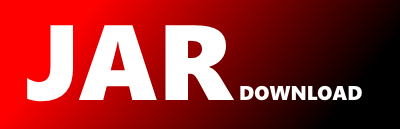
commonMain.aws.sdk.kotlin.services.configservice.model.ConformancePackStatusDetail.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of configservice-jvm Show documentation
Show all versions of configservice-jvm Show documentation
The AWS Kotlin client for Config Service
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.configservice.model
import aws.smithy.kotlin.runtime.time.Instant
/**
* Status details of a conformance pack.
*/
public class ConformancePackStatusDetail private constructor(builder: Builder) {
/**
* Amazon Resource Name (ARN) of comformance pack.
*/
public val conformancePackArn: kotlin.String = requireNotNull(builder.conformancePackArn) { "A non-null value must be provided for conformancePackArn" }
/**
* ID of the conformance pack.
*/
public val conformancePackId: kotlin.String = requireNotNull(builder.conformancePackId) { "A non-null value must be provided for conformancePackId" }
/**
* Name of the conformance pack.
*/
public val conformancePackName: kotlin.String = requireNotNull(builder.conformancePackName) { "A non-null value must be provided for conformancePackName" }
/**
* Indicates deployment status of conformance pack.
*
* Config sets the state of the conformance pack to:
* + CREATE_IN_PROGRESS when a conformance pack creation is in progress for an account.
* + CREATE_COMPLETE when a conformance pack has been successfully created in your account.
* + CREATE_FAILED when a conformance pack creation failed in your account.
* + DELETE_IN_PROGRESS when a conformance pack deletion is in progress.
* + DELETE_FAILED when a conformance pack deletion failed in your account.
*/
public val conformancePackState: aws.sdk.kotlin.services.configservice.model.ConformancePackState = requireNotNull(builder.conformancePackState) { "A non-null value must be provided for conformancePackState" }
/**
* The reason of conformance pack creation failure.
*/
public val conformancePackStatusReason: kotlin.String? = builder.conformancePackStatusReason
/**
* Last time when conformation pack creation and update was successful.
*/
public val lastUpdateCompletedTime: aws.smithy.kotlin.runtime.time.Instant? = builder.lastUpdateCompletedTime
/**
* Last time when conformation pack creation and update was requested.
*/
public val lastUpdateRequestedTime: aws.smithy.kotlin.runtime.time.Instant = requireNotNull(builder.lastUpdateRequestedTime) { "A non-null value must be provided for lastUpdateRequestedTime" }
/**
* Amazon Resource Name (ARN) of CloudFormation stack.
*/
public val stackArn: kotlin.String = requireNotNull(builder.stackArn) { "A non-null value must be provided for stackArn" }
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.configservice.model.ConformancePackStatusDetail = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("ConformancePackStatusDetail(")
append("conformancePackArn=$conformancePackArn,")
append("conformancePackId=$conformancePackId,")
append("conformancePackName=$conformancePackName,")
append("conformancePackState=$conformancePackState,")
append("conformancePackStatusReason=$conformancePackStatusReason,")
append("lastUpdateCompletedTime=$lastUpdateCompletedTime,")
append("lastUpdateRequestedTime=$lastUpdateRequestedTime,")
append("stackArn=$stackArn")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = conformancePackArn.hashCode()
result = 31 * result + (conformancePackId.hashCode())
result = 31 * result + (conformancePackName.hashCode())
result = 31 * result + (conformancePackState.hashCode())
result = 31 * result + (conformancePackStatusReason?.hashCode() ?: 0)
result = 31 * result + (lastUpdateCompletedTime?.hashCode() ?: 0)
result = 31 * result + (lastUpdateRequestedTime.hashCode())
result = 31 * result + (stackArn.hashCode())
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as ConformancePackStatusDetail
if (conformancePackArn != other.conformancePackArn) return false
if (conformancePackId != other.conformancePackId) return false
if (conformancePackName != other.conformancePackName) return false
if (conformancePackState != other.conformancePackState) return false
if (conformancePackStatusReason != other.conformancePackStatusReason) return false
if (lastUpdateCompletedTime != other.lastUpdateCompletedTime) return false
if (lastUpdateRequestedTime != other.lastUpdateRequestedTime) return false
if (stackArn != other.stackArn) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.configservice.model.ConformancePackStatusDetail = Builder(this).apply(block).build()
public class Builder {
/**
* Amazon Resource Name (ARN) of comformance pack.
*/
public var conformancePackArn: kotlin.String? = null
/**
* ID of the conformance pack.
*/
public var conformancePackId: kotlin.String? = null
/**
* Name of the conformance pack.
*/
public var conformancePackName: kotlin.String? = null
/**
* Indicates deployment status of conformance pack.
*
* Config sets the state of the conformance pack to:
* + CREATE_IN_PROGRESS when a conformance pack creation is in progress for an account.
* + CREATE_COMPLETE when a conformance pack has been successfully created in your account.
* + CREATE_FAILED when a conformance pack creation failed in your account.
* + DELETE_IN_PROGRESS when a conformance pack deletion is in progress.
* + DELETE_FAILED when a conformance pack deletion failed in your account.
*/
public var conformancePackState: aws.sdk.kotlin.services.configservice.model.ConformancePackState? = null
/**
* The reason of conformance pack creation failure.
*/
public var conformancePackStatusReason: kotlin.String? = null
/**
* Last time when conformation pack creation and update was successful.
*/
public var lastUpdateCompletedTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* Last time when conformation pack creation and update was requested.
*/
public var lastUpdateRequestedTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* Amazon Resource Name (ARN) of CloudFormation stack.
*/
public var stackArn: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.configservice.model.ConformancePackStatusDetail) : this() {
this.conformancePackArn = x.conformancePackArn
this.conformancePackId = x.conformancePackId
this.conformancePackName = x.conformancePackName
this.conformancePackState = x.conformancePackState
this.conformancePackStatusReason = x.conformancePackStatusReason
this.lastUpdateCompletedTime = x.lastUpdateCompletedTime
this.lastUpdateRequestedTime = x.lastUpdateRequestedTime
this.stackArn = x.stackArn
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.configservice.model.ConformancePackStatusDetail = ConformancePackStatusDetail(this)
internal fun correctErrors(): Builder {
if (conformancePackArn == null) conformancePackArn = ""
if (conformancePackId == null) conformancePackId = ""
if (conformancePackName == null) conformancePackName = ""
if (conformancePackState == null) conformancePackState = ConformancePackState.SdkUnknown("no value provided")
if (lastUpdateRequestedTime == null) lastUpdateRequestedTime = Instant.fromEpochSeconds(0)
if (stackArn == null) stackArn = ""
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy