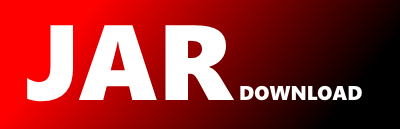
commonMain.aws.sdk.kotlin.services.configservice.model.ConfigExportDeliveryInfo.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of configservice-jvm Show documentation
Show all versions of configservice-jvm Show documentation
The AWS Kotlin client for Config Service
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.configservice.model
import aws.smithy.kotlin.runtime.time.Instant
/**
* Provides status of the delivery of the snapshot or the configuration history to the specified Amazon S3 bucket. Also provides the status of notifications about the Amazon S3 delivery to the specified Amazon SNS topic.
*/
public class ConfigExportDeliveryInfo private constructor(builder: Builder) {
/**
* The time of the last attempted delivery.
*/
public val lastAttemptTime: aws.smithy.kotlin.runtime.time.Instant? = builder.lastAttemptTime
/**
* The error code from the last attempted delivery.
*/
public val lastErrorCode: kotlin.String? = builder.lastErrorCode
/**
* The error message from the last attempted delivery.
*/
public val lastErrorMessage: kotlin.String? = builder.lastErrorMessage
/**
* Status of the last attempted delivery.
*/
public val lastStatus: aws.sdk.kotlin.services.configservice.model.DeliveryStatus? = builder.lastStatus
/**
* The time of the last successful delivery.
*/
public val lastSuccessfulTime: aws.smithy.kotlin.runtime.time.Instant? = builder.lastSuccessfulTime
/**
* The time that the next delivery occurs.
*/
public val nextDeliveryTime: aws.smithy.kotlin.runtime.time.Instant? = builder.nextDeliveryTime
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.configservice.model.ConfigExportDeliveryInfo = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("ConfigExportDeliveryInfo(")
append("lastAttemptTime=$lastAttemptTime,")
append("lastErrorCode=$lastErrorCode,")
append("lastErrorMessage=$lastErrorMessage,")
append("lastStatus=$lastStatus,")
append("lastSuccessfulTime=$lastSuccessfulTime,")
append("nextDeliveryTime=$nextDeliveryTime")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = lastAttemptTime?.hashCode() ?: 0
result = 31 * result + (lastErrorCode?.hashCode() ?: 0)
result = 31 * result + (lastErrorMessage?.hashCode() ?: 0)
result = 31 * result + (lastStatus?.hashCode() ?: 0)
result = 31 * result + (lastSuccessfulTime?.hashCode() ?: 0)
result = 31 * result + (nextDeliveryTime?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as ConfigExportDeliveryInfo
if (lastAttemptTime != other.lastAttemptTime) return false
if (lastErrorCode != other.lastErrorCode) return false
if (lastErrorMessage != other.lastErrorMessage) return false
if (lastStatus != other.lastStatus) return false
if (lastSuccessfulTime != other.lastSuccessfulTime) return false
if (nextDeliveryTime != other.nextDeliveryTime) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.configservice.model.ConfigExportDeliveryInfo = Builder(this).apply(block).build()
public class Builder {
/**
* The time of the last attempted delivery.
*/
public var lastAttemptTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The error code from the last attempted delivery.
*/
public var lastErrorCode: kotlin.String? = null
/**
* The error message from the last attempted delivery.
*/
public var lastErrorMessage: kotlin.String? = null
/**
* Status of the last attempted delivery.
*/
public var lastStatus: aws.sdk.kotlin.services.configservice.model.DeliveryStatus? = null
/**
* The time of the last successful delivery.
*/
public var lastSuccessfulTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The time that the next delivery occurs.
*/
public var nextDeliveryTime: aws.smithy.kotlin.runtime.time.Instant? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.configservice.model.ConfigExportDeliveryInfo) : this() {
this.lastAttemptTime = x.lastAttemptTime
this.lastErrorCode = x.lastErrorCode
this.lastErrorMessage = x.lastErrorMessage
this.lastStatus = x.lastStatus
this.lastSuccessfulTime = x.lastSuccessfulTime
this.nextDeliveryTime = x.nextDeliveryTime
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.configservice.model.ConfigExportDeliveryInfo = ConfigExportDeliveryInfo(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy