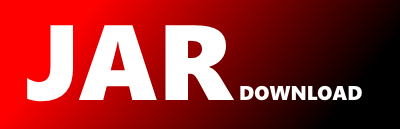
commonMain.aws.sdk.kotlin.services.codepipeline.model.PipelineExecutionSummary.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of codepipeline Show documentation
Show all versions of codepipeline Show documentation
The AWS Kotlin client for CodePipeline
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.codepipeline.model
import aws.smithy.kotlin.runtime.time.Instant
/**
* Summary information about a pipeline execution.
*/
public class PipelineExecutionSummary private constructor(builder: Builder) {
/**
* The date and time of the last change to the pipeline execution, in timestamp format.
*/
public val lastUpdateTime: aws.smithy.kotlin.runtime.time.Instant? = builder.lastUpdateTime
/**
* The ID of the pipeline execution.
*/
public val pipelineExecutionId: kotlin.String? = builder.pipelineExecutionId
/**
* A list of the source artifact revisions that initiated a pipeline execution.
*/
public val sourceRevisions: List? = builder.sourceRevisions
/**
* The date and time when the pipeline execution began, in timestamp format.
*/
public val startTime: aws.smithy.kotlin.runtime.time.Instant? = builder.startTime
/**
* The status of the pipeline execution.
* + InProgress: The pipeline execution is currently running.
* + Stopped: The pipeline execution was manually stopped. For more information, see [Stopped Executions](https://docs.aws.amazon.com/codepipeline/latest/userguide/concepts.html#concepts-executions-stopped).
* + Stopping: The pipeline execution received a request to be manually stopped. Depending on the selected stop mode, the execution is either completing or abandoning in-progress actions. For more information, see [Stopped Executions](https://docs.aws.amazon.com/codepipeline/latest/userguide/concepts.html#concepts-executions-stopped).
* + Succeeded: The pipeline execution was completed successfully.
* + Superseded: While this pipeline execution was waiting for the next stage to be completed, a newer pipeline execution advanced and continued through the pipeline instead. For more information, see [Superseded Executions](https://docs.aws.amazon.com/codepipeline/latest/userguide/concepts.html#concepts-superseded).
* + Failed: The pipeline execution was not completed successfully.
*/
public val status: aws.sdk.kotlin.services.codepipeline.model.PipelineExecutionStatus? = builder.status
/**
* The interaction that stopped a pipeline execution.
*/
public val stopTrigger: aws.sdk.kotlin.services.codepipeline.model.StopExecutionTrigger? = builder.stopTrigger
/**
* The interaction or event that started a pipeline execution, such as automated change detection or a `StartPipelineExecution` API call.
*/
public val trigger: aws.sdk.kotlin.services.codepipeline.model.ExecutionTrigger? = builder.trigger
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.codepipeline.model.PipelineExecutionSummary = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("PipelineExecutionSummary(")
append("lastUpdateTime=$lastUpdateTime,")
append("pipelineExecutionId=$pipelineExecutionId,")
append("sourceRevisions=$sourceRevisions,")
append("startTime=$startTime,")
append("status=$status,")
append("stopTrigger=$stopTrigger,")
append("trigger=$trigger")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = lastUpdateTime?.hashCode() ?: 0
result = 31 * result + (pipelineExecutionId?.hashCode() ?: 0)
result = 31 * result + (sourceRevisions?.hashCode() ?: 0)
result = 31 * result + (startTime?.hashCode() ?: 0)
result = 31 * result + (status?.hashCode() ?: 0)
result = 31 * result + (stopTrigger?.hashCode() ?: 0)
result = 31 * result + (trigger?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as PipelineExecutionSummary
if (lastUpdateTime != other.lastUpdateTime) return false
if (pipelineExecutionId != other.pipelineExecutionId) return false
if (sourceRevisions != other.sourceRevisions) return false
if (startTime != other.startTime) return false
if (status != other.status) return false
if (stopTrigger != other.stopTrigger) return false
if (trigger != other.trigger) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.codepipeline.model.PipelineExecutionSummary = Builder(this).apply(block).build()
public class Builder {
/**
* The date and time of the last change to the pipeline execution, in timestamp format.
*/
public var lastUpdateTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The ID of the pipeline execution.
*/
public var pipelineExecutionId: kotlin.String? = null
/**
* A list of the source artifact revisions that initiated a pipeline execution.
*/
public var sourceRevisions: List? = null
/**
* The date and time when the pipeline execution began, in timestamp format.
*/
public var startTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The status of the pipeline execution.
* + InProgress: The pipeline execution is currently running.
* + Stopped: The pipeline execution was manually stopped. For more information, see [Stopped Executions](https://docs.aws.amazon.com/codepipeline/latest/userguide/concepts.html#concepts-executions-stopped).
* + Stopping: The pipeline execution received a request to be manually stopped. Depending on the selected stop mode, the execution is either completing or abandoning in-progress actions. For more information, see [Stopped Executions](https://docs.aws.amazon.com/codepipeline/latest/userguide/concepts.html#concepts-executions-stopped).
* + Succeeded: The pipeline execution was completed successfully.
* + Superseded: While this pipeline execution was waiting for the next stage to be completed, a newer pipeline execution advanced and continued through the pipeline instead. For more information, see [Superseded Executions](https://docs.aws.amazon.com/codepipeline/latest/userguide/concepts.html#concepts-superseded).
* + Failed: The pipeline execution was not completed successfully.
*/
public var status: aws.sdk.kotlin.services.codepipeline.model.PipelineExecutionStatus? = null
/**
* The interaction that stopped a pipeline execution.
*/
public var stopTrigger: aws.sdk.kotlin.services.codepipeline.model.StopExecutionTrigger? = null
/**
* The interaction or event that started a pipeline execution, such as automated change detection or a `StartPipelineExecution` API call.
*/
public var trigger: aws.sdk.kotlin.services.codepipeline.model.ExecutionTrigger? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.codepipeline.model.PipelineExecutionSummary) : this() {
this.lastUpdateTime = x.lastUpdateTime
this.pipelineExecutionId = x.pipelineExecutionId
this.sourceRevisions = x.sourceRevisions
this.startTime = x.startTime
this.status = x.status
this.stopTrigger = x.stopTrigger
this.trigger = x.trigger
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.codepipeline.model.PipelineExecutionSummary = PipelineExecutionSummary(this)
/**
* construct an [aws.sdk.kotlin.services.codepipeline.model.StopExecutionTrigger] inside the given [block]
*/
public fun stopTrigger(block: aws.sdk.kotlin.services.codepipeline.model.StopExecutionTrigger.Builder.() -> kotlin.Unit) {
this.stopTrigger = aws.sdk.kotlin.services.codepipeline.model.StopExecutionTrigger.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.codepipeline.model.ExecutionTrigger] inside the given [block]
*/
public fun trigger(block: aws.sdk.kotlin.services.codepipeline.model.ExecutionTrigger.Builder.() -> kotlin.Unit) {
this.trigger = aws.sdk.kotlin.services.codepipeline.model.ExecutionTrigger.invoke(block)
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy