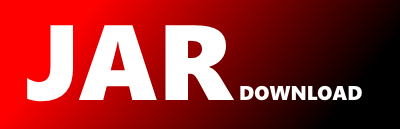
commonMain.aws.sdk.kotlin.services.codestarconnections.model.SyncConfiguration.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of codestarconnections-jvm Show documentation
Show all versions of codestarconnections-jvm Show documentation
The AWS SDK for Kotlin client for CodeStar connections
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.codestarconnections.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Information, such as repository, branch, provider, and resource names for a specific sync configuration.
*/
public class SyncConfiguration private constructor(builder: Builder) {
/**
* The branch associated with a specific sync configuration.
*/
public val branch: kotlin.String = requireNotNull(builder.branch) { "A non-null value must be provided for branch" }
/**
* The file path to the configuration file associated with a specific sync configuration. The path should point to an actual file in the sync configurations linked repository.
*/
public val configFile: kotlin.String? = builder.configFile
/**
* The owner ID for the repository associated with a specific sync configuration, such as the owner ID in GitHub.
*/
public val ownerId: kotlin.String = requireNotNull(builder.ownerId) { "A non-null value must be provided for ownerId" }
/**
* The connection provider type associated with a specific sync configuration, such as GitHub.
*/
public val providerType: aws.sdk.kotlin.services.codestarconnections.model.ProviderType = requireNotNull(builder.providerType) { "A non-null value must be provided for providerType" }
/**
* Whether to enable or disable publishing of deployment status to source providers.
*/
public val publishDeploymentStatus: aws.sdk.kotlin.services.codestarconnections.model.PublishDeploymentStatus? = builder.publishDeploymentStatus
/**
* The ID of the repository link associated with a specific sync configuration.
*/
public val repositoryLinkId: kotlin.String = requireNotNull(builder.repositoryLinkId) { "A non-null value must be provided for repositoryLinkId" }
/**
* The name of the repository associated with a specific sync configuration.
*/
public val repositoryName: kotlin.String = requireNotNull(builder.repositoryName) { "A non-null value must be provided for repositoryName" }
/**
* The name of the connection resource associated with a specific sync configuration.
*/
public val resourceName: kotlin.String = requireNotNull(builder.resourceName) { "A non-null value must be provided for resourceName" }
/**
* The Amazon Resource Name (ARN) of the IAM role associated with a specific sync configuration.
*/
public val roleArn: kotlin.String = requireNotNull(builder.roleArn) { "A non-null value must be provided for roleArn" }
/**
* The type of sync for a specific sync configuration.
*/
public val syncType: aws.sdk.kotlin.services.codestarconnections.model.SyncConfigurationType = requireNotNull(builder.syncType) { "A non-null value must be provided for syncType" }
/**
* When to trigger Git sync to begin the stack update.
*/
public val triggerResourceUpdateOn: aws.sdk.kotlin.services.codestarconnections.model.TriggerResourceUpdateOn? = builder.triggerResourceUpdateOn
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.codestarconnections.model.SyncConfiguration = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("SyncConfiguration(")
append("branch=$branch,")
append("configFile=$configFile,")
append("ownerId=$ownerId,")
append("providerType=$providerType,")
append("publishDeploymentStatus=$publishDeploymentStatus,")
append("repositoryLinkId=$repositoryLinkId,")
append("repositoryName=$repositoryName,")
append("resourceName=$resourceName,")
append("roleArn=$roleArn,")
append("syncType=$syncType,")
append("triggerResourceUpdateOn=$triggerResourceUpdateOn")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = branch.hashCode()
result = 31 * result + (configFile?.hashCode() ?: 0)
result = 31 * result + (ownerId.hashCode())
result = 31 * result + (providerType.hashCode())
result = 31 * result + (publishDeploymentStatus?.hashCode() ?: 0)
result = 31 * result + (repositoryLinkId.hashCode())
result = 31 * result + (repositoryName.hashCode())
result = 31 * result + (resourceName.hashCode())
result = 31 * result + (roleArn.hashCode())
result = 31 * result + (syncType.hashCode())
result = 31 * result + (triggerResourceUpdateOn?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as SyncConfiguration
if (branch != other.branch) return false
if (configFile != other.configFile) return false
if (ownerId != other.ownerId) return false
if (providerType != other.providerType) return false
if (publishDeploymentStatus != other.publishDeploymentStatus) return false
if (repositoryLinkId != other.repositoryLinkId) return false
if (repositoryName != other.repositoryName) return false
if (resourceName != other.resourceName) return false
if (roleArn != other.roleArn) return false
if (syncType != other.syncType) return false
if (triggerResourceUpdateOn != other.triggerResourceUpdateOn) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.codestarconnections.model.SyncConfiguration = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The branch associated with a specific sync configuration.
*/
public var branch: kotlin.String? = null
/**
* The file path to the configuration file associated with a specific sync configuration. The path should point to an actual file in the sync configurations linked repository.
*/
public var configFile: kotlin.String? = null
/**
* The owner ID for the repository associated with a specific sync configuration, such as the owner ID in GitHub.
*/
public var ownerId: kotlin.String? = null
/**
* The connection provider type associated with a specific sync configuration, such as GitHub.
*/
public var providerType: aws.sdk.kotlin.services.codestarconnections.model.ProviderType? = null
/**
* Whether to enable or disable publishing of deployment status to source providers.
*/
public var publishDeploymentStatus: aws.sdk.kotlin.services.codestarconnections.model.PublishDeploymentStatus? = null
/**
* The ID of the repository link associated with a specific sync configuration.
*/
public var repositoryLinkId: kotlin.String? = null
/**
* The name of the repository associated with a specific sync configuration.
*/
public var repositoryName: kotlin.String? = null
/**
* The name of the connection resource associated with a specific sync configuration.
*/
public var resourceName: kotlin.String? = null
/**
* The Amazon Resource Name (ARN) of the IAM role associated with a specific sync configuration.
*/
public var roleArn: kotlin.String? = null
/**
* The type of sync for a specific sync configuration.
*/
public var syncType: aws.sdk.kotlin.services.codestarconnections.model.SyncConfigurationType? = null
/**
* When to trigger Git sync to begin the stack update.
*/
public var triggerResourceUpdateOn: aws.sdk.kotlin.services.codestarconnections.model.TriggerResourceUpdateOn? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.codestarconnections.model.SyncConfiguration) : this() {
this.branch = x.branch
this.configFile = x.configFile
this.ownerId = x.ownerId
this.providerType = x.providerType
this.publishDeploymentStatus = x.publishDeploymentStatus
this.repositoryLinkId = x.repositoryLinkId
this.repositoryName = x.repositoryName
this.resourceName = x.resourceName
this.roleArn = x.roleArn
this.syncType = x.syncType
this.triggerResourceUpdateOn = x.triggerResourceUpdateOn
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.codestarconnections.model.SyncConfiguration = SyncConfiguration(this)
internal fun correctErrors(): Builder {
if (branch == null) branch = ""
if (ownerId == null) ownerId = ""
if (providerType == null) providerType = ProviderType.SdkUnknown("no value provided")
if (repositoryLinkId == null) repositoryLinkId = ""
if (repositoryName == null) repositoryName = ""
if (resourceName == null) resourceName = ""
if (roleArn == null) roleArn = ""
if (syncType == null) syncType = SyncConfigurationType.SdkUnknown("no value provided")
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy