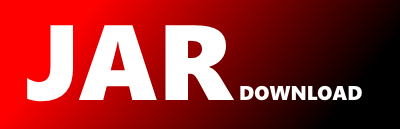
commonMain.aws.sdk.kotlin.services.codepipeline.model.WebhookDefinition.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of codepipeline-jvm Show documentation
Show all versions of codepipeline-jvm Show documentation
The AWS SDK for Kotlin client for CodePipeline
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.codepipeline.model
/**
* Represents information about a webhook and its definition.
*/
public class WebhookDefinition private constructor(builder: Builder) {
/**
* Supported options are GITHUB_HMAC, IP, and UNAUTHENTICATED.
* + For information about the authentication scheme implemented by GITHUB_HMAC, see [Securing your webhooks](https://developer.github.com/webhooks/securing/) on the GitHub Developer website.
* + IP rejects webhooks trigger requests unless they originate from an IP address in the IP range whitelisted in the authentication configuration.
* + UNAUTHENTICATED accepts all webhook trigger requests regardless of origin.
*/
public val authentication: aws.sdk.kotlin.services.codepipeline.model.WebhookAuthenticationType = requireNotNull(builder.authentication) { "A non-null value must be provided for authentication" }
/**
* Properties that configure the authentication applied to incoming webhook trigger requests. The required properties depend on the authentication type. For GITHUB_HMAC, only the `SecretToken `property must be set. For IP, only the `AllowedIPRange `property must be set to a valid CIDR range. For UNAUTHENTICATED, no properties can be set.
*/
public val authenticationConfiguration: aws.sdk.kotlin.services.codepipeline.model.WebhookAuthConfiguration? = builder.authenticationConfiguration
/**
* A list of rules applied to the body/payload sent in the POST request to a webhook URL. All defined rules must pass for the request to be accepted and the pipeline started.
*/
public val filters: List = requireNotNull(builder.filters) { "A non-null value must be provided for filters" }
/**
* The name of the webhook.
*/
public val name: kotlin.String = requireNotNull(builder.name) { "A non-null value must be provided for name" }
/**
* The name of the action in a pipeline you want to connect to the webhook. The action must be from the source (first) stage of the pipeline.
*/
public val targetAction: kotlin.String = requireNotNull(builder.targetAction) { "A non-null value must be provided for targetAction" }
/**
* The name of the pipeline you want to connect to the webhook.
*/
public val targetPipeline: kotlin.String = requireNotNull(builder.targetPipeline) { "A non-null value must be provided for targetPipeline" }
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.codepipeline.model.WebhookDefinition = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("WebhookDefinition(")
append("authentication=$authentication,")
append("authenticationConfiguration=$authenticationConfiguration,")
append("filters=$filters,")
append("name=$name,")
append("targetAction=$targetAction,")
append("targetPipeline=$targetPipeline")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = authentication.hashCode()
result = 31 * result + (authenticationConfiguration?.hashCode() ?: 0)
result = 31 * result + (filters.hashCode())
result = 31 * result + (name.hashCode())
result = 31 * result + (targetAction.hashCode())
result = 31 * result + (targetPipeline.hashCode())
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as WebhookDefinition
if (authentication != other.authentication) return false
if (authenticationConfiguration != other.authenticationConfiguration) return false
if (filters != other.filters) return false
if (name != other.name) return false
if (targetAction != other.targetAction) return false
if (targetPipeline != other.targetPipeline) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.codepipeline.model.WebhookDefinition = Builder(this).apply(block).build()
public class Builder {
/**
* Supported options are GITHUB_HMAC, IP, and UNAUTHENTICATED.
* + For information about the authentication scheme implemented by GITHUB_HMAC, see [Securing your webhooks](https://developer.github.com/webhooks/securing/) on the GitHub Developer website.
* + IP rejects webhooks trigger requests unless they originate from an IP address in the IP range whitelisted in the authentication configuration.
* + UNAUTHENTICATED accepts all webhook trigger requests regardless of origin.
*/
public var authentication: aws.sdk.kotlin.services.codepipeline.model.WebhookAuthenticationType? = null
/**
* Properties that configure the authentication applied to incoming webhook trigger requests. The required properties depend on the authentication type. For GITHUB_HMAC, only the `SecretToken `property must be set. For IP, only the `AllowedIPRange `property must be set to a valid CIDR range. For UNAUTHENTICATED, no properties can be set.
*/
public var authenticationConfiguration: aws.sdk.kotlin.services.codepipeline.model.WebhookAuthConfiguration? = null
/**
* A list of rules applied to the body/payload sent in the POST request to a webhook URL. All defined rules must pass for the request to be accepted and the pipeline started.
*/
public var filters: List? = null
/**
* The name of the webhook.
*/
public var name: kotlin.String? = null
/**
* The name of the action in a pipeline you want to connect to the webhook. The action must be from the source (first) stage of the pipeline.
*/
public var targetAction: kotlin.String? = null
/**
* The name of the pipeline you want to connect to the webhook.
*/
public var targetPipeline: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.codepipeline.model.WebhookDefinition) : this() {
this.authentication = x.authentication
this.authenticationConfiguration = x.authenticationConfiguration
this.filters = x.filters
this.name = x.name
this.targetAction = x.targetAction
this.targetPipeline = x.targetPipeline
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.codepipeline.model.WebhookDefinition = WebhookDefinition(this)
/**
* construct an [aws.sdk.kotlin.services.codepipeline.model.WebhookAuthConfiguration] inside the given [block]
*/
public fun authenticationConfiguration(block: aws.sdk.kotlin.services.codepipeline.model.WebhookAuthConfiguration.Builder.() -> kotlin.Unit) {
this.authenticationConfiguration = aws.sdk.kotlin.services.codepipeline.model.WebhookAuthConfiguration.invoke(block)
}
internal fun correctErrors(): Builder {
if (authentication == null) authentication = WebhookAuthenticationType.SdkUnknown("no value provided")
if (filters == null) filters = emptyList()
if (name == null) name = ""
if (targetAction == null) targetAction = ""
if (targetPipeline == null) targetPipeline = ""
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy