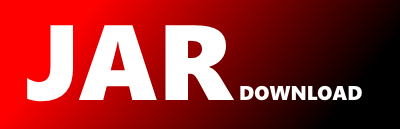
commonMain.aws.sdk.kotlin.services.codepipeline.model.ActionExecution.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of codepipeline-jvm Show documentation
Show all versions of codepipeline-jvm Show documentation
The AWS SDK for Kotlin client for CodePipeline
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.codepipeline.model
import aws.smithy.kotlin.runtime.SdkDsl
import aws.smithy.kotlin.runtime.time.Instant
/**
* Represents information about the run of an action.
*/
public class ActionExecution private constructor(builder: Builder) {
/**
* ID of the workflow action execution in the current stage. Use the GetPipelineState action to retrieve the current action execution details of the current stage.
*
* For older executions, this field might be empty. The action execution ID is available for executions run on or after March 2020.
*/
public val actionExecutionId: kotlin.String? = builder.actionExecutionId
/**
* The details of an error returned by a URL external to Amazon Web Services.
*/
public val errorDetails: aws.sdk.kotlin.services.codepipeline.model.ErrorDetails? = builder.errorDetails
/**
* The external ID of the run of the action.
*/
public val externalExecutionId: kotlin.String? = builder.externalExecutionId
/**
* The URL of a resource external to Amazon Web Services that is used when running the action (for example, an external repository URL).
*/
public val externalExecutionUrl: kotlin.String? = builder.externalExecutionUrl
/**
* The last status change of the action.
*/
public val lastStatusChange: aws.smithy.kotlin.runtime.time.Instant? = builder.lastStatusChange
/**
* The ARN of the user who last changed the pipeline.
*/
public val lastUpdatedBy: kotlin.String? = builder.lastUpdatedBy
/**
* A percentage of completeness of the action as it runs.
*/
public val percentComplete: kotlin.Int? = builder.percentComplete
/**
* The status of the action, or for a completed action, the last status of the action.
*/
public val status: aws.sdk.kotlin.services.codepipeline.model.ActionExecutionStatus? = builder.status
/**
* A summary of the run of the action.
*/
public val summary: kotlin.String? = builder.summary
/**
* The system-generated token used to identify a unique approval request. The token for each open approval request can be obtained using the `GetPipelineState` command. It is used to validate that the approval request corresponding to this token is still valid.
*/
public val token: kotlin.String? = builder.token
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.codepipeline.model.ActionExecution = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("ActionExecution(")
append("actionExecutionId=$actionExecutionId,")
append("errorDetails=$errorDetails,")
append("externalExecutionId=$externalExecutionId,")
append("externalExecutionUrl=$externalExecutionUrl,")
append("lastStatusChange=$lastStatusChange,")
append("lastUpdatedBy=$lastUpdatedBy,")
append("percentComplete=$percentComplete,")
append("status=$status,")
append("summary=$summary,")
append("token=$token")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = actionExecutionId?.hashCode() ?: 0
result = 31 * result + (errorDetails?.hashCode() ?: 0)
result = 31 * result + (externalExecutionId?.hashCode() ?: 0)
result = 31 * result + (externalExecutionUrl?.hashCode() ?: 0)
result = 31 * result + (lastStatusChange?.hashCode() ?: 0)
result = 31 * result + (lastUpdatedBy?.hashCode() ?: 0)
result = 31 * result + (percentComplete ?: 0)
result = 31 * result + (status?.hashCode() ?: 0)
result = 31 * result + (summary?.hashCode() ?: 0)
result = 31 * result + (token?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as ActionExecution
if (actionExecutionId != other.actionExecutionId) return false
if (errorDetails != other.errorDetails) return false
if (externalExecutionId != other.externalExecutionId) return false
if (externalExecutionUrl != other.externalExecutionUrl) return false
if (lastStatusChange != other.lastStatusChange) return false
if (lastUpdatedBy != other.lastUpdatedBy) return false
if (percentComplete != other.percentComplete) return false
if (status != other.status) return false
if (summary != other.summary) return false
if (token != other.token) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.codepipeline.model.ActionExecution = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* ID of the workflow action execution in the current stage. Use the GetPipelineState action to retrieve the current action execution details of the current stage.
*
* For older executions, this field might be empty. The action execution ID is available for executions run on or after March 2020.
*/
public var actionExecutionId: kotlin.String? = null
/**
* The details of an error returned by a URL external to Amazon Web Services.
*/
public var errorDetails: aws.sdk.kotlin.services.codepipeline.model.ErrorDetails? = null
/**
* The external ID of the run of the action.
*/
public var externalExecutionId: kotlin.String? = null
/**
* The URL of a resource external to Amazon Web Services that is used when running the action (for example, an external repository URL).
*/
public var externalExecutionUrl: kotlin.String? = null
/**
* The last status change of the action.
*/
public var lastStatusChange: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The ARN of the user who last changed the pipeline.
*/
public var lastUpdatedBy: kotlin.String? = null
/**
* A percentage of completeness of the action as it runs.
*/
public var percentComplete: kotlin.Int? = null
/**
* The status of the action, or for a completed action, the last status of the action.
*/
public var status: aws.sdk.kotlin.services.codepipeline.model.ActionExecutionStatus? = null
/**
* A summary of the run of the action.
*/
public var summary: kotlin.String? = null
/**
* The system-generated token used to identify a unique approval request. The token for each open approval request can be obtained using the `GetPipelineState` command. It is used to validate that the approval request corresponding to this token is still valid.
*/
public var token: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.codepipeline.model.ActionExecution) : this() {
this.actionExecutionId = x.actionExecutionId
this.errorDetails = x.errorDetails
this.externalExecutionId = x.externalExecutionId
this.externalExecutionUrl = x.externalExecutionUrl
this.lastStatusChange = x.lastStatusChange
this.lastUpdatedBy = x.lastUpdatedBy
this.percentComplete = x.percentComplete
this.status = x.status
this.summary = x.summary
this.token = x.token
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.codepipeline.model.ActionExecution = ActionExecution(this)
/**
* construct an [aws.sdk.kotlin.services.codepipeline.model.ErrorDetails] inside the given [block]
*/
public fun errorDetails(block: aws.sdk.kotlin.services.codepipeline.model.ErrorDetails.Builder.() -> kotlin.Unit) {
this.errorDetails = aws.sdk.kotlin.services.codepipeline.model.ErrorDetails.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy