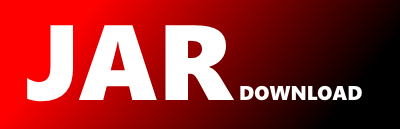
commonMain.aws.sdk.kotlin.services.codepipeline.model.Artifact.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of codepipeline-jvm Show documentation
Show all versions of codepipeline-jvm Show documentation
The AWS SDK for Kotlin client for CodePipeline
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.codepipeline.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Artifacts are the files that are worked on by actions in the pipeline. See the action configuration for each action for details about artifact parameters. For example, the S3 source action artifact is a file name (or file path), and the files are generally provided as a ZIP file. Example artifact name: SampleApp_Windows.zip
*/
public class Artifact private constructor(builder: Builder) {
/**
* The location of an artifact.
*/
public val location: aws.sdk.kotlin.services.codepipeline.model.ArtifactLocation? = builder.location
/**
* The artifact's name.
*/
public val name: kotlin.String? = builder.name
/**
* The artifact's revision ID. Depending on the type of object, this could be a commit ID (GitHub) or a revision ID (Amazon S3).
*/
public val revision: kotlin.String? = builder.revision
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.codepipeline.model.Artifact = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("Artifact(")
append("location=$location,")
append("name=$name,")
append("revision=$revision")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = location?.hashCode() ?: 0
result = 31 * result + (name?.hashCode() ?: 0)
result = 31 * result + (revision?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as Artifact
if (location != other.location) return false
if (name != other.name) return false
if (revision != other.revision) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.codepipeline.model.Artifact = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The location of an artifact.
*/
public var location: aws.sdk.kotlin.services.codepipeline.model.ArtifactLocation? = null
/**
* The artifact's name.
*/
public var name: kotlin.String? = null
/**
* The artifact's revision ID. Depending on the type of object, this could be a commit ID (GitHub) or a revision ID (Amazon S3).
*/
public var revision: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.codepipeline.model.Artifact) : this() {
this.location = x.location
this.name = x.name
this.revision = x.revision
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.codepipeline.model.Artifact = Artifact(this)
/**
* construct an [aws.sdk.kotlin.services.codepipeline.model.ArtifactLocation] inside the given [block]
*/
public fun location(block: aws.sdk.kotlin.services.codepipeline.model.ArtifactLocation.Builder.() -> kotlin.Unit) {
this.location = aws.sdk.kotlin.services.codepipeline.model.ArtifactLocation.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy