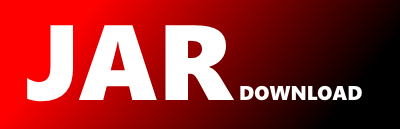
commonMain.aws.sdk.kotlin.services.configservice.model.DeliveryChannel.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of configservice-jvm Show documentation
Show all versions of configservice-jvm Show documentation
The AWS Kotlin client for Config Service
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.configservice.model
/**
* The channel through which Config delivers notifications and updated configuration states.
*/
public class DeliveryChannel private constructor(builder: Builder) {
/**
* The options for how often Config delivers configuration snapshots to the Amazon S3 bucket.
*/
public val configSnapshotDeliveryProperties: aws.sdk.kotlin.services.configservice.model.ConfigSnapshotDeliveryProperties? = builder.configSnapshotDeliveryProperties
/**
* The name of the delivery channel. By default, Config assigns the name "default" when creating the delivery channel. To change the delivery channel name, you must use the DeleteDeliveryChannel action to delete your current delivery channel, and then you must use the PutDeliveryChannel command to create a delivery channel that has the desired name.
*/
public val name: kotlin.String? = builder.name
/**
* The name of the Amazon S3 bucket to which Config delivers configuration snapshots and configuration history files.
*
* If you specify a bucket that belongs to another Amazon Web Services account, that bucket must have policies that grant access permissions to Config. For more information, see [Permissions for the Amazon S3 Bucket](https://docs.aws.amazon.com/config/latest/developerguide/s3-bucket-policy.html) in the *Config Developer Guide*.
*/
public val s3BucketName: kotlin.String? = builder.s3BucketName
/**
* The prefix for the specified Amazon S3 bucket.
*/
public val s3KeyPrefix: kotlin.String? = builder.s3KeyPrefix
/**
* The Amazon Resource Name (ARN) of the Key Management Service (KMS ) KMS key (KMS key) used to encrypt objects delivered by Config. Must belong to the same Region as the destination S3 bucket.
*/
public val s3KmsKeyArn: kotlin.String? = builder.s3KmsKeyArn
/**
* The Amazon Resource Name (ARN) of the Amazon SNS topic to which Config sends notifications about configuration changes.
*
* If you choose a topic from another account, the topic must have policies that grant access permissions to Config. For more information, see [Permissions for the Amazon SNS Topic](https://docs.aws.amazon.com/config/latest/developerguide/sns-topic-policy.html) in the *Config Developer Guide*.
*/
public val snsTopicArn: kotlin.String? = builder.snsTopicArn
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.configservice.model.DeliveryChannel = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("DeliveryChannel(")
append("configSnapshotDeliveryProperties=$configSnapshotDeliveryProperties,")
append("name=$name,")
append("s3BucketName=$s3BucketName,")
append("s3KeyPrefix=$s3KeyPrefix,")
append("s3KmsKeyArn=$s3KmsKeyArn,")
append("snsTopicArn=$snsTopicArn")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = configSnapshotDeliveryProperties?.hashCode() ?: 0
result = 31 * result + (name?.hashCode() ?: 0)
result = 31 * result + (s3BucketName?.hashCode() ?: 0)
result = 31 * result + (s3KeyPrefix?.hashCode() ?: 0)
result = 31 * result + (s3KmsKeyArn?.hashCode() ?: 0)
result = 31 * result + (snsTopicArn?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as DeliveryChannel
if (configSnapshotDeliveryProperties != other.configSnapshotDeliveryProperties) return false
if (name != other.name) return false
if (s3BucketName != other.s3BucketName) return false
if (s3KeyPrefix != other.s3KeyPrefix) return false
if (s3KmsKeyArn != other.s3KmsKeyArn) return false
if (snsTopicArn != other.snsTopicArn) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.configservice.model.DeliveryChannel = Builder(this).apply(block).build()
public class Builder {
/**
* The options for how often Config delivers configuration snapshots to the Amazon S3 bucket.
*/
public var configSnapshotDeliveryProperties: aws.sdk.kotlin.services.configservice.model.ConfigSnapshotDeliveryProperties? = null
/**
* The name of the delivery channel. By default, Config assigns the name "default" when creating the delivery channel. To change the delivery channel name, you must use the DeleteDeliveryChannel action to delete your current delivery channel, and then you must use the PutDeliveryChannel command to create a delivery channel that has the desired name.
*/
public var name: kotlin.String? = null
/**
* The name of the Amazon S3 bucket to which Config delivers configuration snapshots and configuration history files.
*
* If you specify a bucket that belongs to another Amazon Web Services account, that bucket must have policies that grant access permissions to Config. For more information, see [Permissions for the Amazon S3 Bucket](https://docs.aws.amazon.com/config/latest/developerguide/s3-bucket-policy.html) in the *Config Developer Guide*.
*/
public var s3BucketName: kotlin.String? = null
/**
* The prefix for the specified Amazon S3 bucket.
*/
public var s3KeyPrefix: kotlin.String? = null
/**
* The Amazon Resource Name (ARN) of the Key Management Service (KMS ) KMS key (KMS key) used to encrypt objects delivered by Config. Must belong to the same Region as the destination S3 bucket.
*/
public var s3KmsKeyArn: kotlin.String? = null
/**
* The Amazon Resource Name (ARN) of the Amazon SNS topic to which Config sends notifications about configuration changes.
*
* If you choose a topic from another account, the topic must have policies that grant access permissions to Config. For more information, see [Permissions for the Amazon SNS Topic](https://docs.aws.amazon.com/config/latest/developerguide/sns-topic-policy.html) in the *Config Developer Guide*.
*/
public var snsTopicArn: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.configservice.model.DeliveryChannel) : this() {
this.configSnapshotDeliveryProperties = x.configSnapshotDeliveryProperties
this.name = x.name
this.s3BucketName = x.s3BucketName
this.s3KeyPrefix = x.s3KeyPrefix
this.s3KmsKeyArn = x.s3KmsKeyArn
this.snsTopicArn = x.snsTopicArn
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.configservice.model.DeliveryChannel = DeliveryChannel(this)
/**
* construct an [aws.sdk.kotlin.services.configservice.model.ConfigSnapshotDeliveryProperties] inside the given [block]
*/
public fun configSnapshotDeliveryProperties(block: aws.sdk.kotlin.services.configservice.model.ConfigSnapshotDeliveryProperties.Builder.() -> kotlin.Unit) {
this.configSnapshotDeliveryProperties = aws.sdk.kotlin.services.configservice.model.ConfigSnapshotDeliveryProperties.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy