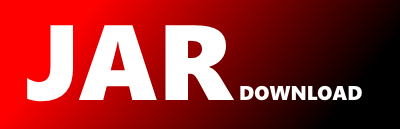
commonMain.aws.sdk.kotlin.services.codepipeline.model.RuleState.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of codepipeline-jvm Show documentation
Show all versions of codepipeline-jvm Show documentation
The AWS SDK for Kotlin client for CodePipeline
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.codepipeline.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Returns information about the state of a rule.
*
* Values returned in the `revisionId` field indicate the rule revision information, such as the commit ID, for the current state.
*/
public class RuleState private constructor(builder: Builder) {
/**
* The ID of the current revision of the artifact successfully worked on by the job.
*/
public val currentRevision: aws.sdk.kotlin.services.codepipeline.model.RuleRevision? = builder.currentRevision
/**
* A URL link for more information about the state of the action, such as a details page.
*/
public val entityUrl: kotlin.String? = builder.entityUrl
/**
* Represents information about the latest run of an rule.
*/
public val latestExecution: aws.sdk.kotlin.services.codepipeline.model.RuleExecution? = builder.latestExecution
/**
* A URL link for more information about the revision, such as a commit details page.
*/
public val revisionUrl: kotlin.String? = builder.revisionUrl
/**
* The name of the rule.
*/
public val ruleName: kotlin.String? = builder.ruleName
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.codepipeline.model.RuleState = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("RuleState(")
append("currentRevision=$currentRevision,")
append("entityUrl=$entityUrl,")
append("latestExecution=$latestExecution,")
append("revisionUrl=$revisionUrl,")
append("ruleName=$ruleName")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = currentRevision?.hashCode() ?: 0
result = 31 * result + (entityUrl?.hashCode() ?: 0)
result = 31 * result + (latestExecution?.hashCode() ?: 0)
result = 31 * result + (revisionUrl?.hashCode() ?: 0)
result = 31 * result + (ruleName?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as RuleState
if (currentRevision != other.currentRevision) return false
if (entityUrl != other.entityUrl) return false
if (latestExecution != other.latestExecution) return false
if (revisionUrl != other.revisionUrl) return false
if (ruleName != other.ruleName) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.codepipeline.model.RuleState = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The ID of the current revision of the artifact successfully worked on by the job.
*/
public var currentRevision: aws.sdk.kotlin.services.codepipeline.model.RuleRevision? = null
/**
* A URL link for more information about the state of the action, such as a details page.
*/
public var entityUrl: kotlin.String? = null
/**
* Represents information about the latest run of an rule.
*/
public var latestExecution: aws.sdk.kotlin.services.codepipeline.model.RuleExecution? = null
/**
* A URL link for more information about the revision, such as a commit details page.
*/
public var revisionUrl: kotlin.String? = null
/**
* The name of the rule.
*/
public var ruleName: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.codepipeline.model.RuleState) : this() {
this.currentRevision = x.currentRevision
this.entityUrl = x.entityUrl
this.latestExecution = x.latestExecution
this.revisionUrl = x.revisionUrl
this.ruleName = x.ruleName
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.codepipeline.model.RuleState = RuleState(this)
/**
* construct an [aws.sdk.kotlin.services.codepipeline.model.RuleRevision] inside the given [block]
*/
public fun currentRevision(block: aws.sdk.kotlin.services.codepipeline.model.RuleRevision.Builder.() -> kotlin.Unit) {
this.currentRevision = aws.sdk.kotlin.services.codepipeline.model.RuleRevision.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.codepipeline.model.RuleExecution] inside the given [block]
*/
public fun latestExecution(block: aws.sdk.kotlin.services.codepipeline.model.RuleExecution.Builder.() -> kotlin.Unit) {
this.latestExecution = aws.sdk.kotlin.services.codepipeline.model.RuleExecution.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy