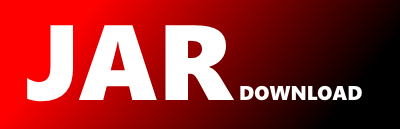
commonMain.aws.sdk.kotlin.services.codepipeline.model.ThirdPartyJobData.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of codepipeline-jvm Show documentation
Show all versions of codepipeline-jvm Show documentation
The AWS SDK for Kotlin client for CodePipeline
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.codepipeline.model
/**
* Represents information about the job data for a partner action.
*/
public class ThirdPartyJobData private constructor(builder: Builder) {
/**
* Represents information about an action configuration.
*/
public val actionConfiguration: aws.sdk.kotlin.services.codepipeline.model.ActionConfiguration? = builder.actionConfiguration
/**
* Represents information about an action type.
*/
public val actionTypeId: aws.sdk.kotlin.services.codepipeline.model.ActionTypeId? = builder.actionTypeId
/**
* Represents an Amazon Web Services session credentials object. These credentials are temporary credentials that are issued by Amazon Web Services Secure Token Service (STS). They can be used to access input and output artifacts in the S3 bucket used to store artifact for the pipeline in CodePipeline.
*/
public val artifactCredentials: aws.sdk.kotlin.services.codepipeline.model.AwsSessionCredentials? = builder.artifactCredentials
/**
* A system-generated token, such as a CodeDeploy deployment ID, that a job requires to continue the job asynchronously.
*/
public val continuationToken: kotlin.String? = builder.continuationToken
/**
* The encryption key used to encrypt and decrypt data in the artifact store for the pipeline, such as an Amazon Web Services Key Management Service (Amazon Web Services KMS) key. This is optional and might not be present.
*/
public val encryptionKey: aws.sdk.kotlin.services.codepipeline.model.EncryptionKey? = builder.encryptionKey
/**
* The name of the artifact that is worked on by the action, if any. This name might be system-generated, such as "MyApp", or it might be defined by the user when the action is created. The input artifact name must match the name of an output artifact generated by an action in an earlier action or stage of the pipeline.
*/
public val inputArtifacts: List? = builder.inputArtifacts
/**
* The name of the artifact that is the result of the action, if any. This name might be system-generated, such as "MyBuiltApp", or it might be defined by the user when the action is created.
*/
public val outputArtifacts: List? = builder.outputArtifacts
/**
* Represents information about a pipeline to a job worker.
*
* Does not include `pipelineArn` and `pipelineExecutionId` for ThirdParty jobs.
*/
public val pipelineContext: aws.sdk.kotlin.services.codepipeline.model.PipelineContext? = builder.pipelineContext
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.codepipeline.model.ThirdPartyJobData = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("ThirdPartyJobData(")
append("actionConfiguration=$actionConfiguration,")
append("actionTypeId=$actionTypeId,")
append("artifactCredentials=*** Sensitive Data Redacted ***,")
append("continuationToken=$continuationToken,")
append("encryptionKey=$encryptionKey,")
append("inputArtifacts=$inputArtifacts,")
append("outputArtifacts=$outputArtifacts,")
append("pipelineContext=$pipelineContext")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = actionConfiguration?.hashCode() ?: 0
result = 31 * result + (actionTypeId?.hashCode() ?: 0)
result = 31 * result + (artifactCredentials?.hashCode() ?: 0)
result = 31 * result + (continuationToken?.hashCode() ?: 0)
result = 31 * result + (encryptionKey?.hashCode() ?: 0)
result = 31 * result + (inputArtifacts?.hashCode() ?: 0)
result = 31 * result + (outputArtifacts?.hashCode() ?: 0)
result = 31 * result + (pipelineContext?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as ThirdPartyJobData
if (actionConfiguration != other.actionConfiguration) return false
if (actionTypeId != other.actionTypeId) return false
if (artifactCredentials != other.artifactCredentials) return false
if (continuationToken != other.continuationToken) return false
if (encryptionKey != other.encryptionKey) return false
if (inputArtifacts != other.inputArtifacts) return false
if (outputArtifacts != other.outputArtifacts) return false
if (pipelineContext != other.pipelineContext) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.codepipeline.model.ThirdPartyJobData = Builder(this).apply(block).build()
public class Builder {
/**
* Represents information about an action configuration.
*/
public var actionConfiguration: aws.sdk.kotlin.services.codepipeline.model.ActionConfiguration? = null
/**
* Represents information about an action type.
*/
public var actionTypeId: aws.sdk.kotlin.services.codepipeline.model.ActionTypeId? = null
/**
* Represents an Amazon Web Services session credentials object. These credentials are temporary credentials that are issued by Amazon Web Services Secure Token Service (STS). They can be used to access input and output artifacts in the S3 bucket used to store artifact for the pipeline in CodePipeline.
*/
public var artifactCredentials: aws.sdk.kotlin.services.codepipeline.model.AwsSessionCredentials? = null
/**
* A system-generated token, such as a CodeDeploy deployment ID, that a job requires to continue the job asynchronously.
*/
public var continuationToken: kotlin.String? = null
/**
* The encryption key used to encrypt and decrypt data in the artifact store for the pipeline, such as an Amazon Web Services Key Management Service (Amazon Web Services KMS) key. This is optional and might not be present.
*/
public var encryptionKey: aws.sdk.kotlin.services.codepipeline.model.EncryptionKey? = null
/**
* The name of the artifact that is worked on by the action, if any. This name might be system-generated, such as "MyApp", or it might be defined by the user when the action is created. The input artifact name must match the name of an output artifact generated by an action in an earlier action or stage of the pipeline.
*/
public var inputArtifacts: List? = null
/**
* The name of the artifact that is the result of the action, if any. This name might be system-generated, such as "MyBuiltApp", or it might be defined by the user when the action is created.
*/
public var outputArtifacts: List? = null
/**
* Represents information about a pipeline to a job worker.
*
* Does not include `pipelineArn` and `pipelineExecutionId` for ThirdParty jobs.
*/
public var pipelineContext: aws.sdk.kotlin.services.codepipeline.model.PipelineContext? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.codepipeline.model.ThirdPartyJobData) : this() {
this.actionConfiguration = x.actionConfiguration
this.actionTypeId = x.actionTypeId
this.artifactCredentials = x.artifactCredentials
this.continuationToken = x.continuationToken
this.encryptionKey = x.encryptionKey
this.inputArtifacts = x.inputArtifacts
this.outputArtifacts = x.outputArtifacts
this.pipelineContext = x.pipelineContext
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.codepipeline.model.ThirdPartyJobData = ThirdPartyJobData(this)
/**
* construct an [aws.sdk.kotlin.services.codepipeline.model.ActionConfiguration] inside the given [block]
*/
public fun actionConfiguration(block: aws.sdk.kotlin.services.codepipeline.model.ActionConfiguration.Builder.() -> kotlin.Unit) {
this.actionConfiguration = aws.sdk.kotlin.services.codepipeline.model.ActionConfiguration.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.codepipeline.model.ActionTypeId] inside the given [block]
*/
public fun actionTypeId(block: aws.sdk.kotlin.services.codepipeline.model.ActionTypeId.Builder.() -> kotlin.Unit) {
this.actionTypeId = aws.sdk.kotlin.services.codepipeline.model.ActionTypeId.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.codepipeline.model.AwsSessionCredentials] inside the given [block]
*/
public fun artifactCredentials(block: aws.sdk.kotlin.services.codepipeline.model.AwsSessionCredentials.Builder.() -> kotlin.Unit) {
this.artifactCredentials = aws.sdk.kotlin.services.codepipeline.model.AwsSessionCredentials.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.codepipeline.model.EncryptionKey] inside the given [block]
*/
public fun encryptionKey(block: aws.sdk.kotlin.services.codepipeline.model.EncryptionKey.Builder.() -> kotlin.Unit) {
this.encryptionKey = aws.sdk.kotlin.services.codepipeline.model.EncryptionKey.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.codepipeline.model.PipelineContext] inside the given [block]
*/
public fun pipelineContext(block: aws.sdk.kotlin.services.codepipeline.model.PipelineContext.Builder.() -> kotlin.Unit) {
this.pipelineContext = aws.sdk.kotlin.services.codepipeline.model.PipelineContext.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy