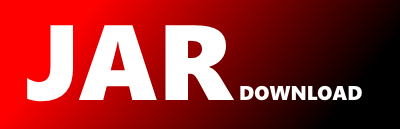
commonMain.aws.sdk.kotlin.services.codepipeline.model.ActionConfigurationProperty.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of codepipeline-jvm Show documentation
Show all versions of codepipeline-jvm Show documentation
The AWS SDK for Kotlin client for CodePipeline
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.codepipeline.model
/**
* Represents information about an action configuration property.
*/
public class ActionConfigurationProperty private constructor(builder: Builder) {
/**
* The description of the action configuration property that is displayed to users.
*/
public val description: kotlin.String? = builder.description
/**
* Whether the configuration property is a key.
*/
public val key: kotlin.Boolean = builder.key
/**
* The name of the action configuration property.
*/
public val name: kotlin.String = requireNotNull(builder.name) { "A non-null value must be provided for name" }
/**
* Indicates that the property is used with `PollForJobs`. When creating a custom action, an action can have up to one queryable property. If it has one, that property must be both required and not secret.
*
* If you create a pipeline with a custom action type, and that custom action contains a queryable property, the value for that configuration property is subject to other restrictions. The value must be less than or equal to twenty (20) characters. The value can contain only alphanumeric characters, underscores, and hyphens.
*/
public val queryable: kotlin.Boolean = builder.queryable
/**
* Whether the configuration property is a required value.
*/
public val required: kotlin.Boolean = builder.required
/**
* Whether the configuration property is secret. Secrets are hidden from all calls except for `GetJobDetails`, `GetThirdPartyJobDetails`, `PollForJobs`, and `PollForThirdPartyJobs`.
*
* When updating a pipeline, passing * * * * * without changing any other values of the action preserves the previous value of the secret.
*/
public val secret: kotlin.Boolean = builder.secret
/**
* The type of the configuration property.
*/
public val type: aws.sdk.kotlin.services.codepipeline.model.ActionConfigurationPropertyType? = builder.type
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.codepipeline.model.ActionConfigurationProperty = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("ActionConfigurationProperty(")
append("description=$description,")
append("key=$key,")
append("name=$name,")
append("queryable=$queryable,")
append("required=$required,")
append("secret=$secret,")
append("type=$type")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = description?.hashCode() ?: 0
result = 31 * result + (key.hashCode())
result = 31 * result + (name.hashCode())
result = 31 * result + (queryable.hashCode())
result = 31 * result + (required.hashCode())
result = 31 * result + (secret.hashCode())
result = 31 * result + (type?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as ActionConfigurationProperty
if (description != other.description) return false
if (key != other.key) return false
if (name != other.name) return false
if (queryable != other.queryable) return false
if (required != other.required) return false
if (secret != other.secret) return false
if (type != other.type) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.codepipeline.model.ActionConfigurationProperty = Builder(this).apply(block).build()
public class Builder {
/**
* The description of the action configuration property that is displayed to users.
*/
public var description: kotlin.String? = null
/**
* Whether the configuration property is a key.
*/
public var key: kotlin.Boolean = false
/**
* The name of the action configuration property.
*/
public var name: kotlin.String? = null
/**
* Indicates that the property is used with `PollForJobs`. When creating a custom action, an action can have up to one queryable property. If it has one, that property must be both required and not secret.
*
* If you create a pipeline with a custom action type, and that custom action contains a queryable property, the value for that configuration property is subject to other restrictions. The value must be less than or equal to twenty (20) characters. The value can contain only alphanumeric characters, underscores, and hyphens.
*/
public var queryable: kotlin.Boolean = false
/**
* Whether the configuration property is a required value.
*/
public var required: kotlin.Boolean = false
/**
* Whether the configuration property is secret. Secrets are hidden from all calls except for `GetJobDetails`, `GetThirdPartyJobDetails`, `PollForJobs`, and `PollForThirdPartyJobs`.
*
* When updating a pipeline, passing * * * * * without changing any other values of the action preserves the previous value of the secret.
*/
public var secret: kotlin.Boolean = false
/**
* The type of the configuration property.
*/
public var type: aws.sdk.kotlin.services.codepipeline.model.ActionConfigurationPropertyType? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.codepipeline.model.ActionConfigurationProperty) : this() {
this.description = x.description
this.key = x.key
this.name = x.name
this.queryable = x.queryable
this.required = x.required
this.secret = x.secret
this.type = x.type
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.codepipeline.model.ActionConfigurationProperty = ActionConfigurationProperty(this)
internal fun correctErrors(): Builder {
if (name == null) name = ""
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy