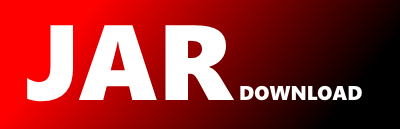
commonMain.aws.sdk.kotlin.services.codepipeline.model.PipelineDeclaration.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of codepipeline-jvm Show documentation
Show all versions of codepipeline-jvm Show documentation
The AWS SDK for Kotlin client for CodePipeline
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.codepipeline.model
/**
* Represents the structure of actions and stages to be performed in the pipeline.
*/
public class PipelineDeclaration private constructor(builder: Builder) {
/**
* Represents information about the S3 bucket where artifacts are stored for the pipeline.
*
* You must include either `artifactStore` or `artifactStores` in your pipeline, but you cannot use both. If you create a cross-region action in your pipeline, you must use `artifactStores`.
*/
public val artifactStore: aws.sdk.kotlin.services.codepipeline.model.ArtifactStore? = builder.artifactStore
/**
* A mapping of `artifactStore` objects and their corresponding Amazon Web Services Regions. There must be an artifact store for the pipeline Region and for each cross-region action in the pipeline.
*
* You must include either `artifactStore` or `artifactStores` in your pipeline, but you cannot use both. If you create a cross-region action in your pipeline, you must use `artifactStores`.
*/
public val artifactStores: Map? = builder.artifactStores
/**
* The name of the pipeline.
*/
public val name: kotlin.String = requireNotNull(builder.name) { "A non-null value must be provided for name" }
/**
* CodePipeline provides the following pipeline types, which differ in characteristics and price, so that you can tailor your pipeline features and cost to the needs of your applications.
* + V1 type pipelines have a JSON structure that contains standard pipeline, stage, and action-level parameters.
* + V2 type pipelines have the same structure as a V1 type, along with additional parameters for release safety and trigger configuration.
*
* Including V2 parameters, such as triggers on Git tags, in the pipeline JSON when creating or updating a pipeline will result in the pipeline having the V2 type of pipeline and the associated costs.
*
* For information about pricing for CodePipeline, see [Pricing](https://aws.amazon.com/codepipeline/pricing/).
*
* For information about which type of pipeline to choose, see [What type of pipeline is right for me?](https://docs.aws.amazon.com/codepipeline/latest/userguide/pipeline-types-planning.html).
*
* V2 type pipelines, along with triggers on Git tags and pipeline-level variables, are not currently supported for CloudFormation and CDK resources in CodePipeline. For more information about V2 type pipelines, see [Pipeline types](https://docs.aws.amazon.com/codepipeline/latest/userguide/pipeline-types.html) in the *CodePipeline User Guide*.
*/
public val pipelineType: aws.sdk.kotlin.services.codepipeline.model.PipelineType? = builder.pipelineType
/**
* The Amazon Resource Name (ARN) for CodePipeline to use to either perform actions with no `actionRoleArn`, or to use to assume roles for actions with an `actionRoleArn`.
*/
public val roleArn: kotlin.String = requireNotNull(builder.roleArn) { "A non-null value must be provided for roleArn" }
/**
* The stage in which to perform the action.
*/
public val stages: List = requireNotNull(builder.stages) { "A non-null value must be provided for stages" }
/**
* The trigger configuration specifying a type of event, such as Git tags, that starts the pipeline.
*
* When a trigger configuration is specified, default change detection for repository and branch commits is disabled.
*/
public val triggers: List? = builder.triggers
/**
* A list that defines the pipeline variables for a pipeline resource. Variable names can have alphanumeric and underscore characters, and the values must match `[A-Za-z0-9@\-_]+`.
*/
public val variables: List? = builder.variables
/**
* The version number of the pipeline. A new pipeline always has a version number of 1. This number is incremented when a pipeline is updated.
*/
public val version: kotlin.Int? = builder.version
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.codepipeline.model.PipelineDeclaration = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("PipelineDeclaration(")
append("artifactStore=$artifactStore,")
append("artifactStores=$artifactStores,")
append("name=$name,")
append("pipelineType=$pipelineType,")
append("roleArn=$roleArn,")
append("stages=$stages,")
append("triggers=$triggers,")
append("variables=$variables,")
append("version=$version")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = artifactStore?.hashCode() ?: 0
result = 31 * result + (artifactStores?.hashCode() ?: 0)
result = 31 * result + (name.hashCode())
result = 31 * result + (pipelineType?.hashCode() ?: 0)
result = 31 * result + (roleArn.hashCode())
result = 31 * result + (stages.hashCode())
result = 31 * result + (triggers?.hashCode() ?: 0)
result = 31 * result + (variables?.hashCode() ?: 0)
result = 31 * result + (version ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as PipelineDeclaration
if (artifactStore != other.artifactStore) return false
if (artifactStores != other.artifactStores) return false
if (name != other.name) return false
if (pipelineType != other.pipelineType) return false
if (roleArn != other.roleArn) return false
if (stages != other.stages) return false
if (triggers != other.triggers) return false
if (variables != other.variables) return false
if (version != other.version) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.codepipeline.model.PipelineDeclaration = Builder(this).apply(block).build()
public class Builder {
/**
* Represents information about the S3 bucket where artifacts are stored for the pipeline.
*
* You must include either `artifactStore` or `artifactStores` in your pipeline, but you cannot use both. If you create a cross-region action in your pipeline, you must use `artifactStores`.
*/
public var artifactStore: aws.sdk.kotlin.services.codepipeline.model.ArtifactStore? = null
/**
* A mapping of `artifactStore` objects and their corresponding Amazon Web Services Regions. There must be an artifact store for the pipeline Region and for each cross-region action in the pipeline.
*
* You must include either `artifactStore` or `artifactStores` in your pipeline, but you cannot use both. If you create a cross-region action in your pipeline, you must use `artifactStores`.
*/
public var artifactStores: Map? = null
/**
* The name of the pipeline.
*/
public var name: kotlin.String? = null
/**
* CodePipeline provides the following pipeline types, which differ in characteristics and price, so that you can tailor your pipeline features and cost to the needs of your applications.
* + V1 type pipelines have a JSON structure that contains standard pipeline, stage, and action-level parameters.
* + V2 type pipelines have the same structure as a V1 type, along with additional parameters for release safety and trigger configuration.
*
* Including V2 parameters, such as triggers on Git tags, in the pipeline JSON when creating or updating a pipeline will result in the pipeline having the V2 type of pipeline and the associated costs.
*
* For information about pricing for CodePipeline, see [Pricing](https://aws.amazon.com/codepipeline/pricing/).
*
* For information about which type of pipeline to choose, see [What type of pipeline is right for me?](https://docs.aws.amazon.com/codepipeline/latest/userguide/pipeline-types-planning.html).
*
* V2 type pipelines, along with triggers on Git tags and pipeline-level variables, are not currently supported for CloudFormation and CDK resources in CodePipeline. For more information about V2 type pipelines, see [Pipeline types](https://docs.aws.amazon.com/codepipeline/latest/userguide/pipeline-types.html) in the *CodePipeline User Guide*.
*/
public var pipelineType: aws.sdk.kotlin.services.codepipeline.model.PipelineType? = null
/**
* The Amazon Resource Name (ARN) for CodePipeline to use to either perform actions with no `actionRoleArn`, or to use to assume roles for actions with an `actionRoleArn`.
*/
public var roleArn: kotlin.String? = null
/**
* The stage in which to perform the action.
*/
public var stages: List? = null
/**
* The trigger configuration specifying a type of event, such as Git tags, that starts the pipeline.
*
* When a trigger configuration is specified, default change detection for repository and branch commits is disabled.
*/
public var triggers: List? = null
/**
* A list that defines the pipeline variables for a pipeline resource. Variable names can have alphanumeric and underscore characters, and the values must match `[A-Za-z0-9@\-_]+`.
*/
public var variables: List? = null
/**
* The version number of the pipeline. A new pipeline always has a version number of 1. This number is incremented when a pipeline is updated.
*/
public var version: kotlin.Int? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.codepipeline.model.PipelineDeclaration) : this() {
this.artifactStore = x.artifactStore
this.artifactStores = x.artifactStores
this.name = x.name
this.pipelineType = x.pipelineType
this.roleArn = x.roleArn
this.stages = x.stages
this.triggers = x.triggers
this.variables = x.variables
this.version = x.version
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.codepipeline.model.PipelineDeclaration = PipelineDeclaration(this)
/**
* construct an [aws.sdk.kotlin.services.codepipeline.model.ArtifactStore] inside the given [block]
*/
public fun artifactStore(block: aws.sdk.kotlin.services.codepipeline.model.ArtifactStore.Builder.() -> kotlin.Unit) {
this.artifactStore = aws.sdk.kotlin.services.codepipeline.model.ArtifactStore.invoke(block)
}
internal fun correctErrors(): Builder {
if (name == null) name = ""
if (roleArn == null) roleArn = ""
if (stages == null) stages = emptyList()
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy