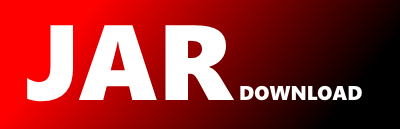
commonMain.aws.sdk.kotlin.services.codepipeline.model.PipelineContext.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of codepipeline Show documentation
Show all versions of codepipeline Show documentation
The AWS Kotlin client for CodePipeline
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.codepipeline.model
/**
* Represents information about a pipeline to a job worker.
*
* PipelineContext contains `pipelineArn` and `pipelineExecutionId` for custom action jobs. The `pipelineArn` and `pipelineExecutionId` fields are not populated for ThirdParty action jobs.
*/
public class PipelineContext private constructor(builder: Builder) {
/**
* The context of an action to a job worker in the stage of a pipeline.
*/
public val action: aws.sdk.kotlin.services.codepipeline.model.ActionContext? = builder.action
/**
* The Amazon Resource Name (ARN) of the pipeline.
*/
public val pipelineArn: kotlin.String? = builder.pipelineArn
/**
* The execution ID of the pipeline.
*/
public val pipelineExecutionId: kotlin.String? = builder.pipelineExecutionId
/**
* The name of the pipeline. This is a user-specified value. Pipeline names must be unique across all pipeline names under an Amazon Web Services account.
*/
public val pipelineName: kotlin.String? = builder.pipelineName
/**
* The stage of the pipeline.
*/
public val stage: aws.sdk.kotlin.services.codepipeline.model.StageContext? = builder.stage
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.codepipeline.model.PipelineContext = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("PipelineContext(")
append("action=$action,")
append("pipelineArn=$pipelineArn,")
append("pipelineExecutionId=$pipelineExecutionId,")
append("pipelineName=$pipelineName,")
append("stage=$stage")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = action?.hashCode() ?: 0
result = 31 * result + (pipelineArn?.hashCode() ?: 0)
result = 31 * result + (pipelineExecutionId?.hashCode() ?: 0)
result = 31 * result + (pipelineName?.hashCode() ?: 0)
result = 31 * result + (stage?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as PipelineContext
if (action != other.action) return false
if (pipelineArn != other.pipelineArn) return false
if (pipelineExecutionId != other.pipelineExecutionId) return false
if (pipelineName != other.pipelineName) return false
if (stage != other.stage) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.codepipeline.model.PipelineContext = Builder(this).apply(block).build()
public class Builder {
/**
* The context of an action to a job worker in the stage of a pipeline.
*/
public var action: aws.sdk.kotlin.services.codepipeline.model.ActionContext? = null
/**
* The Amazon Resource Name (ARN) of the pipeline.
*/
public var pipelineArn: kotlin.String? = null
/**
* The execution ID of the pipeline.
*/
public var pipelineExecutionId: kotlin.String? = null
/**
* The name of the pipeline. This is a user-specified value. Pipeline names must be unique across all pipeline names under an Amazon Web Services account.
*/
public var pipelineName: kotlin.String? = null
/**
* The stage of the pipeline.
*/
public var stage: aws.sdk.kotlin.services.codepipeline.model.StageContext? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.codepipeline.model.PipelineContext) : this() {
this.action = x.action
this.pipelineArn = x.pipelineArn
this.pipelineExecutionId = x.pipelineExecutionId
this.pipelineName = x.pipelineName
this.stage = x.stage
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.codepipeline.model.PipelineContext = PipelineContext(this)
/**
* construct an [aws.sdk.kotlin.services.codepipeline.model.ActionContext] inside the given [block]
*/
public fun action(block: aws.sdk.kotlin.services.codepipeline.model.ActionContext.Builder.() -> kotlin.Unit) {
this.action = aws.sdk.kotlin.services.codepipeline.model.ActionContext.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.codepipeline.model.StageContext] inside the given [block]
*/
public fun stage(block: aws.sdk.kotlin.services.codepipeline.model.StageContext.Builder.() -> kotlin.Unit) {
this.stage = aws.sdk.kotlin.services.codepipeline.model.StageContext.invoke(block)
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy