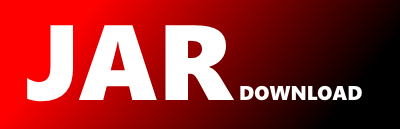
commonMain.aws.sdk.kotlin.services.codepipeline.model.PipelineSummary.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of codepipeline-jvm Show documentation
Show all versions of codepipeline-jvm Show documentation
The AWS SDK for Kotlin client for CodePipeline
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.codepipeline.model
import aws.smithy.kotlin.runtime.time.Instant
/**
* Returns a summary of a pipeline.
*/
public class PipelineSummary private constructor(builder: Builder) {
/**
* The date and time the pipeline was created, in timestamp format.
*/
public val created: aws.smithy.kotlin.runtime.time.Instant? = builder.created
/**
* The name of the pipeline.
*/
public val name: kotlin.String? = builder.name
/**
* CodePipeline provides the following pipeline types, which differ in characteristics and price, so that you can tailor your pipeline features and cost to the needs of your applications.
* + V1 type pipelines have a JSON structure that contains standard pipeline, stage, and action-level parameters.
* + V2 type pipelines have the same structure as a V1 type, along with additional parameters for release safety and trigger configuration.
*
* Including V2 parameters, such as triggers on Git tags, in the pipeline JSON when creating or updating a pipeline will result in the pipeline having the V2 type of pipeline and the associated costs.
*
* For information about pricing for CodePipeline, see [Pricing](https://aws.amazon.com/codepipeline/pricing/).
*
* For information about which type of pipeline to choose, see [What type of pipeline is right for me?](https://docs.aws.amazon.com/codepipeline/latest/userguide/pipeline-types-planning.html).
*
* V2 type pipelines, along with triggers on Git tags and pipeline-level variables, are not currently supported for CloudFormation and CDK resources in CodePipeline. For more information about V2 type pipelines, see [Pipeline types](https://docs.aws.amazon.com/codepipeline/latest/userguide/pipeline-types.html) in the *CodePipeline User Guide*.
*/
public val pipelineType: aws.sdk.kotlin.services.codepipeline.model.PipelineType? = builder.pipelineType
/**
* The date and time of the last update to the pipeline, in timestamp format.
*/
public val updated: aws.smithy.kotlin.runtime.time.Instant? = builder.updated
/**
* The version number of the pipeline.
*/
public val version: kotlin.Int? = builder.version
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.codepipeline.model.PipelineSummary = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("PipelineSummary(")
append("created=$created,")
append("name=$name,")
append("pipelineType=$pipelineType,")
append("updated=$updated,")
append("version=$version")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = created?.hashCode() ?: 0
result = 31 * result + (name?.hashCode() ?: 0)
result = 31 * result + (pipelineType?.hashCode() ?: 0)
result = 31 * result + (updated?.hashCode() ?: 0)
result = 31 * result + (version ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as PipelineSummary
if (created != other.created) return false
if (name != other.name) return false
if (pipelineType != other.pipelineType) return false
if (updated != other.updated) return false
if (version != other.version) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.codepipeline.model.PipelineSummary = Builder(this).apply(block).build()
public class Builder {
/**
* The date and time the pipeline was created, in timestamp format.
*/
public var created: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The name of the pipeline.
*/
public var name: kotlin.String? = null
/**
* CodePipeline provides the following pipeline types, which differ in characteristics and price, so that you can tailor your pipeline features and cost to the needs of your applications.
* + V1 type pipelines have a JSON structure that contains standard pipeline, stage, and action-level parameters.
* + V2 type pipelines have the same structure as a V1 type, along with additional parameters for release safety and trigger configuration.
*
* Including V2 parameters, such as triggers on Git tags, in the pipeline JSON when creating or updating a pipeline will result in the pipeline having the V2 type of pipeline and the associated costs.
*
* For information about pricing for CodePipeline, see [Pricing](https://aws.amazon.com/codepipeline/pricing/).
*
* For information about which type of pipeline to choose, see [What type of pipeline is right for me?](https://docs.aws.amazon.com/codepipeline/latest/userguide/pipeline-types-planning.html).
*
* V2 type pipelines, along with triggers on Git tags and pipeline-level variables, are not currently supported for CloudFormation and CDK resources in CodePipeline. For more information about V2 type pipelines, see [Pipeline types](https://docs.aws.amazon.com/codepipeline/latest/userguide/pipeline-types.html) in the *CodePipeline User Guide*.
*/
public var pipelineType: aws.sdk.kotlin.services.codepipeline.model.PipelineType? = null
/**
* The date and time of the last update to the pipeline, in timestamp format.
*/
public var updated: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The version number of the pipeline.
*/
public var version: kotlin.Int? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.codepipeline.model.PipelineSummary) : this() {
this.created = x.created
this.name = x.name
this.pipelineType = x.pipelineType
this.updated = x.updated
this.version = x.version
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.codepipeline.model.PipelineSummary = PipelineSummary(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy