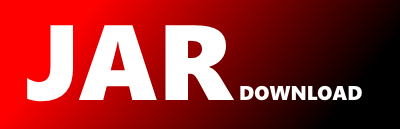
commonMain.aws.sdk.kotlin.services.codepipeline.model.PutApprovalResultRequest.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of codepipeline Show documentation
Show all versions of codepipeline Show documentation
The AWS Kotlin client for CodePipeline
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.codepipeline.model
/**
* Represents the input of a `PutApprovalResult` action.
*/
public class PutApprovalResultRequest private constructor(builder: Builder) {
/**
* The name of the action for which approval is requested.
*/
public val actionName: kotlin.String? = builder.actionName
/**
* The name of the pipeline that contains the action.
*/
public val pipelineName: kotlin.String? = builder.pipelineName
/**
* Represents information about the result of the approval request.
*/
public val result: aws.sdk.kotlin.services.codepipeline.model.ApprovalResult? = builder.result
/**
* The name of the stage that contains the action.
*/
public val stageName: kotlin.String? = builder.stageName
/**
* The system-generated token used to identify a unique approval request. The token for each open approval request can be obtained using the GetPipelineState action. It is used to validate that the approval request corresponding to this token is still valid.
*/
public val token: kotlin.String? = builder.token
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.codepipeline.model.PutApprovalResultRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("PutApprovalResultRequest(")
append("actionName=$actionName,")
append("pipelineName=$pipelineName,")
append("result=$result,")
append("stageName=$stageName,")
append("token=$token")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = actionName?.hashCode() ?: 0
result = 31 * result + (pipelineName?.hashCode() ?: 0)
result = 31 * result + (result?.hashCode() ?: 0)
result = 31 * result + (stageName?.hashCode() ?: 0)
result = 31 * result + (token?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as PutApprovalResultRequest
if (actionName != other.actionName) return false
if (pipelineName != other.pipelineName) return false
if (result != other.result) return false
if (stageName != other.stageName) return false
if (token != other.token) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.codepipeline.model.PutApprovalResultRequest = Builder(this).apply(block).build()
public class Builder {
/**
* The name of the action for which approval is requested.
*/
public var actionName: kotlin.String? = null
/**
* The name of the pipeline that contains the action.
*/
public var pipelineName: kotlin.String? = null
/**
* Represents information about the result of the approval request.
*/
public var result: aws.sdk.kotlin.services.codepipeline.model.ApprovalResult? = null
/**
* The name of the stage that contains the action.
*/
public var stageName: kotlin.String? = null
/**
* The system-generated token used to identify a unique approval request. The token for each open approval request can be obtained using the GetPipelineState action. It is used to validate that the approval request corresponding to this token is still valid.
*/
public var token: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.codepipeline.model.PutApprovalResultRequest) : this() {
this.actionName = x.actionName
this.pipelineName = x.pipelineName
this.result = x.result
this.stageName = x.stageName
this.token = x.token
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.codepipeline.model.PutApprovalResultRequest = PutApprovalResultRequest(this)
/**
* construct an [aws.sdk.kotlin.services.codepipeline.model.ApprovalResult] inside the given [block]
*/
public fun result(block: aws.sdk.kotlin.services.codepipeline.model.ApprovalResult.Builder.() -> kotlin.Unit) {
this.result = aws.sdk.kotlin.services.codepipeline.model.ApprovalResult.invoke(block)
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy