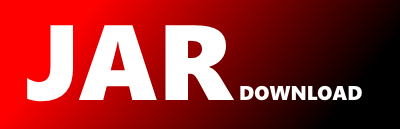
commonMain.aws.sdk.kotlin.services.codepipeline.model.AwsSessionCredentials.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of codepipeline-jvm Show documentation
Show all versions of codepipeline-jvm Show documentation
The AWS SDK for Kotlin client for CodePipeline
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.codepipeline.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Represents an Amazon Web Services session credentials object. These credentials are temporary credentials that are issued by Amazon Web Services Secure Token Service (STS). They can be used to access input and output artifacts in the S3 bucket used to store artifact for the pipeline in CodePipeline.
*/
public class AwsSessionCredentials private constructor(builder: Builder) {
/**
* The access key for the session.
*/
public val accessKeyId: kotlin.String = requireNotNull(builder.accessKeyId) { "A non-null value must be provided for accessKeyId" }
/**
* The secret access key for the session.
*/
public val secretAccessKey: kotlin.String = requireNotNull(builder.secretAccessKey) { "A non-null value must be provided for secretAccessKey" }
/**
* The token for the session.
*/
public val sessionToken: kotlin.String = requireNotNull(builder.sessionToken) { "A non-null value must be provided for sessionToken" }
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.codepipeline.model.AwsSessionCredentials = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("AwsSessionCredentials(")
append("*** Sensitive Data Redacted ***")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = accessKeyId.hashCode()
result = 31 * result + (secretAccessKey.hashCode())
result = 31 * result + (sessionToken.hashCode())
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as AwsSessionCredentials
if (accessKeyId != other.accessKeyId) return false
if (secretAccessKey != other.secretAccessKey) return false
if (sessionToken != other.sessionToken) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.codepipeline.model.AwsSessionCredentials = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The access key for the session.
*/
public var accessKeyId: kotlin.String? = null
/**
* The secret access key for the session.
*/
public var secretAccessKey: kotlin.String? = null
/**
* The token for the session.
*/
public var sessionToken: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.codepipeline.model.AwsSessionCredentials) : this() {
this.accessKeyId = x.accessKeyId
this.secretAccessKey = x.secretAccessKey
this.sessionToken = x.sessionToken
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.codepipeline.model.AwsSessionCredentials = AwsSessionCredentials(this)
internal fun correctErrors(): Builder {
if (accessKeyId == null) accessKeyId = ""
if (secretAccessKey == null) secretAccessKey = ""
if (sessionToken == null) sessionToken = ""
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy