesOverride
/**
* The user-defined depth of history, with a minimum value of 0, that overrides, for this
* batch build only, any previous depth of history defined in the batch build project.
*/
val gitCloneDepthOverride: kotlin.Int? = builder.gitCloneDepthOverride
/**
* A GitSubmodulesConfig object that overrides the Git submodules configuration
* for this batch build.
*/
val gitSubmodulesConfigOverride: aws.sdk.kotlin.services.codebuild.model.GitSubmodulesConfig? = builder.gitSubmodulesConfigOverride
/**
* A unique, case sensitive identifier you provide to ensure the idempotency of the
* StartBuildBatch request. The token is included in the
* StartBuildBatch request and is valid for five minutes. If you repeat
* the StartBuildBatch request with the same token, but change a parameter,
* CodeBuild returns a parameter mismatch error.
*/
val idempotencyToken: kotlin.String? = builder.idempotencyToken
/**
* The name of an image for this batch build that overrides the one specified in the batch
* build project.
*/
val imageOverride: kotlin.String? = builder.imageOverride
/**
* The type of credentials CodeBuild uses to pull images in your batch build. There are two valid
* values:
* CODEBUILD
* Specifies that CodeBuild uses its own credentials. This requires that you
* modify your ECR repository policy to trust CodeBuild's service principal.
* SERVICE_ROLE
* Specifies that CodeBuild uses your build project's service role.
* When using a cross-account or private registry image, you must use
* SERVICE_ROLE credentials. When using an CodeBuild curated image,
* you must use CODEBUILD credentials.
*/
val imagePullCredentialsTypeOverride: aws.sdk.kotlin.services.codebuild.model.ImagePullCredentialsType? = builder.imagePullCredentialsTypeOverride
/**
* Enable this flag to override the insecure SSL setting that is specified in the batch build
* project. The insecure SSL setting determines whether to ignore SSL warnings while
* connecting to the project source code. This override applies only if the build's source
* is GitHub Enterprise.
*/
val insecureSslOverride: kotlin.Boolean? = builder.insecureSslOverride
/**
* A LogsConfig object that override the log settings defined in the batch build
* project.
*/
val logsConfigOverride: aws.sdk.kotlin.services.codebuild.model.LogsConfig? = builder.logsConfigOverride
/**
* Enable this flag to override privileged mode in the batch build project.
*/
val privilegedModeOverride: kotlin.Boolean? = builder.privilegedModeOverride
/**
* The name of the project.
*/
val projectName: kotlin.String? = builder.projectName
/**
* The number of minutes a batch build is allowed to be queued before it times out.
*/
val queuedTimeoutInMinutesOverride: kotlin.Int? = builder.queuedTimeoutInMinutesOverride
/**
* A RegistryCredential object that overrides credentials for access to a
* private registry.
*/
val registryCredentialOverride: aws.sdk.kotlin.services.codebuild.model.RegistryCredential? = builder.registryCredentialOverride
/**
* Set to true to report to your source provider the status of a batch build's
* start and completion. If you use this option with a source provider other than GitHub,
* GitHub Enterprise, or Bitbucket, an invalidInputException is thrown.
* The status of a build triggered by a webhook is always reported to your source
* provider.
*/
val reportBuildBatchStatusOverride: kotlin.Boolean? = builder.reportBuildBatchStatusOverride
/**
* An array of ProjectArtifacts objects that override the secondary artifacts
* defined in the batch build project.
*/
val secondaryArtifactsOverride: List? = builder.secondaryArtifactsOverride
/**
* An array of ProjectSource objects that override the secondary sources
* defined in the batch build project.
*/
val secondarySourcesOverride: List? = builder.secondarySourcesOverride
/**
* An array of ProjectSourceVersion objects that override the secondary source
* versions in the batch build project.
*/
val secondarySourcesVersionOverride: List? = builder.secondarySourcesVersionOverride
/**
* The name of a service role for this batch build that overrides the one specified in the
* batch build project.
*/
val serviceRoleOverride: kotlin.String? = builder.serviceRoleOverride
/**
* A SourceAuth object that overrides the one defined in the batch build
* project. This override applies only if the build project's source is BitBucket or
* GitHub.
*/
val sourceAuthOverride: aws.sdk.kotlin.services.codebuild.model.SourceAuth? = builder.sourceAuthOverride
/**
* A location that overrides, for this batch build, the source location defined in
* the batch build project.
*/
val sourceLocationOverride: kotlin.String? = builder.sourceLocationOverride
/**
* The source input type that overrides the source input defined in the batch
* build project.
*/
val sourceTypeOverride: aws.sdk.kotlin.services.codebuild.model.SourceType? = builder.sourceTypeOverride
/**
* The version of the batch build input to be built, for this build only. If not specified,
* the latest version is used. If specified, the contents depends on the source
* provider:
* CodeCommit
* The commit ID, branch, or Git tag to use.
* GitHub
* The commit ID, pull request ID, branch name, or tag name that corresponds
* to the version of the source code you want to build. If a pull request ID is
* specified, it must use the format pr/pull-request-ID (for
* example pr/25). If a branch name is specified, the branch's
* HEAD commit ID is used. If not specified, the default branch's HEAD commit
* ID is used.
* Bitbucket
* The commit ID, branch name, or tag name that corresponds to the version of
* the source code you want to build. If a branch name is specified, the
* branch's HEAD commit ID is used. If not specified, the default branch's HEAD
* commit ID is used.
* Amazon S3
* The version ID of the object that represents the build input ZIP file to
* use.
* If sourceVersion is specified at the project level, then this
* sourceVersion (at the build level) takes precedence.
* For more information, see Source Version Sample
* with CodeBuild in the CodeBuild User Guide.
*/
val sourceVersion: kotlin.String? = builder.sourceVersion
companion object {
operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.codebuild.model.StartBuildBatchRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("StartBuildBatchRequest(")
append("artifactsOverride=$artifactsOverride,")
append("buildBatchConfigOverride=$buildBatchConfigOverride,")
append("buildTimeoutInMinutesOverride=$buildTimeoutInMinutesOverride,")
append("buildspecOverride=$buildspecOverride,")
append("cacheOverride=$cacheOverride,")
append("certificateOverride=$certificateOverride,")
append("computeTypeOverride=$computeTypeOverride,")
append("debugSessionEnabled=$debugSessionEnabled,")
append("encryptionKeyOverride=$encryptionKeyOverride,")
append("environmentTypeOverride=$environmentTypeOverride,")
append("environmentVariablesOverride=$environmentVariablesOverride,")
append("gitCloneDepthOverride=$gitCloneDepthOverride,")
append("gitSubmodulesConfigOverride=$gitSubmodulesConfigOverride,")
append("idempotencyToken=$idempotencyToken,")
append("imageOverride=$imageOverride,")
append("imagePullCredentialsTypeOverride=$imagePullCredentialsTypeOverride,")
append("insecureSslOverride=$insecureSslOverride,")
append("logsConfigOverride=$logsConfigOverride,")
append("privilegedModeOverride=$privilegedModeOverride,")
append("projectName=$projectName,")
append("queuedTimeoutInMinutesOverride=$queuedTimeoutInMinutesOverride,")
append("registryCredentialOverride=$registryCredentialOverride,")
append("reportBuildBatchStatusOverride=$reportBuildBatchStatusOverride,")
append("secondaryArtifactsOverride=$secondaryArtifactsOverride,")
append("secondarySourcesOverride=$secondarySourcesOverride,")
append("secondarySourcesVersionOverride=$secondarySourcesVersionOverride,")
append("serviceRoleOverride=$serviceRoleOverride,")
append("sourceAuthOverride=$sourceAuthOverride,")
append("sourceLocationOverride=$sourceLocationOverride,")
append("sourceTypeOverride=$sourceTypeOverride,")
append("sourceVersion=$sourceVersion)")
}
override fun hashCode(): kotlin.Int {
var result = artifactsOverride?.hashCode() ?: 0
result = 31 * result + (buildBatchConfigOverride?.hashCode() ?: 0)
result = 31 * result + (buildTimeoutInMinutesOverride ?: 0)
result = 31 * result + (buildspecOverride?.hashCode() ?: 0)
result = 31 * result + (cacheOverride?.hashCode() ?: 0)
result = 31 * result + (certificateOverride?.hashCode() ?: 0)
result = 31 * result + (computeTypeOverride?.hashCode() ?: 0)
result = 31 * result + (debugSessionEnabled?.hashCode() ?: 0)
result = 31 * result + (encryptionKeyOverride?.hashCode() ?: 0)
result = 31 * result + (environmentTypeOverride?.hashCode() ?: 0)
result = 31 * result + (environmentVariablesOverride?.hashCode() ?: 0)
result = 31 * result + (gitCloneDepthOverride ?: 0)
result = 31 * result + (gitSubmodulesConfigOverride?.hashCode() ?: 0)
result = 31 * result + (idempotencyToken?.hashCode() ?: 0)
result = 31 * result + (imageOverride?.hashCode() ?: 0)
result = 31 * result + (imagePullCredentialsTypeOverride?.hashCode() ?: 0)
result = 31 * result + (insecureSslOverride?.hashCode() ?: 0)
result = 31 * result + (logsConfigOverride?.hashCode() ?: 0)
result = 31 * result + (privilegedModeOverride?.hashCode() ?: 0)
result = 31 * result + (projectName?.hashCode() ?: 0)
result = 31 * result + (queuedTimeoutInMinutesOverride ?: 0)
result = 31 * result + (registryCredentialOverride?.hashCode() ?: 0)
result = 31 * result + (reportBuildBatchStatusOverride?.hashCode() ?: 0)
result = 31 * result + (secondaryArtifactsOverride?.hashCode() ?: 0)
result = 31 * result + (secondarySourcesOverride?.hashCode() ?: 0)
result = 31 * result + (secondarySourcesVersionOverride?.hashCode() ?: 0)
result = 31 * result + (serviceRoleOverride?.hashCode() ?: 0)
result = 31 * result + (sourceAuthOverride?.hashCode() ?: 0)
result = 31 * result + (sourceLocationOverride?.hashCode() ?: 0)
result = 31 * result + (sourceTypeOverride?.hashCode() ?: 0)
result = 31 * result + (sourceVersion?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as StartBuildBatchRequest
if (artifactsOverride != other.artifactsOverride) return false
if (buildBatchConfigOverride != other.buildBatchConfigOverride) return false
if (buildTimeoutInMinutesOverride != other.buildTimeoutInMinutesOverride) return false
if (buildspecOverride != other.buildspecOverride) return false
if (cacheOverride != other.cacheOverride) return false
if (certificateOverride != other.certificateOverride) return false
if (computeTypeOverride != other.computeTypeOverride) return false
if (debugSessionEnabled != other.debugSessionEnabled) return false
if (encryptionKeyOverride != other.encryptionKeyOverride) return false
if (environmentTypeOverride != other.environmentTypeOverride) return false
if (environmentVariablesOverride != other.environmentVariablesOverride) return false
if (gitCloneDepthOverride != other.gitCloneDepthOverride) return false
if (gitSubmodulesConfigOverride != other.gitSubmodulesConfigOverride) return false
if (idempotencyToken != other.idempotencyToken) return false
if (imageOverride != other.imageOverride) return false
if (imagePullCredentialsTypeOverride != other.imagePullCredentialsTypeOverride) return false
if (insecureSslOverride != other.insecureSslOverride) return false
if (logsConfigOverride != other.logsConfigOverride) return false
if (privilegedModeOverride != other.privilegedModeOverride) return false
if (projectName != other.projectName) return false
if (queuedTimeoutInMinutesOverride != other.queuedTimeoutInMinutesOverride) return false
if (registryCredentialOverride != other.registryCredentialOverride) return false
if (reportBuildBatchStatusOverride != other.reportBuildBatchStatusOverride) return false
if (secondaryArtifactsOverride != other.secondaryArtifactsOverride) return false
if (secondarySourcesOverride != other.secondarySourcesOverride) return false
if (secondarySourcesVersionOverride != other.secondarySourcesVersionOverride) return false
if (serviceRoleOverride != other.serviceRoleOverride) return false
if (sourceAuthOverride != other.sourceAuthOverride) return false
if (sourceLocationOverride != other.sourceLocationOverride) return false
if (sourceTypeOverride != other.sourceTypeOverride) return false
if (sourceVersion != other.sourceVersion) return false
return true
}
inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.codebuild.model.StartBuildBatchRequest = Builder(this).apply(block).build()
class Builder {
/**
* An array of ProjectArtifacts objects that contains information about the
* build output artifact overrides for the build project.
*/
var artifactsOverride: aws.sdk.kotlin.services.codebuild.model.ProjectArtifacts? = null
/**
* A BuildBatchConfigOverride object that contains batch build configuration
* overrides.
*/
var buildBatchConfigOverride: aws.sdk.kotlin.services.codebuild.model.ProjectBuildBatchConfig? = null
/**
* Overrides the build timeout specified in the batch build project.
*/
var buildTimeoutInMinutesOverride: kotlin.Int? = null
/**
* A buildspec file declaration that overrides, for this build only, the latest one
* already defined in the build project.
* If this value is set, it can be either an inline buildspec definition, the path to an
* alternate buildspec file relative to the value of the built-in
* CODEBUILD_SRC_DIR environment variable, or the path to an S3 bucket.
* The bucket must be in the same Amazon Web Services Region as the build project. Specify the buildspec
* file using its ARN (for example,
* arn:aws:s3:::my-codebuild-sample2/buildspec.yml). If this value is not
* provided or is set to an empty string, the source code must contain a buildspec file in
* its root directory. For more information, see Buildspec File Name and Storage Location.
*/
var buildspecOverride: kotlin.String? = null
/**
* A ProjectCache object that specifies cache overrides.
*/
var cacheOverride: aws.sdk.kotlin.services.codebuild.model.ProjectCache? = null
/**
* The name of a certificate for this batch build that overrides the one specified in the batch build
* project.
*/
var certificateOverride: kotlin.String? = null
/**
* The name of a compute type for this batch build that overrides the one specified in the
* batch build project.
*/
var computeTypeOverride: aws.sdk.kotlin.services.codebuild.model.ComputeType? = null
/**
* Specifies if session debugging is enabled for this batch build. For more information, see
* Viewing a running build in Session Manager. Batch session debugging is not supported for matrix batch builds.
*/
var debugSessionEnabled: kotlin.Boolean? = null
/**
* The Key Management Service customer master key (CMK) that overrides the one specified in the batch build
* project. The CMK key encrypts the build output artifacts.
* You can use a cross-account KMS key to encrypt the build output artifacts if your
* service role has permission to that key.
* You can specify either the Amazon Resource Name (ARN) of the CMK or, if available, the CMK's alias (using
* the format alias/).
*/
var encryptionKeyOverride: kotlin.String? = null
/**
* A container type for this batch build that overrides the one specified in the batch build
* project.
*/
var environmentTypeOverride: aws.sdk.kotlin.services.codebuild.model.EnvironmentType? = null
/**
* An array of EnvironmentVariable objects that override, or add to, the
* environment variables defined in the batch build project.
*/
var environmentVariablesOverride: List? = null
/**
* The user-defined depth of history, with a minimum value of 0, that overrides, for this
* batch build only, any previous depth of history defined in the batch build project.
*/
var gitCloneDepthOverride: kotlin.Int? = null
/**
* A GitSubmodulesConfig object that overrides the Git submodules configuration
* for this batch build.
*/
var gitSubmodulesConfigOverride: aws.sdk.kotlin.services.codebuild.model.GitSubmodulesConfig? = null
/**
* A unique, case sensitive identifier you provide to ensure the idempotency of the
* StartBuildBatch request. The token is included in the
* StartBuildBatch request and is valid for five minutes. If you repeat
* the StartBuildBatch request with the same token, but change a parameter,
* CodeBuild returns a parameter mismatch error.
*/
var idempotencyToken: kotlin.String? = null
/**
* The name of an image for this batch build that overrides the one specified in the batch
* build project.
*/
var imageOverride: kotlin.String? = null
/**
* The type of credentials CodeBuild uses to pull images in your batch build. There are two valid
* values:
* CODEBUILD
* Specifies that CodeBuild uses its own credentials. This requires that you
* modify your ECR repository policy to trust CodeBuild's service principal.
* SERVICE_ROLE
* Specifies that CodeBuild uses your build project's service role.
* When using a cross-account or private registry image, you must use
* SERVICE_ROLE credentials. When using an CodeBuild curated image,
* you must use CODEBUILD credentials.
*/
var imagePullCredentialsTypeOverride: aws.sdk.kotlin.services.codebuild.model.ImagePullCredentialsType? = null
/**
* Enable this flag to override the insecure SSL setting that is specified in the batch build
* project. The insecure SSL setting determines whether to ignore SSL warnings while
* connecting to the project source code. This override applies only if the build's source
* is GitHub Enterprise.
*/
var insecureSslOverride: kotlin.Boolean? = null
/**
* A LogsConfig object that override the log settings defined in the batch build
* project.
*/
var logsConfigOverride: aws.sdk.kotlin.services.codebuild.model.LogsConfig? = null
/**
* Enable this flag to override privileged mode in the batch build project.
*/
var privilegedModeOverride: kotlin.Boolean? = null
/**
* The name of the project.
*/
var projectName: kotlin.String? = null
/**
* The number of minutes a batch build is allowed to be queued before it times out.
*/
var queuedTimeoutInMinutesOverride: kotlin.Int? = null
/**
* A RegistryCredential object that overrides credentials for access to a
* private registry.
*/
var registryCredentialOverride: aws.sdk.kotlin.services.codebuild.model.RegistryCredential? = null
/**
* Set to true to report to your source provider the status of a batch build's
* start and completion. If you use this option with a source provider other than GitHub,
* GitHub Enterprise, or Bitbucket, an invalidInputException is thrown.
* The status of a build triggered by a webhook is always reported to your source
* provider.
*/
var reportBuildBatchStatusOverride: kotlin.Boolean? = null
/**
* An array of ProjectArtifacts objects that override the secondary artifacts
* defined in the batch build project.
*/
var secondaryArtifactsOverride: List? = null
/**
* An array of ProjectSource objects that override the secondary sources
* defined in the batch build project.
*/
var secondarySourcesOverride: List? = null
/**
* An array of ProjectSourceVersion objects that override the secondary source
* versions in the batch build project.
*/
var secondarySourcesVersionOverride: List? = null
/**
* The name of a service role for this batch build that overrides the one specified in the
* batch build project.
*/
var serviceRoleOverride: kotlin.String? = null
/**
* A SourceAuth object that overrides the one defined in the batch build
* project. This override applies only if the build project's source is BitBucket or
* GitHub.
*/
var sourceAuthOverride: aws.sdk.kotlin.services.codebuild.model.SourceAuth? = null
/**
* A location that overrides, for this batch build, the source location defined in
* the batch build project.
*/
var sourceLocationOverride: kotlin.String? = null
/**
* The source input type that overrides the source input defined in the batch
* build project.
*/
var sourceTypeOverride: aws.sdk.kotlin.services.codebuild.model.SourceType? = null
/**
* The version of the batch build input to be built, for this build only. If not specified,
* the latest version is used. If specified, the contents depends on the source
* provider:
* CodeCommit
* The commit ID, branch, or Git tag to use.
* GitHub
* The commit ID, pull request ID, branch name, or tag name that corresponds
* to the version of the source code you want to build. If a pull request ID is
* specified, it must use the format pr/pull-request-ID (for
* example pr/25). If a branch name is specified, the branch's
* HEAD commit ID is used. If not specified, the default branch's HEAD commit
* ID is used.
* Bitbucket
* The commit ID, branch name, or tag name that corresponds to the version of
* the source code you want to build. If a branch name is specified, the
* branch's HEAD commit ID is used. If not specified, the default branch's HEAD
* commit ID is used.
* Amazon S3
* The version ID of the object that represents the build input ZIP file to
* use.
* If sourceVersion is specified at the project level, then this
* sourceVersion (at the build level) takes precedence.
* For more information, see Source Version Sample
* with CodeBuild in the CodeBuild User Guide.
*/
var sourceVersion: kotlin.String? = null
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.codebuild.model.StartBuildBatchRequest) : this() {
this.artifactsOverride = x.artifactsOverride
this.buildBatchConfigOverride = x.buildBatchConfigOverride
this.buildTimeoutInMinutesOverride = x.buildTimeoutInMinutesOverride
this.buildspecOverride = x.buildspecOverride
this.cacheOverride = x.cacheOverride
this.certificateOverride = x.certificateOverride
this.computeTypeOverride = x.computeTypeOverride
this.debugSessionEnabled = x.debugSessionEnabled
this.encryptionKeyOverride = x.encryptionKeyOverride
this.environmentTypeOverride = x.environmentTypeOverride
this.environmentVariablesOverride = x.environmentVariablesOverride
this.gitCloneDepthOverride = x.gitCloneDepthOverride
this.gitSubmodulesConfigOverride = x.gitSubmodulesConfigOverride
this.idempotencyToken = x.idempotencyToken
this.imageOverride = x.imageOverride
this.imagePullCredentialsTypeOverride = x.imagePullCredentialsTypeOverride
this.insecureSslOverride = x.insecureSslOverride
this.logsConfigOverride = x.logsConfigOverride
this.privilegedModeOverride = x.privilegedModeOverride
this.projectName = x.projectName
this.queuedTimeoutInMinutesOverride = x.queuedTimeoutInMinutesOverride
this.registryCredentialOverride = x.registryCredentialOverride
this.reportBuildBatchStatusOverride = x.reportBuildBatchStatusOverride
this.secondaryArtifactsOverride = x.secondaryArtifactsOverride
this.secondarySourcesOverride = x.secondarySourcesOverride
this.secondarySourcesVersionOverride = x.secondarySourcesVersionOverride
this.serviceRoleOverride = x.serviceRoleOverride
this.sourceAuthOverride = x.sourceAuthOverride
this.sourceLocationOverride = x.sourceLocationOverride
this.sourceTypeOverride = x.sourceTypeOverride
this.sourceVersion = x.sourceVersion
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.codebuild.model.StartBuildBatchRequest = StartBuildBatchRequest(this)
/**
* construct an [aws.sdk.kotlin.services.codebuild.model.ProjectArtifacts] inside the given [block]
*/
fun artifactsOverride(block: aws.sdk.kotlin.services.codebuild.model.ProjectArtifacts.Builder.() -> kotlin.Unit) {
this.artifactsOverride = aws.sdk.kotlin.services.codebuild.model.ProjectArtifacts.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.codebuild.model.ProjectBuildBatchConfig] inside the given [block]
*/
fun buildBatchConfigOverride(block: aws.sdk.kotlin.services.codebuild.model.ProjectBuildBatchConfig.Builder.() -> kotlin.Unit) {
this.buildBatchConfigOverride = aws.sdk.kotlin.services.codebuild.model.ProjectBuildBatchConfig.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.codebuild.model.ProjectCache] inside the given [block]
*/
fun cacheOverride(block: aws.sdk.kotlin.services.codebuild.model.ProjectCache.Builder.() -> kotlin.Unit) {
this.cacheOverride = aws.sdk.kotlin.services.codebuild.model.ProjectCache.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.codebuild.model.GitSubmodulesConfig] inside the given [block]
*/
fun gitSubmodulesConfigOverride(block: aws.sdk.kotlin.services.codebuild.model.GitSubmodulesConfig.Builder.() -> kotlin.Unit) {
this.gitSubmodulesConfigOverride = aws.sdk.kotlin.services.codebuild.model.GitSubmodulesConfig.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.codebuild.model.LogsConfig] inside the given [block]
*/
fun logsConfigOverride(block: aws.sdk.kotlin.services.codebuild.model.LogsConfig.Builder.() -> kotlin.Unit) {
this.logsConfigOverride = aws.sdk.kotlin.services.codebuild.model.LogsConfig.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.codebuild.model.RegistryCredential] inside the given [block]
*/
fun registryCredentialOverride(block: aws.sdk.kotlin.services.codebuild.model.RegistryCredential.Builder.() -> kotlin.Unit) {
this.registryCredentialOverride = aws.sdk.kotlin.services.codebuild.model.RegistryCredential.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.codebuild.model.SourceAuth] inside the given [block]
*/
fun sourceAuthOverride(block: aws.sdk.kotlin.services.codebuild.model.SourceAuth.Builder.() -> kotlin.Unit) {
this.sourceAuthOverride = aws.sdk.kotlin.services.codebuild.model.SourceAuth.invoke(block)
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy