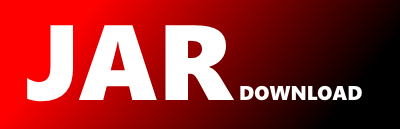
commonMain.aws.sdk.kotlin.services.configservice.model.AggregateEvaluationResult.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of configservice-jvm Show documentation
Show all versions of configservice-jvm Show documentation
The AWS Kotlin client for Config Service
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.configservice.model
import aws.smithy.kotlin.runtime.time.Instant
/**
* The details of an Config evaluation for an account ID and region in an aggregator. Provides the Amazon Web Services resource that was evaluated, the compliance of the resource, related time stamps, and supplementary information.
*/
public class AggregateEvaluationResult private constructor(builder: Builder) {
/**
* The 12-digit account ID of the source account.
*/
public val accountId: kotlin.String? = builder.accountId
/**
* Supplementary information about how the agrregate evaluation determined the compliance.
*/
public val annotation: kotlin.String? = builder.annotation
/**
* The source region from where the data is aggregated.
*/
public val awsRegion: kotlin.String? = builder.awsRegion
/**
* The resource compliance status.
*
* For the `AggregationEvaluationResult` data type, Config supports only the `COMPLIANT` and `NON_COMPLIANT`. Config does not support the `NOT_APPLICABLE` and `INSUFFICIENT_DATA` value.
*/
public val complianceType: aws.sdk.kotlin.services.configservice.model.ComplianceType? = builder.complianceType
/**
* The time when the Config rule evaluated the Amazon Web Services resource.
*/
public val configRuleInvokedTime: aws.smithy.kotlin.runtime.time.Instant? = builder.configRuleInvokedTime
/**
* Uniquely identifies the evaluation result.
*/
public val evaluationResultIdentifier: aws.sdk.kotlin.services.configservice.model.EvaluationResultIdentifier? = builder.evaluationResultIdentifier
/**
* The time when Config recorded the aggregate evaluation result.
*/
public val resultRecordedTime: aws.smithy.kotlin.runtime.time.Instant? = builder.resultRecordedTime
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.configservice.model.AggregateEvaluationResult = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("AggregateEvaluationResult(")
append("accountId=$accountId,")
append("annotation=$annotation,")
append("awsRegion=$awsRegion,")
append("complianceType=$complianceType,")
append("configRuleInvokedTime=$configRuleInvokedTime,")
append("evaluationResultIdentifier=$evaluationResultIdentifier,")
append("resultRecordedTime=$resultRecordedTime")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = accountId?.hashCode() ?: 0
result = 31 * result + (annotation?.hashCode() ?: 0)
result = 31 * result + (awsRegion?.hashCode() ?: 0)
result = 31 * result + (complianceType?.hashCode() ?: 0)
result = 31 * result + (configRuleInvokedTime?.hashCode() ?: 0)
result = 31 * result + (evaluationResultIdentifier?.hashCode() ?: 0)
result = 31 * result + (resultRecordedTime?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as AggregateEvaluationResult
if (accountId != other.accountId) return false
if (annotation != other.annotation) return false
if (awsRegion != other.awsRegion) return false
if (complianceType != other.complianceType) return false
if (configRuleInvokedTime != other.configRuleInvokedTime) return false
if (evaluationResultIdentifier != other.evaluationResultIdentifier) return false
if (resultRecordedTime != other.resultRecordedTime) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.configservice.model.AggregateEvaluationResult = Builder(this).apply(block).build()
public class Builder {
/**
* The 12-digit account ID of the source account.
*/
public var accountId: kotlin.String? = null
/**
* Supplementary information about how the agrregate evaluation determined the compliance.
*/
public var annotation: kotlin.String? = null
/**
* The source region from where the data is aggregated.
*/
public var awsRegion: kotlin.String? = null
/**
* The resource compliance status.
*
* For the `AggregationEvaluationResult` data type, Config supports only the `COMPLIANT` and `NON_COMPLIANT`. Config does not support the `NOT_APPLICABLE` and `INSUFFICIENT_DATA` value.
*/
public var complianceType: aws.sdk.kotlin.services.configservice.model.ComplianceType? = null
/**
* The time when the Config rule evaluated the Amazon Web Services resource.
*/
public var configRuleInvokedTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* Uniquely identifies the evaluation result.
*/
public var evaluationResultIdentifier: aws.sdk.kotlin.services.configservice.model.EvaluationResultIdentifier? = null
/**
* The time when Config recorded the aggregate evaluation result.
*/
public var resultRecordedTime: aws.smithy.kotlin.runtime.time.Instant? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.configservice.model.AggregateEvaluationResult) : this() {
this.accountId = x.accountId
this.annotation = x.annotation
this.awsRegion = x.awsRegion
this.complianceType = x.complianceType
this.configRuleInvokedTime = x.configRuleInvokedTime
this.evaluationResultIdentifier = x.evaluationResultIdentifier
this.resultRecordedTime = x.resultRecordedTime
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.configservice.model.AggregateEvaluationResult = AggregateEvaluationResult(this)
/**
* construct an [aws.sdk.kotlin.services.configservice.model.EvaluationResultIdentifier] inside the given [block]
*/
public fun evaluationResultIdentifier(block: aws.sdk.kotlin.services.configservice.model.EvaluationResultIdentifier.Builder.() -> kotlin.Unit) {
this.evaluationResultIdentifier = aws.sdk.kotlin.services.configservice.model.EvaluationResultIdentifier.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy