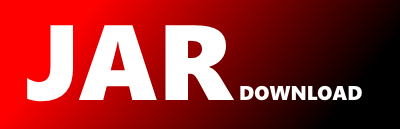
commonMain.aws.sdk.kotlin.services.codepipeline.model.PutJobSuccessResultRequest.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of codepipeline-jvm Show documentation
Show all versions of codepipeline-jvm Show documentation
The AWS SDK for Kotlin client for CodePipeline
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.codepipeline.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Represents the input of a `PutJobSuccessResult` action.
*/
public class PutJobSuccessResultRequest private constructor(builder: Builder) {
/**
* A token generated by a job worker, such as a CodeDeploy deployment ID, that a successful job provides to identify a custom action in progress. Future jobs use this token to identify the running instance of the action. It can be reused to return more information about the progress of the custom action. When the action is complete, no continuation token should be supplied.
*/
public val continuationToken: kotlin.String? = builder.continuationToken
/**
* The ID of the current revision of the artifact successfully worked on by the job.
*/
public val currentRevision: aws.sdk.kotlin.services.codepipeline.model.CurrentRevision? = builder.currentRevision
/**
* The execution details of the successful job, such as the actions taken by the job worker.
*/
public val executionDetails: aws.sdk.kotlin.services.codepipeline.model.ExecutionDetails? = builder.executionDetails
/**
* The unique system-generated ID of the job that succeeded. This is the same ID returned from `PollForJobs`.
*/
public val jobId: kotlin.String? = builder.jobId
/**
* Key-value pairs produced as output by a job worker that can be made available to a downstream action configuration. `outputVariables` can be included only when there is no continuation token on the request.
*/
public val outputVariables: Map? = builder.outputVariables
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.codepipeline.model.PutJobSuccessResultRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("PutJobSuccessResultRequest(")
append("continuationToken=$continuationToken,")
append("currentRevision=$currentRevision,")
append("executionDetails=$executionDetails,")
append("jobId=$jobId,")
append("outputVariables=$outputVariables")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = continuationToken?.hashCode() ?: 0
result = 31 * result + (currentRevision?.hashCode() ?: 0)
result = 31 * result + (executionDetails?.hashCode() ?: 0)
result = 31 * result + (jobId?.hashCode() ?: 0)
result = 31 * result + (outputVariables?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as PutJobSuccessResultRequest
if (continuationToken != other.continuationToken) return false
if (currentRevision != other.currentRevision) return false
if (executionDetails != other.executionDetails) return false
if (jobId != other.jobId) return false
if (outputVariables != other.outputVariables) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.codepipeline.model.PutJobSuccessResultRequest = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* A token generated by a job worker, such as a CodeDeploy deployment ID, that a successful job provides to identify a custom action in progress. Future jobs use this token to identify the running instance of the action. It can be reused to return more information about the progress of the custom action. When the action is complete, no continuation token should be supplied.
*/
public var continuationToken: kotlin.String? = null
/**
* The ID of the current revision of the artifact successfully worked on by the job.
*/
public var currentRevision: aws.sdk.kotlin.services.codepipeline.model.CurrentRevision? = null
/**
* The execution details of the successful job, such as the actions taken by the job worker.
*/
public var executionDetails: aws.sdk.kotlin.services.codepipeline.model.ExecutionDetails? = null
/**
* The unique system-generated ID of the job that succeeded. This is the same ID returned from `PollForJobs`.
*/
public var jobId: kotlin.String? = null
/**
* Key-value pairs produced as output by a job worker that can be made available to a downstream action configuration. `outputVariables` can be included only when there is no continuation token on the request.
*/
public var outputVariables: Map? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.codepipeline.model.PutJobSuccessResultRequest) : this() {
this.continuationToken = x.continuationToken
this.currentRevision = x.currentRevision
this.executionDetails = x.executionDetails
this.jobId = x.jobId
this.outputVariables = x.outputVariables
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.codepipeline.model.PutJobSuccessResultRequest = PutJobSuccessResultRequest(this)
/**
* construct an [aws.sdk.kotlin.services.codepipeline.model.CurrentRevision] inside the given [block]
*/
public fun currentRevision(block: aws.sdk.kotlin.services.codepipeline.model.CurrentRevision.Builder.() -> kotlin.Unit) {
this.currentRevision = aws.sdk.kotlin.services.codepipeline.model.CurrentRevision.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.codepipeline.model.ExecutionDetails] inside the given [block]
*/
public fun executionDetails(block: aws.sdk.kotlin.services.codepipeline.model.ExecutionDetails.Builder.() -> kotlin.Unit) {
this.executionDetails = aws.sdk.kotlin.services.codepipeline.model.ExecutionDetails.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy