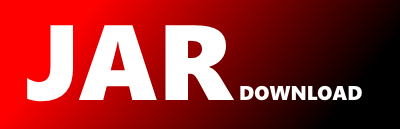
commonMain.aws.sdk.kotlin.services.codestarconnections.model.CreateHostRequest.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of codestarconnections-jvm Show documentation
Show all versions of codestarconnections-jvm Show documentation
The AWS SDK for Kotlin client for CodeStar connections
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.codestarconnections.model
import aws.smithy.kotlin.runtime.SdkDsl
public class CreateHostRequest private constructor(builder: Builder) {
/**
* The name of the host to be created.
*/
public val name: kotlin.String? = builder.name
/**
* The endpoint of the infrastructure to be represented by the host after it is created.
*/
public val providerEndpoint: kotlin.String? = builder.providerEndpoint
/**
* The name of the installed provider to be associated with your connection. The host resource represents the infrastructure where your provider type is installed. The valid provider type is GitHub Enterprise Server.
*/
public val providerType: aws.sdk.kotlin.services.codestarconnections.model.ProviderType? = builder.providerType
/**
* Tags for the host to be created.
*/
public val tags: List? = builder.tags
/**
* The VPC configuration to be provisioned for the host. A VPC must be configured and the infrastructure to be represented by the host must already be connected to the VPC.
*/
public val vpcConfiguration: aws.sdk.kotlin.services.codestarconnections.model.VpcConfiguration? = builder.vpcConfiguration
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.codestarconnections.model.CreateHostRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("CreateHostRequest(")
append("name=$name,")
append("providerEndpoint=$providerEndpoint,")
append("providerType=$providerType,")
append("tags=$tags,")
append("vpcConfiguration=$vpcConfiguration")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = name?.hashCode() ?: 0
result = 31 * result + (providerEndpoint?.hashCode() ?: 0)
result = 31 * result + (providerType?.hashCode() ?: 0)
result = 31 * result + (tags?.hashCode() ?: 0)
result = 31 * result + (vpcConfiguration?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as CreateHostRequest
if (name != other.name) return false
if (providerEndpoint != other.providerEndpoint) return false
if (providerType != other.providerType) return false
if (tags != other.tags) return false
if (vpcConfiguration != other.vpcConfiguration) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.codestarconnections.model.CreateHostRequest = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The name of the host to be created.
*/
public var name: kotlin.String? = null
/**
* The endpoint of the infrastructure to be represented by the host after it is created.
*/
public var providerEndpoint: kotlin.String? = null
/**
* The name of the installed provider to be associated with your connection. The host resource represents the infrastructure where your provider type is installed. The valid provider type is GitHub Enterprise Server.
*/
public var providerType: aws.sdk.kotlin.services.codestarconnections.model.ProviderType? = null
/**
* Tags for the host to be created.
*/
public var tags: List? = null
/**
* The VPC configuration to be provisioned for the host. A VPC must be configured and the infrastructure to be represented by the host must already be connected to the VPC.
*/
public var vpcConfiguration: aws.sdk.kotlin.services.codestarconnections.model.VpcConfiguration? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.codestarconnections.model.CreateHostRequest) : this() {
this.name = x.name
this.providerEndpoint = x.providerEndpoint
this.providerType = x.providerType
this.tags = x.tags
this.vpcConfiguration = x.vpcConfiguration
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.codestarconnections.model.CreateHostRequest = CreateHostRequest(this)
/**
* construct an [aws.sdk.kotlin.services.codestarconnections.model.VpcConfiguration] inside the given [block]
*/
public fun vpcConfiguration(block: aws.sdk.kotlin.services.codestarconnections.model.VpcConfiguration.Builder.() -> kotlin.Unit) {
this.vpcConfiguration = aws.sdk.kotlin.services.codestarconnections.model.VpcConfiguration.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy