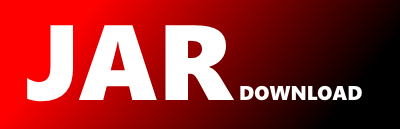
commonMain.aws.sdk.kotlin.services.codepipeline.model.ActionDeclaration.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of codepipeline Show documentation
Show all versions of codepipeline Show documentation
The AWS Kotlin client for CodePipeline
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.codepipeline.model
/**
* Represents information about an action declaration.
*/
public class ActionDeclaration private constructor(builder: Builder) {
/**
* Specifies the action type and the provider of the action.
*/
public val actionTypeId: aws.sdk.kotlin.services.codepipeline.model.ActionTypeId? = builder.actionTypeId
/**
* The action's configuration. These are key-value pairs that specify input values for an action. For more information, see [Action Structure Requirements in CodePipeline](https://docs.aws.amazon.com/codepipeline/latest/userguide/reference-pipeline-structure.html#action-requirements). For the list of configuration properties for the CloudFormation action type in CodePipeline, see [Configuration Properties Reference](https://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/continuous-delivery-codepipeline-action-reference.html) in the *CloudFormation User Guide*. For template snippets with examples, see [Using Parameter Override Functions with CodePipeline Pipelines](https://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/continuous-delivery-codepipeline-parameter-override-functions.html) in the *CloudFormation User Guide*.
*
* The values can be represented in either JSON or YAML format. For example, the JSON configuration item format is as follows:
*
* *JSON:*
*
* `"Configuration" : { Key : Value },`
*/
public val configuration: Map? = builder.configuration
/**
* The name or ID of the artifact consumed by the action, such as a test or build artifact.
*/
public val inputArtifacts: List? = builder.inputArtifacts
/**
* The action declaration's name.
*/
public val name: kotlin.String? = builder.name
/**
* The variable namespace associated with the action. All variables produced as output by this action fall under this namespace.
*/
public val namespace: kotlin.String? = builder.namespace
/**
* The name or ID of the result of the action declaration, such as a test or build artifact.
*/
public val outputArtifacts: List? = builder.outputArtifacts
/**
* The action declaration's Amazon Web Services Region, such as us-east-1.
*/
public val region: kotlin.String? = builder.region
/**
* The ARN of the IAM service role that performs the declared action. This is assumed through the roleArn for the pipeline.
*/
public val roleArn: kotlin.String? = builder.roleArn
/**
* The order in which actions are run.
*/
public val runOrder: kotlin.Int? = builder.runOrder
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.codepipeline.model.ActionDeclaration = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("ActionDeclaration(")
append("actionTypeId=$actionTypeId,")
append("configuration=$configuration,")
append("inputArtifacts=$inputArtifacts,")
append("name=$name,")
append("namespace=$namespace,")
append("outputArtifacts=$outputArtifacts,")
append("region=$region,")
append("roleArn=$roleArn,")
append("runOrder=$runOrder")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = actionTypeId?.hashCode() ?: 0
result = 31 * result + (configuration?.hashCode() ?: 0)
result = 31 * result + (inputArtifacts?.hashCode() ?: 0)
result = 31 * result + (name?.hashCode() ?: 0)
result = 31 * result + (namespace?.hashCode() ?: 0)
result = 31 * result + (outputArtifacts?.hashCode() ?: 0)
result = 31 * result + (region?.hashCode() ?: 0)
result = 31 * result + (roleArn?.hashCode() ?: 0)
result = 31 * result + (runOrder ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as ActionDeclaration
if (actionTypeId != other.actionTypeId) return false
if (configuration != other.configuration) return false
if (inputArtifacts != other.inputArtifacts) return false
if (name != other.name) return false
if (namespace != other.namespace) return false
if (outputArtifacts != other.outputArtifacts) return false
if (region != other.region) return false
if (roleArn != other.roleArn) return false
if (runOrder != other.runOrder) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.codepipeline.model.ActionDeclaration = Builder(this).apply(block).build()
public class Builder {
/**
* Specifies the action type and the provider of the action.
*/
public var actionTypeId: aws.sdk.kotlin.services.codepipeline.model.ActionTypeId? = null
/**
* The action's configuration. These are key-value pairs that specify input values for an action. For more information, see [Action Structure Requirements in CodePipeline](https://docs.aws.amazon.com/codepipeline/latest/userguide/reference-pipeline-structure.html#action-requirements). For the list of configuration properties for the CloudFormation action type in CodePipeline, see [Configuration Properties Reference](https://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/continuous-delivery-codepipeline-action-reference.html) in the *CloudFormation User Guide*. For template snippets with examples, see [Using Parameter Override Functions with CodePipeline Pipelines](https://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/continuous-delivery-codepipeline-parameter-override-functions.html) in the *CloudFormation User Guide*.
*
* The values can be represented in either JSON or YAML format. For example, the JSON configuration item format is as follows:
*
* *JSON:*
*
* `"Configuration" : { Key : Value },`
*/
public var configuration: Map? = null
/**
* The name or ID of the artifact consumed by the action, such as a test or build artifact.
*/
public var inputArtifacts: List? = null
/**
* The action declaration's name.
*/
public var name: kotlin.String? = null
/**
* The variable namespace associated with the action. All variables produced as output by this action fall under this namespace.
*/
public var namespace: kotlin.String? = null
/**
* The name or ID of the result of the action declaration, such as a test or build artifact.
*/
public var outputArtifacts: List? = null
/**
* The action declaration's Amazon Web Services Region, such as us-east-1.
*/
public var region: kotlin.String? = null
/**
* The ARN of the IAM service role that performs the declared action. This is assumed through the roleArn for the pipeline.
*/
public var roleArn: kotlin.String? = null
/**
* The order in which actions are run.
*/
public var runOrder: kotlin.Int? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.codepipeline.model.ActionDeclaration) : this() {
this.actionTypeId = x.actionTypeId
this.configuration = x.configuration
this.inputArtifacts = x.inputArtifacts
this.name = x.name
this.namespace = x.namespace
this.outputArtifacts = x.outputArtifacts
this.region = x.region
this.roleArn = x.roleArn
this.runOrder = x.runOrder
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.codepipeline.model.ActionDeclaration = ActionDeclaration(this)
/**
* construct an [aws.sdk.kotlin.services.codepipeline.model.ActionTypeId] inside the given [block]
*/
public fun actionTypeId(block: aws.sdk.kotlin.services.codepipeline.model.ActionTypeId.Builder.() -> kotlin.Unit) {
this.actionTypeId = aws.sdk.kotlin.services.codepipeline.model.ActionTypeId.invoke(block)
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy