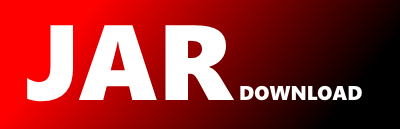
commonMain.aws.sdk.kotlin.services.codepipeline.model.PutThirdPartyJobSuccessResultRequest.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of codepipeline-jvm Show documentation
Show all versions of codepipeline-jvm Show documentation
The AWS SDK for Kotlin client for CodePipeline
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.codepipeline.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Represents the input of a `PutThirdPartyJobSuccessResult` action.
*/
public class PutThirdPartyJobSuccessResultRequest private constructor(builder: Builder) {
/**
* The clientToken portion of the clientId and clientToken pair used to verify that the calling entity is allowed access to the job and its details.
*/
public val clientToken: kotlin.String? = builder.clientToken
/**
* A token generated by a job worker, such as a CodeDeploy deployment ID, that a successful job provides to identify a partner action in progress. Future jobs use this token to identify the running instance of the action. It can be reused to return more information about the progress of the partner action. When the action is complete, no continuation token should be supplied.
*/
public val continuationToken: kotlin.String? = builder.continuationToken
/**
* Represents information about a current revision.
*/
public val currentRevision: aws.sdk.kotlin.services.codepipeline.model.CurrentRevision? = builder.currentRevision
/**
* The details of the actions taken and results produced on an artifact as it passes through stages in the pipeline.
*/
public val executionDetails: aws.sdk.kotlin.services.codepipeline.model.ExecutionDetails? = builder.executionDetails
/**
* The ID of the job that successfully completed. This is the same ID returned from `PollForThirdPartyJobs`.
*/
public val jobId: kotlin.String? = builder.jobId
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.codepipeline.model.PutThirdPartyJobSuccessResultRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("PutThirdPartyJobSuccessResultRequest(")
append("clientToken=$clientToken,")
append("continuationToken=$continuationToken,")
append("currentRevision=$currentRevision,")
append("executionDetails=$executionDetails,")
append("jobId=$jobId")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = clientToken?.hashCode() ?: 0
result = 31 * result + (continuationToken?.hashCode() ?: 0)
result = 31 * result + (currentRevision?.hashCode() ?: 0)
result = 31 * result + (executionDetails?.hashCode() ?: 0)
result = 31 * result + (jobId?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as PutThirdPartyJobSuccessResultRequest
if (clientToken != other.clientToken) return false
if (continuationToken != other.continuationToken) return false
if (currentRevision != other.currentRevision) return false
if (executionDetails != other.executionDetails) return false
if (jobId != other.jobId) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.codepipeline.model.PutThirdPartyJobSuccessResultRequest = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The clientToken portion of the clientId and clientToken pair used to verify that the calling entity is allowed access to the job and its details.
*/
public var clientToken: kotlin.String? = null
/**
* A token generated by a job worker, such as a CodeDeploy deployment ID, that a successful job provides to identify a partner action in progress. Future jobs use this token to identify the running instance of the action. It can be reused to return more information about the progress of the partner action. When the action is complete, no continuation token should be supplied.
*/
public var continuationToken: kotlin.String? = null
/**
* Represents information about a current revision.
*/
public var currentRevision: aws.sdk.kotlin.services.codepipeline.model.CurrentRevision? = null
/**
* The details of the actions taken and results produced on an artifact as it passes through stages in the pipeline.
*/
public var executionDetails: aws.sdk.kotlin.services.codepipeline.model.ExecutionDetails? = null
/**
* The ID of the job that successfully completed. This is the same ID returned from `PollForThirdPartyJobs`.
*/
public var jobId: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.codepipeline.model.PutThirdPartyJobSuccessResultRequest) : this() {
this.clientToken = x.clientToken
this.continuationToken = x.continuationToken
this.currentRevision = x.currentRevision
this.executionDetails = x.executionDetails
this.jobId = x.jobId
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.codepipeline.model.PutThirdPartyJobSuccessResultRequest = PutThirdPartyJobSuccessResultRequest(this)
/**
* construct an [aws.sdk.kotlin.services.codepipeline.model.CurrentRevision] inside the given [block]
*/
public fun currentRevision(block: aws.sdk.kotlin.services.codepipeline.model.CurrentRevision.Builder.() -> kotlin.Unit) {
this.currentRevision = aws.sdk.kotlin.services.codepipeline.model.CurrentRevision.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.codepipeline.model.ExecutionDetails] inside the given [block]
*/
public fun executionDetails(block: aws.sdk.kotlin.services.codepipeline.model.ExecutionDetails.Builder.() -> kotlin.Unit) {
this.executionDetails = aws.sdk.kotlin.services.codepipeline.model.ExecutionDetails.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy