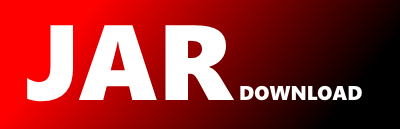
commonMain.aws.sdk.kotlin.services.codegurureviewer.CodeGuruReviewerClient.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of codegurureviewer-jvm Show documentation
Show all versions of codegurureviewer-jvm Show documentation
The AWS SDK for Kotlin client for CodeGuru Reviewer
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.codegurureviewer
import aws.sdk.kotlin.runtime.auth.credentials.DefaultChainCredentialsProvider
import aws.sdk.kotlin.runtime.auth.credentials.internal.manage
import aws.sdk.kotlin.runtime.client.AwsSdkClientConfig
import aws.sdk.kotlin.runtime.config.AbstractAwsSdkClientFactory
import aws.sdk.kotlin.runtime.config.endpoints.resolveEndpointUrl
import aws.sdk.kotlin.runtime.config.profile.AwsProfile
import aws.sdk.kotlin.runtime.config.profile.AwsSharedConfig
import aws.sdk.kotlin.runtime.http.retries.AwsRetryPolicy
import aws.sdk.kotlin.services.codegurureviewer.auth.CodeGuruReviewerAuthSchemeProvider
import aws.sdk.kotlin.services.codegurureviewer.auth.DefaultCodeGuruReviewerAuthSchemeProvider
import aws.sdk.kotlin.services.codegurureviewer.endpoints.CodeGuruReviewerEndpointParameters
import aws.sdk.kotlin.services.codegurureviewer.endpoints.CodeGuruReviewerEndpointProvider
import aws.sdk.kotlin.services.codegurureviewer.endpoints.DefaultCodeGuruReviewerEndpointProvider
import aws.sdk.kotlin.services.codegurureviewer.model.AssociateRepositoryRequest
import aws.sdk.kotlin.services.codegurureviewer.model.AssociateRepositoryResponse
import aws.sdk.kotlin.services.codegurureviewer.model.CreateCodeReviewRequest
import aws.sdk.kotlin.services.codegurureviewer.model.CreateCodeReviewResponse
import aws.sdk.kotlin.services.codegurureviewer.model.DescribeCodeReviewRequest
import aws.sdk.kotlin.services.codegurureviewer.model.DescribeCodeReviewResponse
import aws.sdk.kotlin.services.codegurureviewer.model.DescribeRecommendationFeedbackRequest
import aws.sdk.kotlin.services.codegurureviewer.model.DescribeRecommendationFeedbackResponse
import aws.sdk.kotlin.services.codegurureviewer.model.DescribeRepositoryAssociationRequest
import aws.sdk.kotlin.services.codegurureviewer.model.DescribeRepositoryAssociationResponse
import aws.sdk.kotlin.services.codegurureviewer.model.DisassociateRepositoryRequest
import aws.sdk.kotlin.services.codegurureviewer.model.DisassociateRepositoryResponse
import aws.sdk.kotlin.services.codegurureviewer.model.ListCodeReviewsRequest
import aws.sdk.kotlin.services.codegurureviewer.model.ListCodeReviewsResponse
import aws.sdk.kotlin.services.codegurureviewer.model.ListRecommendationFeedbackRequest
import aws.sdk.kotlin.services.codegurureviewer.model.ListRecommendationFeedbackResponse
import aws.sdk.kotlin.services.codegurureviewer.model.ListRecommendationsRequest
import aws.sdk.kotlin.services.codegurureviewer.model.ListRecommendationsResponse
import aws.sdk.kotlin.services.codegurureviewer.model.ListRepositoryAssociationsRequest
import aws.sdk.kotlin.services.codegurureviewer.model.ListRepositoryAssociationsResponse
import aws.sdk.kotlin.services.codegurureviewer.model.ListTagsForResourceRequest
import aws.sdk.kotlin.services.codegurureviewer.model.ListTagsForResourceResponse
import aws.sdk.kotlin.services.codegurureviewer.model.PutRecommendationFeedbackRequest
import aws.sdk.kotlin.services.codegurureviewer.model.PutRecommendationFeedbackResponse
import aws.sdk.kotlin.services.codegurureviewer.model.TagResourceRequest
import aws.sdk.kotlin.services.codegurureviewer.model.TagResourceResponse
import aws.sdk.kotlin.services.codegurureviewer.model.UntagResourceRequest
import aws.sdk.kotlin.services.codegurureviewer.model.UntagResourceResponse
import aws.smithy.kotlin.runtime.auth.awscredentials.CredentialsProvider
import aws.smithy.kotlin.runtime.auth.awscredentials.CredentialsProviderConfig
import aws.smithy.kotlin.runtime.awsprotocol.ClockSkewInterceptor
import aws.smithy.kotlin.runtime.client.AbstractSdkClientBuilder
import aws.smithy.kotlin.runtime.client.AbstractSdkClientFactory
import aws.smithy.kotlin.runtime.client.IdempotencyTokenConfig
import aws.smithy.kotlin.runtime.client.IdempotencyTokenProvider
import aws.smithy.kotlin.runtime.client.LogMode
import aws.smithy.kotlin.runtime.client.RetryClientConfig
import aws.smithy.kotlin.runtime.client.RetryStrategyClientConfig
import aws.smithy.kotlin.runtime.client.RetryStrategyClientConfigImpl
import aws.smithy.kotlin.runtime.client.SdkClient
import aws.smithy.kotlin.runtime.client.SdkClientConfig
import aws.smithy.kotlin.runtime.http.auth.AuthScheme
import aws.smithy.kotlin.runtime.http.auth.HttpAuthConfig
import aws.smithy.kotlin.runtime.http.config.HttpClientConfig
import aws.smithy.kotlin.runtime.http.config.HttpEngineConfig
import aws.smithy.kotlin.runtime.http.engine.HttpClientEngine
import aws.smithy.kotlin.runtime.http.engine.HttpEngineConfigImpl
import aws.smithy.kotlin.runtime.http.interceptors.HttpInterceptor
import aws.smithy.kotlin.runtime.net.url.Url
import aws.smithy.kotlin.runtime.retries.RetryStrategy
import aws.smithy.kotlin.runtime.retries.policy.RetryPolicy
import aws.smithy.kotlin.runtime.telemetry.Global
import aws.smithy.kotlin.runtime.telemetry.TelemetryConfig
import aws.smithy.kotlin.runtime.telemetry.TelemetryProvider
import aws.smithy.kotlin.runtime.util.LazyAsyncValue
import kotlin.collections.List
import kotlin.jvm.JvmStatic
public const val ServiceId: String = "CodeGuru Reviewer"
public const val SdkVersion: String = "1.3.42"
public const val ServiceApiVersion: String = "2019-09-19"
/**
* This section provides documentation for the Amazon CodeGuru Reviewer API operations. CodeGuru Reviewer is a service that uses program analysis and machine learning to detect potential defects that are difficult for developers to find and recommends fixes in your Java and Python code.
*
* By proactively detecting and providing recommendations for addressing code defects and implementing best practices, CodeGuru Reviewer improves the overall quality and maintainability of your code base during the code review stage. For more information about CodeGuru Reviewer, see the *[Amazon CodeGuru Reviewer User Guide](https://docs.aws.amazon.com/codeguru/latest/reviewer-ug/welcome.html).*
*
* To improve the security of your CodeGuru Reviewer API calls, you can establish a private connection between your VPC and CodeGuru Reviewer by creating an *interface VPC endpoint*. For more information, see [CodeGuru Reviewer and interface VPC endpoints (Amazon Web Services PrivateLink)](https://docs.aws.amazon.com/codeguru/latest/reviewer-ug/vpc-interface-endpoints.html) in the *Amazon CodeGuru Reviewer User Guide*.
*/
public interface CodeGuruReviewerClient : SdkClient {
/**
* CodeGuruReviewerClient's configuration
*/
public override val config: Config
public companion object : AbstractAwsSdkClientFactory()
{
@JvmStatic
override fun builder(): Builder = Builder()
override fun finalizeConfig(builder: Builder) {
super.finalizeConfig(builder)
builder.config.interceptors.add(0, ClockSkewInterceptor())
}
override suspend fun finalizeEnvironmentalConfig(builder: Builder, sharedConfig: LazyAsyncValue, activeProfile: LazyAsyncValue) {
super.finalizeEnvironmentalConfig(builder, sharedConfig, activeProfile)
builder.config.endpointUrl = builder.config.endpointUrl ?: resolveEndpointUrl(
sharedConfig,
"CodeGuruReviewer",
"CODEGURU_REVIEWER",
"codeguru_reviewer",
)
}
}
public class Builder internal constructor(): AbstractSdkClientBuilder() {
override val config: Config.Builder = Config.Builder()
override fun newClient(config: Config): CodeGuruReviewerClient = DefaultCodeGuruReviewerClient(config)
}
public class Config private constructor(builder: Builder) : AwsSdkClientConfig, CredentialsProviderConfig, HttpAuthConfig, HttpClientConfig, HttpEngineConfig by builder.buildHttpEngineConfig(), IdempotencyTokenConfig, RetryClientConfig, RetryStrategyClientConfig by builder.buildRetryStrategyClientConfig(), SdkClientConfig, TelemetryConfig {
override val clientName: String = builder.clientName
override val region: String? = builder.region
override val authSchemes: kotlin.collections.List = builder.authSchemes
override val credentialsProvider: CredentialsProvider = builder.credentialsProvider ?: DefaultChainCredentialsProvider(httpClient = httpClient, region = region).manage()
public val endpointProvider: CodeGuruReviewerEndpointProvider = builder.endpointProvider ?: DefaultCodeGuruReviewerEndpointProvider()
public val endpointUrl: Url? = builder.endpointUrl
override val idempotencyTokenProvider: IdempotencyTokenProvider = builder.idempotencyTokenProvider ?: IdempotencyTokenProvider.Default
override val interceptors: kotlin.collections.List = builder.interceptors
override val logMode: LogMode = builder.logMode ?: LogMode.Default
override val retryPolicy: RetryPolicy = builder.retryPolicy ?: AwsRetryPolicy.Default
override val telemetryProvider: TelemetryProvider = builder.telemetryProvider ?: TelemetryProvider.Global
override val useDualStack: Boolean = builder.useDualStack ?: false
override val useFips: Boolean = builder.useFips ?: false
override val applicationId: String? = builder.applicationId
public val authSchemeProvider: CodeGuruReviewerAuthSchemeProvider = builder.authSchemeProvider ?: DefaultCodeGuruReviewerAuthSchemeProvider()
public companion object {
public inline operator fun invoke(block: Builder.() -> kotlin.Unit): Config = Builder().apply(block).build()
}
public fun toBuilder(): Builder = Builder().apply {
clientName = [email protected]
region = [email protected]
authSchemes = [email protected]
credentialsProvider = [email protected]
endpointProvider = [email protected]
endpointUrl = [email protected]
httpClient = [email protected]
idempotencyTokenProvider = [email protected]
interceptors = [email protected]()
logMode = [email protected]
retryPolicy = [email protected]
retryStrategy = [email protected]
telemetryProvider = [email protected]
useDualStack = [email protected]
useFips = [email protected]
applicationId = [email protected]
authSchemeProvider = [email protected]
}
public class Builder : AwsSdkClientConfig.Builder, CredentialsProviderConfig.Builder, HttpAuthConfig.Builder, HttpClientConfig.Builder, HttpEngineConfig.Builder by HttpEngineConfigImpl.BuilderImpl(), IdempotencyTokenConfig.Builder, RetryClientConfig.Builder, RetryStrategyClientConfig.Builder by RetryStrategyClientConfigImpl.BuilderImpl(), SdkClientConfig.Builder, TelemetryConfig.Builder {
/**
* A reader-friendly name for the client.
*/
override var clientName: String = "CodeGuru Reviewer"
/**
* The AWS region (e.g. `us-west-2`) to make requests to. See about AWS
* [global infrastructure](https://aws.amazon.com/about-aws/global-infrastructure/regions_az/) for more
* information
*/
override var region: String? = null
/**
* Register new or override default [AuthScheme]s configured for this client. By default, the set
* of auth schemes configured comes from the service model. An auth scheme configured explicitly takes
* precedence over the defaults and can be used to customize identity resolution and signing for specific
* authentication schemes.
*/
override var authSchemes: kotlin.collections.List = emptyList()
/**
* The AWS credentials provider to use for authenticating requests. If not provided a
* [aws.sdk.kotlin.runtime.auth.credentials.DefaultChainCredentialsProvider] instance will be used.
* NOTE: The caller is responsible for managing the lifetime of the provider when set. The SDK
* client will not close it when the client is closed.
*/
override var credentialsProvider: CredentialsProvider? = null
/**
* The endpoint provider used to determine where to make service requests. **This is an advanced config
* option.**
*
* Endpoint resolution occurs as part of the workflow for every request made via the service client.
*
* The inputs to endpoint resolution are defined on a per-service basis (see [EndpointParameters]).
*/
public var endpointProvider: CodeGuruReviewerEndpointProvider? = null
/**
* A custom endpoint to route requests to. The endpoint set here is passed to the configured
* [endpointProvider], which may inspect and modify it as needed.
*
* Setting a custom endpointUrl should generally be preferred to overriding the [endpointProvider] and is
* the recommended way to route requests to development or preview instances of a service.
*
* **This is an advanced config option.**
*/
public var endpointUrl: Url? = null
/**
* Override the default idempotency token generator. SDK clients will generate tokens for members
* that represent idempotent tokens when not explicitly set by the caller using this generator.
*/
override var idempotencyTokenProvider: IdempotencyTokenProvider? = null
/**
* Add an [aws.smithy.kotlin.runtime.client.Interceptor] that will have access to read and modify
* the request and response objects as they are processed by the SDK.
* Interceptors added using this method are executed in the order they are configured and are always
* later than any added automatically by the SDK.
*/
override var interceptors: kotlin.collections.MutableList = kotlin.collections.mutableListOf()
/**
* Configure events that will be logged. By default clients will not output
* raw requests or responses. Use this setting to opt-in to additional debug logging.
*
* This can be used to configure logging of requests, responses, retries, etc of SDK clients.
*
* **NOTE**: Logging of raw requests or responses may leak sensitive information! It may also have
* performance considerations when dumping the request/response body. This is primarily a tool for
* debug purposes.
*/
override var logMode: LogMode? = null
/**
* The policy to use for evaluating operation results and determining whether/how to retry.
*/
override var retryPolicy: RetryPolicy? = null
/**
* The telemetry provider used to instrument the SDK operations with. By default, the global telemetry
* provider will be used.
*/
override var telemetryProvider: TelemetryProvider? = null
/**
* Flag to toggle whether to use dual-stack endpoints when making requests.
* See [https://docs.aws.amazon.com/sdkref/latest/guide/feature-endpoints.html] for more information.
* ` Disabled by default.
*/
override var useDualStack: Boolean? = null
/**
* Flag to toggle whether to use [FIPS](https://aws.amazon.com/compliance/fips/) endpoints when making requests.
* ` Disabled by default.
*/
override var useFips: Boolean? = null
/**
* An optional application specific identifier.
* When set it will be appended to the User-Agent header of every request in the form of: `app/{applicationId}`.
* When not explicitly set, the value will be loaded from the following locations:
*
* - JVM System Property: `aws.userAgentAppId`
* - Environment variable: `AWS_SDK_UA_APP_ID`
* - Shared configuration profile attribute: `sdk_ua_app_id`
*
* See [shared configuration settings](https://docs.aws.amazon.com/sdkref/latest/guide/settings-reference.html)
* reference for more information on environment variables and shared config settings.
*/
override var applicationId: String? = null
/**
* Configure the provider used to resolve the authentication scheme to use for a particular operation.
*/
public var authSchemeProvider: CodeGuruReviewerAuthSchemeProvider? = null
override fun build(): Config = Config(this)
}
}
/**
* Use to associate an Amazon Web Services CodeCommit repository or a repository managed by Amazon Web Services CodeStar Connections with Amazon CodeGuru Reviewer. When you associate a repository, CodeGuru Reviewer reviews source code changes in the repository's pull requests and provides automatic recommendations. You can view recommendations using the CodeGuru Reviewer console. For more information, see [Recommendations in Amazon CodeGuru Reviewer](https://docs.aws.amazon.com/codeguru/latest/reviewer-ug/recommendations.html) in the *Amazon CodeGuru Reviewer User Guide.*
*
* If you associate a CodeCommit or S3 repository, it must be in the same Amazon Web Services Region and Amazon Web Services account where its CodeGuru Reviewer code reviews are configured.
*
* Bitbucket and GitHub Enterprise Server repositories are managed by Amazon Web Services CodeStar Connections to connect to CodeGuru Reviewer. For more information, see [Associate a repository](https://docs.aws.amazon.com/codeguru/latest/reviewer-ug/getting-started-associate-repository.html) in the *Amazon CodeGuru Reviewer User Guide.*
*
* You cannot use the CodeGuru Reviewer SDK or the Amazon Web Services CLI to associate a GitHub repository with Amazon CodeGuru Reviewer. To associate a GitHub repository, use the console. For more information, see [Getting started with CodeGuru Reviewer](https://docs.aws.amazon.com/codeguru/latest/reviewer-ug/getting-started-with-guru.html) in the *CodeGuru Reviewer User Guide.*
*/
public suspend fun associateRepository(input: AssociateRepositoryRequest): AssociateRepositoryResponse
/**
* Use to create a code review with a [CodeReviewType](https://docs.aws.amazon.com/codeguru/latest/reviewer-api/API_CodeReviewType.html) of `RepositoryAnalysis`. This type of code review analyzes all code under a specified branch in an associated repository. `PullRequest` code reviews are automatically triggered by a pull request.
*/
public suspend fun createCodeReview(input: CreateCodeReviewRequest): CreateCodeReviewResponse
/**
* Returns the metadata associated with the code review along with its status.
*/
public suspend fun describeCodeReview(input: DescribeCodeReviewRequest): DescribeCodeReviewResponse
/**
* Describes the customer feedback for a CodeGuru Reviewer recommendation.
*/
public suspend fun describeRecommendationFeedback(input: DescribeRecommendationFeedbackRequest): DescribeRecommendationFeedbackResponse
/**
* Returns a [RepositoryAssociation](https://docs.aws.amazon.com/codeguru/latest/reviewer-api/API_RepositoryAssociation.html) object that contains information about the requested repository association.
*/
public suspend fun describeRepositoryAssociation(input: DescribeRepositoryAssociationRequest): DescribeRepositoryAssociationResponse
/**
* Removes the association between Amazon CodeGuru Reviewer and a repository.
*/
public suspend fun disassociateRepository(input: DisassociateRepositoryRequest): DisassociateRepositoryResponse
/**
* Lists all the code reviews that the customer has created in the past 90 days.
*/
public suspend fun listCodeReviews(input: ListCodeReviewsRequest): ListCodeReviewsResponse
/**
* Returns a list of [RecommendationFeedbackSummary](https://docs.aws.amazon.com/codeguru/latest/reviewer-api/API_RecommendationFeedbackSummary.html) objects that contain customer recommendation feedback for all CodeGuru Reviewer users.
*/
public suspend fun listRecommendationFeedback(input: ListRecommendationFeedbackRequest): ListRecommendationFeedbackResponse
/**
* Returns the list of all recommendations for a completed code review.
*/
public suspend fun listRecommendations(input: ListRecommendationsRequest): ListRecommendationsResponse
/**
* Returns a list of [RepositoryAssociationSummary](https://docs.aws.amazon.com/codeguru/latest/reviewer-api/API_RepositoryAssociationSummary.html) objects that contain summary information about a repository association. You can filter the returned list by [ProviderType](https://docs.aws.amazon.com/codeguru/latest/reviewer-api/API_RepositoryAssociationSummary.html#reviewer-Type-RepositoryAssociationSummary-ProviderType), [Name](https://docs.aws.amazon.com/codeguru/latest/reviewer-api/API_RepositoryAssociationSummary.html#reviewer-Type-RepositoryAssociationSummary-Name), [State](https://docs.aws.amazon.com/codeguru/latest/reviewer-api/API_RepositoryAssociationSummary.html#reviewer-Type-RepositoryAssociationSummary-State), and [Owner](https://docs.aws.amazon.com/codeguru/latest/reviewer-api/API_RepositoryAssociationSummary.html#reviewer-Type-RepositoryAssociationSummary-Owner).
*/
public suspend fun listRepositoryAssociations(input: ListRepositoryAssociationsRequest = ListRepositoryAssociationsRequest { }): ListRepositoryAssociationsResponse
/**
* Returns the list of tags associated with an associated repository resource.
*/
public suspend fun listTagsForResource(input: ListTagsForResourceRequest): ListTagsForResourceResponse
/**
* Stores customer feedback for a CodeGuru Reviewer recommendation. When this API is called again with different reactions the previous feedback is overwritten.
*/
public suspend fun putRecommendationFeedback(input: PutRecommendationFeedbackRequest): PutRecommendationFeedbackResponse
/**
* Adds one or more tags to an associated repository.
*/
public suspend fun tagResource(input: TagResourceRequest): TagResourceResponse
/**
* Removes a tag from an associated repository.
*/
public suspend fun untagResource(input: UntagResourceRequest): UntagResourceResponse
}
/**
* Create a copy of the client with one or more configuration values overridden.
* This method allows the caller to perform scoped config overrides for one or more client operations.
*
* Any resources created on your behalf will be shared between clients, and will only be closed when ALL clients using them are closed.
* If you provide a resource (e.g. [HttpClientEngine]) to the SDK, you are responsible for managing the lifetime of that resource.
*/
public fun CodeGuruReviewerClient.withConfig(block: CodeGuruReviewerClient.Config.Builder.() -> Unit): CodeGuruReviewerClient {
val newConfig = config.toBuilder().apply(block).build()
return DefaultCodeGuruReviewerClient(newConfig)
}
/**
* Use to associate an Amazon Web Services CodeCommit repository or a repository managed by Amazon Web Services CodeStar Connections with Amazon CodeGuru Reviewer. When you associate a repository, CodeGuru Reviewer reviews source code changes in the repository's pull requests and provides automatic recommendations. You can view recommendations using the CodeGuru Reviewer console. For more information, see [Recommendations in Amazon CodeGuru Reviewer](https://docs.aws.amazon.com/codeguru/latest/reviewer-ug/recommendations.html) in the *Amazon CodeGuru Reviewer User Guide.*
*
* If you associate a CodeCommit or S3 repository, it must be in the same Amazon Web Services Region and Amazon Web Services account where its CodeGuru Reviewer code reviews are configured.
*
* Bitbucket and GitHub Enterprise Server repositories are managed by Amazon Web Services CodeStar Connections to connect to CodeGuru Reviewer. For more information, see [Associate a repository](https://docs.aws.amazon.com/codeguru/latest/reviewer-ug/getting-started-associate-repository.html) in the *Amazon CodeGuru Reviewer User Guide.*
*
* You cannot use the CodeGuru Reviewer SDK or the Amazon Web Services CLI to associate a GitHub repository with Amazon CodeGuru Reviewer. To associate a GitHub repository, use the console. For more information, see [Getting started with CodeGuru Reviewer](https://docs.aws.amazon.com/codeguru/latest/reviewer-ug/getting-started-with-guru.html) in the *CodeGuru Reviewer User Guide.*
*/
public suspend inline fun CodeGuruReviewerClient.associateRepository(crossinline block: AssociateRepositoryRequest.Builder.() -> Unit): AssociateRepositoryResponse = associateRepository(AssociateRepositoryRequest.Builder().apply(block).build())
/**
* Use to create a code review with a [CodeReviewType](https://docs.aws.amazon.com/codeguru/latest/reviewer-api/API_CodeReviewType.html) of `RepositoryAnalysis`. This type of code review analyzes all code under a specified branch in an associated repository. `PullRequest` code reviews are automatically triggered by a pull request.
*/
public suspend inline fun CodeGuruReviewerClient.createCodeReview(crossinline block: CreateCodeReviewRequest.Builder.() -> Unit): CreateCodeReviewResponse = createCodeReview(CreateCodeReviewRequest.Builder().apply(block).build())
/**
* Returns the metadata associated with the code review along with its status.
*/
public suspend inline fun CodeGuruReviewerClient.describeCodeReview(crossinline block: DescribeCodeReviewRequest.Builder.() -> Unit): DescribeCodeReviewResponse = describeCodeReview(DescribeCodeReviewRequest.Builder().apply(block).build())
/**
* Describes the customer feedback for a CodeGuru Reviewer recommendation.
*/
public suspend inline fun CodeGuruReviewerClient.describeRecommendationFeedback(crossinline block: DescribeRecommendationFeedbackRequest.Builder.() -> Unit): DescribeRecommendationFeedbackResponse = describeRecommendationFeedback(DescribeRecommendationFeedbackRequest.Builder().apply(block).build())
/**
* Returns a [RepositoryAssociation](https://docs.aws.amazon.com/codeguru/latest/reviewer-api/API_RepositoryAssociation.html) object that contains information about the requested repository association.
*/
public suspend inline fun CodeGuruReviewerClient.describeRepositoryAssociation(crossinline block: DescribeRepositoryAssociationRequest.Builder.() -> Unit): DescribeRepositoryAssociationResponse = describeRepositoryAssociation(DescribeRepositoryAssociationRequest.Builder().apply(block).build())
/**
* Removes the association between Amazon CodeGuru Reviewer and a repository.
*/
public suspend inline fun CodeGuruReviewerClient.disassociateRepository(crossinline block: DisassociateRepositoryRequest.Builder.() -> Unit): DisassociateRepositoryResponse = disassociateRepository(DisassociateRepositoryRequest.Builder().apply(block).build())
/**
* Lists all the code reviews that the customer has created in the past 90 days.
*/
public suspend inline fun CodeGuruReviewerClient.listCodeReviews(crossinline block: ListCodeReviewsRequest.Builder.() -> Unit): ListCodeReviewsResponse = listCodeReviews(ListCodeReviewsRequest.Builder().apply(block).build())
/**
* Returns a list of [RecommendationFeedbackSummary](https://docs.aws.amazon.com/codeguru/latest/reviewer-api/API_RecommendationFeedbackSummary.html) objects that contain customer recommendation feedback for all CodeGuru Reviewer users.
*/
public suspend inline fun CodeGuruReviewerClient.listRecommendationFeedback(crossinline block: ListRecommendationFeedbackRequest.Builder.() -> Unit): ListRecommendationFeedbackResponse = listRecommendationFeedback(ListRecommendationFeedbackRequest.Builder().apply(block).build())
/**
* Returns the list of all recommendations for a completed code review.
*/
public suspend inline fun CodeGuruReviewerClient.listRecommendations(crossinline block: ListRecommendationsRequest.Builder.() -> Unit): ListRecommendationsResponse = listRecommendations(ListRecommendationsRequest.Builder().apply(block).build())
/**
* Returns a list of [RepositoryAssociationSummary](https://docs.aws.amazon.com/codeguru/latest/reviewer-api/API_RepositoryAssociationSummary.html) objects that contain summary information about a repository association. You can filter the returned list by [ProviderType](https://docs.aws.amazon.com/codeguru/latest/reviewer-api/API_RepositoryAssociationSummary.html#reviewer-Type-RepositoryAssociationSummary-ProviderType), [Name](https://docs.aws.amazon.com/codeguru/latest/reviewer-api/API_RepositoryAssociationSummary.html#reviewer-Type-RepositoryAssociationSummary-Name), [State](https://docs.aws.amazon.com/codeguru/latest/reviewer-api/API_RepositoryAssociationSummary.html#reviewer-Type-RepositoryAssociationSummary-State), and [Owner](https://docs.aws.amazon.com/codeguru/latest/reviewer-api/API_RepositoryAssociationSummary.html#reviewer-Type-RepositoryAssociationSummary-Owner).
*/
public suspend inline fun CodeGuruReviewerClient.listRepositoryAssociations(crossinline block: ListRepositoryAssociationsRequest.Builder.() -> Unit): ListRepositoryAssociationsResponse = listRepositoryAssociations(ListRepositoryAssociationsRequest.Builder().apply(block).build())
/**
* Returns the list of tags associated with an associated repository resource.
*/
public suspend inline fun CodeGuruReviewerClient.listTagsForResource(crossinline block: ListTagsForResourceRequest.Builder.() -> Unit): ListTagsForResourceResponse = listTagsForResource(ListTagsForResourceRequest.Builder().apply(block).build())
/**
* Stores customer feedback for a CodeGuru Reviewer recommendation. When this API is called again with different reactions the previous feedback is overwritten.
*/
public suspend inline fun CodeGuruReviewerClient.putRecommendationFeedback(crossinline block: PutRecommendationFeedbackRequest.Builder.() -> Unit): PutRecommendationFeedbackResponse = putRecommendationFeedback(PutRecommendationFeedbackRequest.Builder().apply(block).build())
/**
* Adds one or more tags to an associated repository.
*/
public suspend inline fun CodeGuruReviewerClient.tagResource(crossinline block: TagResourceRequest.Builder.() -> Unit): TagResourceResponse = tagResource(TagResourceRequest.Builder().apply(block).build())
/**
* Removes a tag from an associated repository.
*/
public suspend inline fun CodeGuruReviewerClient.untagResource(crossinline block: UntagResourceRequest.Builder.() -> Unit): UntagResourceResponse = untagResource(UntagResourceRequest.Builder().apply(block).build())
© 2015 - 2025 Weber Informatics LLC | Privacy Policy