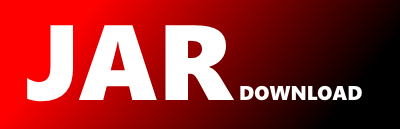
commonMain.aws.sdk.kotlin.services.codepipeline.model.ActionExecutionDetail.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of codepipeline Show documentation
Show all versions of codepipeline Show documentation
The AWS Kotlin client for CodePipeline
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.codepipeline.model
import aws.smithy.kotlin.runtime.time.Instant
/**
* Returns information about an execution of an action, including the action execution ID, and the name, version, and timing of the action.
*/
class ActionExecutionDetail private constructor(builder: Builder) {
/**
* The action execution ID.
*/
val actionExecutionId: kotlin.String? = builder.actionExecutionId
/**
* The name of the action.
*/
val actionName: kotlin.String? = builder.actionName
/**
* Input details for the action execution, such as role ARN, Region, and input artifacts.
*/
val input: aws.sdk.kotlin.services.codepipeline.model.ActionExecutionInput? = builder.input
/**
* The last update time of the action execution.
*/
val lastUpdateTime: aws.smithy.kotlin.runtime.time.Instant? = builder.lastUpdateTime
/**
* Output details for the action execution, such as the action execution result.
*/
val output: aws.sdk.kotlin.services.codepipeline.model.ActionExecutionOutput? = builder.output
/**
* The pipeline execution ID for the action execution.
*/
val pipelineExecutionId: kotlin.String? = builder.pipelineExecutionId
/**
* The version of the pipeline where the action was run.
*/
val pipelineVersion: kotlin.Int? = builder.pipelineVersion
/**
* The name of the stage that contains the action.
*/
val stageName: kotlin.String? = builder.stageName
/**
* The start time of the action execution.
*/
val startTime: aws.smithy.kotlin.runtime.time.Instant? = builder.startTime
/**
* The status of the action execution. Status categories are `InProgress`, `Succeeded`, and `Failed`.
*/
val status: aws.sdk.kotlin.services.codepipeline.model.ActionExecutionStatus? = builder.status
companion object {
operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.codepipeline.model.ActionExecutionDetail = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("ActionExecutionDetail(")
append("actionExecutionId=$actionExecutionId,")
append("actionName=$actionName,")
append("input=$input,")
append("lastUpdateTime=$lastUpdateTime,")
append("output=$output,")
append("pipelineExecutionId=$pipelineExecutionId,")
append("pipelineVersion=$pipelineVersion,")
append("stageName=$stageName,")
append("startTime=$startTime,")
append("status=$status)")
}
override fun hashCode(): kotlin.Int {
var result = actionExecutionId?.hashCode() ?: 0
result = 31 * result + (actionName?.hashCode() ?: 0)
result = 31 * result + (input?.hashCode() ?: 0)
result = 31 * result + (lastUpdateTime?.hashCode() ?: 0)
result = 31 * result + (output?.hashCode() ?: 0)
result = 31 * result + (pipelineExecutionId?.hashCode() ?: 0)
result = 31 * result + (pipelineVersion ?: 0)
result = 31 * result + (stageName?.hashCode() ?: 0)
result = 31 * result + (startTime?.hashCode() ?: 0)
result = 31 * result + (status?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as ActionExecutionDetail
if (actionExecutionId != other.actionExecutionId) return false
if (actionName != other.actionName) return false
if (input != other.input) return false
if (lastUpdateTime != other.lastUpdateTime) return false
if (output != other.output) return false
if (pipelineExecutionId != other.pipelineExecutionId) return false
if (pipelineVersion != other.pipelineVersion) return false
if (stageName != other.stageName) return false
if (startTime != other.startTime) return false
if (status != other.status) return false
return true
}
inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.codepipeline.model.ActionExecutionDetail = Builder(this).apply(block).build()
class Builder {
/**
* The action execution ID.
*/
var actionExecutionId: kotlin.String? = null
/**
* The name of the action.
*/
var actionName: kotlin.String? = null
/**
* Input details for the action execution, such as role ARN, Region, and input artifacts.
*/
var input: aws.sdk.kotlin.services.codepipeline.model.ActionExecutionInput? = null
/**
* The last update time of the action execution.
*/
var lastUpdateTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* Output details for the action execution, such as the action execution result.
*/
var output: aws.sdk.kotlin.services.codepipeline.model.ActionExecutionOutput? = null
/**
* The pipeline execution ID for the action execution.
*/
var pipelineExecutionId: kotlin.String? = null
/**
* The version of the pipeline where the action was run.
*/
var pipelineVersion: kotlin.Int? = null
/**
* The name of the stage that contains the action.
*/
var stageName: kotlin.String? = null
/**
* The start time of the action execution.
*/
var startTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The status of the action execution. Status categories are `InProgress`, `Succeeded`, and `Failed`.
*/
var status: aws.sdk.kotlin.services.codepipeline.model.ActionExecutionStatus? = null
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.codepipeline.model.ActionExecutionDetail) : this() {
this.actionExecutionId = x.actionExecutionId
this.actionName = x.actionName
this.input = x.input
this.lastUpdateTime = x.lastUpdateTime
this.output = x.output
this.pipelineExecutionId = x.pipelineExecutionId
this.pipelineVersion = x.pipelineVersion
this.stageName = x.stageName
this.startTime = x.startTime
this.status = x.status
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.codepipeline.model.ActionExecutionDetail = ActionExecutionDetail(this)
/**
* construct an [aws.sdk.kotlin.services.codepipeline.model.ActionExecutionInput] inside the given [block]
*/
fun input(block: aws.sdk.kotlin.services.codepipeline.model.ActionExecutionInput.Builder.() -> kotlin.Unit) {
this.input = aws.sdk.kotlin.services.codepipeline.model.ActionExecutionInput.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.codepipeline.model.ActionExecutionOutput] inside the given [block]
*/
fun output(block: aws.sdk.kotlin.services.codepipeline.model.ActionExecutionOutput.Builder.() -> kotlin.Unit) {
this.output = aws.sdk.kotlin.services.codepipeline.model.ActionExecutionOutput.invoke(block)
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy