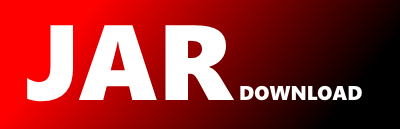
commonMain.aws.sdk.kotlin.services.codepipeline.model.SourceRevisionOverride.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of codepipeline-jvm Show documentation
Show all versions of codepipeline-jvm Show documentation
The AWS SDK for Kotlin client for CodePipeline
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.codepipeline.model
/**
* A list that allows you to specify, or override, the source revision for a pipeline execution that's being started. A source revision is the version with all the changes to your application code, or source artifact, for the pipeline execution.
*/
public class SourceRevisionOverride private constructor(builder: Builder) {
/**
* The name of the action where the override will be applied.
*/
public val actionName: kotlin.String = requireNotNull(builder.actionName) { "A non-null value must be provided for actionName" }
/**
* The type of source revision, based on the source provider. For example, the revision type for the CodeCommit action provider is the commit ID.
*/
public val revisionType: aws.sdk.kotlin.services.codepipeline.model.SourceRevisionType = requireNotNull(builder.revisionType) { "A non-null value must be provided for revisionType" }
/**
* The source revision, or version of your source artifact, with the changes that you want to run in the pipeline execution.
*/
public val revisionValue: kotlin.String = requireNotNull(builder.revisionValue) { "A non-null value must be provided for revisionValue" }
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.codepipeline.model.SourceRevisionOverride = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("SourceRevisionOverride(")
append("actionName=$actionName,")
append("revisionType=$revisionType,")
append("revisionValue=$revisionValue")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = actionName.hashCode()
result = 31 * result + (revisionType.hashCode())
result = 31 * result + (revisionValue.hashCode())
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as SourceRevisionOverride
if (actionName != other.actionName) return false
if (revisionType != other.revisionType) return false
if (revisionValue != other.revisionValue) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.codepipeline.model.SourceRevisionOverride = Builder(this).apply(block).build()
public class Builder {
/**
* The name of the action where the override will be applied.
*/
public var actionName: kotlin.String? = null
/**
* The type of source revision, based on the source provider. For example, the revision type for the CodeCommit action provider is the commit ID.
*/
public var revisionType: aws.sdk.kotlin.services.codepipeline.model.SourceRevisionType? = null
/**
* The source revision, or version of your source artifact, with the changes that you want to run in the pipeline execution.
*/
public var revisionValue: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.codepipeline.model.SourceRevisionOverride) : this() {
this.actionName = x.actionName
this.revisionType = x.revisionType
this.revisionValue = x.revisionValue
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.codepipeline.model.SourceRevisionOverride = SourceRevisionOverride(this)
internal fun correctErrors(): Builder {
if (actionName == null) actionName = ""
if (revisionType == null) revisionType = SourceRevisionType.SdkUnknown("no value provided")
if (revisionValue == null) revisionValue = ""
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy