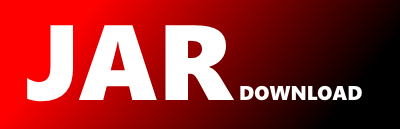
commonMain.aws.sdk.kotlin.services.codestarconnections.model.Connection.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of codestarconnections-jvm Show documentation
Show all versions of codestarconnections-jvm Show documentation
The AWS SDK for Kotlin client for CodeStar connections
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.codestarconnections.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* A resource that is used to connect third-party source providers with services like CodePipeline.
*
* Note: A connection created through CloudFormation, the CLI, or the SDK is in `PENDING` status by default. You can make its status `AVAILABLE` by updating the connection in the console.
*/
public class Connection private constructor(builder: Builder) {
/**
* The Amazon Resource Name (ARN) of the connection. The ARN is used as the connection reference when the connection is shared between Amazon Web Services.
*
* The ARN is never reused if the connection is deleted.
*/
public val connectionArn: kotlin.String? = builder.connectionArn
/**
* The name of the connection. Connection names must be unique in an Amazon Web Services account.
*/
public val connectionName: kotlin.String? = builder.connectionName
/**
* The current status of the connection.
*/
public val connectionStatus: aws.sdk.kotlin.services.codestarconnections.model.ConnectionStatus? = builder.connectionStatus
/**
* The Amazon Resource Name (ARN) of the host associated with the connection.
*/
public val hostArn: kotlin.String? = builder.hostArn
/**
* The identifier of the external provider where your third-party code repository is configured. For Bitbucket, this is the account ID of the owner of the Bitbucket repository.
*/
public val ownerAccountId: kotlin.String? = builder.ownerAccountId
/**
* The name of the external provider where your third-party code repository is configured.
*/
public val providerType: aws.sdk.kotlin.services.codestarconnections.model.ProviderType? = builder.providerType
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.codestarconnections.model.Connection = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("Connection(")
append("connectionArn=$connectionArn,")
append("connectionName=$connectionName,")
append("connectionStatus=$connectionStatus,")
append("hostArn=$hostArn,")
append("ownerAccountId=$ownerAccountId,")
append("providerType=$providerType")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = connectionArn?.hashCode() ?: 0
result = 31 * result + (connectionName?.hashCode() ?: 0)
result = 31 * result + (connectionStatus?.hashCode() ?: 0)
result = 31 * result + (hostArn?.hashCode() ?: 0)
result = 31 * result + (ownerAccountId?.hashCode() ?: 0)
result = 31 * result + (providerType?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as Connection
if (connectionArn != other.connectionArn) return false
if (connectionName != other.connectionName) return false
if (connectionStatus != other.connectionStatus) return false
if (hostArn != other.hostArn) return false
if (ownerAccountId != other.ownerAccountId) return false
if (providerType != other.providerType) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.codestarconnections.model.Connection = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The Amazon Resource Name (ARN) of the connection. The ARN is used as the connection reference when the connection is shared between Amazon Web Services.
*
* The ARN is never reused if the connection is deleted.
*/
public var connectionArn: kotlin.String? = null
/**
* The name of the connection. Connection names must be unique in an Amazon Web Services account.
*/
public var connectionName: kotlin.String? = null
/**
* The current status of the connection.
*/
public var connectionStatus: aws.sdk.kotlin.services.codestarconnections.model.ConnectionStatus? = null
/**
* The Amazon Resource Name (ARN) of the host associated with the connection.
*/
public var hostArn: kotlin.String? = null
/**
* The identifier of the external provider where your third-party code repository is configured. For Bitbucket, this is the account ID of the owner of the Bitbucket repository.
*/
public var ownerAccountId: kotlin.String? = null
/**
* The name of the external provider where your third-party code repository is configured.
*/
public var providerType: aws.sdk.kotlin.services.codestarconnections.model.ProviderType? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.codestarconnections.model.Connection) : this() {
this.connectionArn = x.connectionArn
this.connectionName = x.connectionName
this.connectionStatus = x.connectionStatus
this.hostArn = x.hostArn
this.ownerAccountId = x.ownerAccountId
this.providerType = x.providerType
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.codestarconnections.model.Connection = Connection(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy