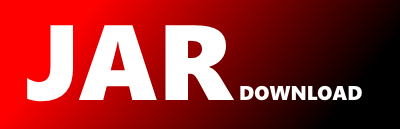
commonMain.aws.sdk.kotlin.services.configservice.model.ConfigurationItem.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.configservice.model
import aws.smithy.kotlin.runtime.time.Instant
/**
* A list that contains detailed configurations of a specified resource.
*/
public class ConfigurationItem private constructor(builder: Builder) {
/**
* The 12-digit Amazon Web Services account ID associated with the resource.
*/
public val accountId: kotlin.String? = builder.accountId
/**
* Amazon Resource Name (ARN) associated with the resource.
*/
public val arn: kotlin.String? = builder.arn
/**
* The Availability Zone associated with the resource.
*/
public val availabilityZone: kotlin.String? = builder.availabilityZone
/**
* The region where the resource resides.
*/
public val awsRegion: kotlin.String? = builder.awsRegion
/**
* The description of the resource configuration.
*/
public val configuration: kotlin.String? = builder.configuration
/**
* The time when the configuration recording was initiated.
*/
public val configurationItemCaptureTime: aws.smithy.kotlin.runtime.time.Instant? = builder.configurationItemCaptureTime
/**
* Unique MD5 hash that represents the configuration item's state.
*
* You can use MD5 hash to compare the states of two or more configuration items that are associated with the same resource.
*/
public val configurationItemMd5Hash: kotlin.String? = builder.configurationItemMd5Hash
/**
* The configuration item status. The valid values are:
* + OK – The resource configuration has been updated
* + ResourceDiscovered – The resource was newly discovered
* + ResourceNotRecorded – The resource was discovered but its configuration was not recorded since the recorder excludes the recording of resources of this type
* + ResourceDeleted – The resource was deleted
* + ResourceDeletedNotRecorded – The resource was deleted but its configuration was not recorded since the recorder excludes the recording of resources of this type
*/
public val configurationItemStatus: aws.sdk.kotlin.services.configservice.model.ConfigurationItemStatus? = builder.configurationItemStatus
/**
* An identifier that indicates the ordering of the configuration items of a resource.
*/
public val configurationStateId: kotlin.String? = builder.configurationStateId
/**
* A list of CloudTrail event IDs.
*
* A populated field indicates that the current configuration was initiated by the events recorded in the CloudTrail log. For more information about CloudTrail, see [What Is CloudTrail](https://docs.aws.amazon.com/awscloudtrail/latest/userguide/what_is_cloud_trail_top_level.html).
*
* An empty field indicates that the current configuration was not initiated by any event. As of Version 1.3, the relatedEvents field is empty. You can access the [LookupEvents API](https://docs.aws.amazon.com/awscloudtrail/latest/APIReference/API_LookupEvents.html) in the *CloudTrail API Reference* to retrieve the events for the resource.
*/
public val relatedEvents: List? = builder.relatedEvents
/**
* A list of related Amazon Web Services resources.
*/
public val relationships: List? = builder.relationships
/**
* The time stamp when the resource was created.
*/
public val resourceCreationTime: aws.smithy.kotlin.runtime.time.Instant? = builder.resourceCreationTime
/**
* The ID of the resource (for example, `sg-xxxxxx`).
*/
public val resourceId: kotlin.String? = builder.resourceId
/**
* The custom name of the resource, if available.
*/
public val resourceName: kotlin.String? = builder.resourceName
/**
* The type of Amazon Web Services resource.
*/
public val resourceType: aws.sdk.kotlin.services.configservice.model.ResourceType? = builder.resourceType
/**
* Configuration attributes that Config returns for certain resource types to supplement the information returned for the `configuration` parameter.
*/
public val supplementaryConfiguration: Map? = builder.supplementaryConfiguration
/**
* A mapping of key value tags associated with the resource.
*/
public val tags: Map? = builder.tags
/**
* The version number of the resource configuration.
*/
public val version: kotlin.String? = builder.version
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.configservice.model.ConfigurationItem = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("ConfigurationItem(")
append("accountId=$accountId,")
append("arn=$arn,")
append("availabilityZone=$availabilityZone,")
append("awsRegion=$awsRegion,")
append("configuration=$configuration,")
append("configurationItemCaptureTime=$configurationItemCaptureTime,")
append("configurationItemMd5Hash=$configurationItemMd5Hash,")
append("configurationItemStatus=$configurationItemStatus,")
append("configurationStateId=$configurationStateId,")
append("relatedEvents=$relatedEvents,")
append("relationships=$relationships,")
append("resourceCreationTime=$resourceCreationTime,")
append("resourceId=$resourceId,")
append("resourceName=$resourceName,")
append("resourceType=$resourceType,")
append("supplementaryConfiguration=$supplementaryConfiguration,")
append("tags=$tags,")
append("version=$version")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = accountId?.hashCode() ?: 0
result = 31 * result + (arn?.hashCode() ?: 0)
result = 31 * result + (availabilityZone?.hashCode() ?: 0)
result = 31 * result + (awsRegion?.hashCode() ?: 0)
result = 31 * result + (configuration?.hashCode() ?: 0)
result = 31 * result + (configurationItemCaptureTime?.hashCode() ?: 0)
result = 31 * result + (configurationItemMd5Hash?.hashCode() ?: 0)
result = 31 * result + (configurationItemStatus?.hashCode() ?: 0)
result = 31 * result + (configurationStateId?.hashCode() ?: 0)
result = 31 * result + (relatedEvents?.hashCode() ?: 0)
result = 31 * result + (relationships?.hashCode() ?: 0)
result = 31 * result + (resourceCreationTime?.hashCode() ?: 0)
result = 31 * result + (resourceId?.hashCode() ?: 0)
result = 31 * result + (resourceName?.hashCode() ?: 0)
result = 31 * result + (resourceType?.hashCode() ?: 0)
result = 31 * result + (supplementaryConfiguration?.hashCode() ?: 0)
result = 31 * result + (tags?.hashCode() ?: 0)
result = 31 * result + (version?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as ConfigurationItem
if (accountId != other.accountId) return false
if (arn != other.arn) return false
if (availabilityZone != other.availabilityZone) return false
if (awsRegion != other.awsRegion) return false
if (configuration != other.configuration) return false
if (configurationItemCaptureTime != other.configurationItemCaptureTime) return false
if (configurationItemMd5Hash != other.configurationItemMd5Hash) return false
if (configurationItemStatus != other.configurationItemStatus) return false
if (configurationStateId != other.configurationStateId) return false
if (relatedEvents != other.relatedEvents) return false
if (relationships != other.relationships) return false
if (resourceCreationTime != other.resourceCreationTime) return false
if (resourceId != other.resourceId) return false
if (resourceName != other.resourceName) return false
if (resourceType != other.resourceType) return false
if (supplementaryConfiguration != other.supplementaryConfiguration) return false
if (tags != other.tags) return false
if (version != other.version) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.configservice.model.ConfigurationItem = Builder(this).apply(block).build()
public class Builder {
/**
* The 12-digit Amazon Web Services account ID associated with the resource.
*/
public var accountId: kotlin.String? = null
/**
* Amazon Resource Name (ARN) associated with the resource.
*/
public var arn: kotlin.String? = null
/**
* The Availability Zone associated with the resource.
*/
public var availabilityZone: kotlin.String? = null
/**
* The region where the resource resides.
*/
public var awsRegion: kotlin.String? = null
/**
* The description of the resource configuration.
*/
public var configuration: kotlin.String? = null
/**
* The time when the configuration recording was initiated.
*/
public var configurationItemCaptureTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* Unique MD5 hash that represents the configuration item's state.
*
* You can use MD5 hash to compare the states of two or more configuration items that are associated with the same resource.
*/
public var configurationItemMd5Hash: kotlin.String? = null
/**
* The configuration item status. The valid values are:
* + OK – The resource configuration has been updated
* + ResourceDiscovered – The resource was newly discovered
* + ResourceNotRecorded – The resource was discovered but its configuration was not recorded since the recorder excludes the recording of resources of this type
* + ResourceDeleted – The resource was deleted
* + ResourceDeletedNotRecorded – The resource was deleted but its configuration was not recorded since the recorder excludes the recording of resources of this type
*/
public var configurationItemStatus: aws.sdk.kotlin.services.configservice.model.ConfigurationItemStatus? = null
/**
* An identifier that indicates the ordering of the configuration items of a resource.
*/
public var configurationStateId: kotlin.String? = null
/**
* A list of CloudTrail event IDs.
*
* A populated field indicates that the current configuration was initiated by the events recorded in the CloudTrail log. For more information about CloudTrail, see [What Is CloudTrail](https://docs.aws.amazon.com/awscloudtrail/latest/userguide/what_is_cloud_trail_top_level.html).
*
* An empty field indicates that the current configuration was not initiated by any event. As of Version 1.3, the relatedEvents field is empty. You can access the [LookupEvents API](https://docs.aws.amazon.com/awscloudtrail/latest/APIReference/API_LookupEvents.html) in the *CloudTrail API Reference* to retrieve the events for the resource.
*/
public var relatedEvents: List? = null
/**
* A list of related Amazon Web Services resources.
*/
public var relationships: List? = null
/**
* The time stamp when the resource was created.
*/
public var resourceCreationTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The ID of the resource (for example, `sg-xxxxxx`).
*/
public var resourceId: kotlin.String? = null
/**
* The custom name of the resource, if available.
*/
public var resourceName: kotlin.String? = null
/**
* The type of Amazon Web Services resource.
*/
public var resourceType: aws.sdk.kotlin.services.configservice.model.ResourceType? = null
/**
* Configuration attributes that Config returns for certain resource types to supplement the information returned for the `configuration` parameter.
*/
public var supplementaryConfiguration: Map? = null
/**
* A mapping of key value tags associated with the resource.
*/
public var tags: Map? = null
/**
* The version number of the resource configuration.
*/
public var version: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.configservice.model.ConfigurationItem) : this() {
this.accountId = x.accountId
this.arn = x.arn
this.availabilityZone = x.availabilityZone
this.awsRegion = x.awsRegion
this.configuration = x.configuration
this.configurationItemCaptureTime = x.configurationItemCaptureTime
this.configurationItemMd5Hash = x.configurationItemMd5Hash
this.configurationItemStatus = x.configurationItemStatus
this.configurationStateId = x.configurationStateId
this.relatedEvents = x.relatedEvents
this.relationships = x.relationships
this.resourceCreationTime = x.resourceCreationTime
this.resourceId = x.resourceId
this.resourceName = x.resourceName
this.resourceType = x.resourceType
this.supplementaryConfiguration = x.supplementaryConfiguration
this.tags = x.tags
this.version = x.version
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.configservice.model.ConfigurationItem = ConfigurationItem(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy