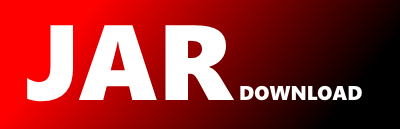
commonMain.aws.sdk.kotlin.services.configservice.model.Evaluation.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of configservice-jvm Show documentation
Show all versions of configservice-jvm Show documentation
The AWS Kotlin client for Config Service
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.configservice.model
import aws.smithy.kotlin.runtime.time.Instant
/**
* Identifies an Amazon Web Services resource and indicates whether it complies with the Config rule that it was evaluated against.
*/
public class Evaluation private constructor(builder: Builder) {
/**
* Supplementary information about how the evaluation determined the compliance.
*/
public val annotation: kotlin.String? = builder.annotation
/**
* The ID of the Amazon Web Services resource that was evaluated.
*/
public val complianceResourceId: kotlin.String = requireNotNull(builder.complianceResourceId) { "A non-null value must be provided for complianceResourceId" }
/**
* The type of Amazon Web Services resource that was evaluated.
*/
public val complianceResourceType: kotlin.String = requireNotNull(builder.complianceResourceType) { "A non-null value must be provided for complianceResourceType" }
/**
* Indicates whether the Amazon Web Services resource complies with the Config rule that it was evaluated against.
*
* For the `Evaluation` data type, Config supports only the `COMPLIANT`, `NON_COMPLIANT`, and `NOT_APPLICABLE` values. Config does not support the `INSUFFICIENT_DATA` value for this data type.
*
* Similarly, Config does not accept `INSUFFICIENT_DATA` as the value for `ComplianceType` from a `PutEvaluations` request. For example, an Lambda function for a custom Config rule cannot pass an `INSUFFICIENT_DATA` value to Config.
*/
public val complianceType: aws.sdk.kotlin.services.configservice.model.ComplianceType = requireNotNull(builder.complianceType) { "A non-null value must be provided for complianceType" }
/**
* The time of the event in Config that triggered the evaluation. For event-based evaluations, the time indicates when Config created the configuration item that triggered the evaluation. For periodic evaluations, the time indicates when Config triggered the evaluation at the frequency that you specified (for example, every 24 hours).
*/
public val orderingTimestamp: aws.smithy.kotlin.runtime.time.Instant = requireNotNull(builder.orderingTimestamp) { "A non-null value must be provided for orderingTimestamp" }
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.configservice.model.Evaluation = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("Evaluation(")
append("annotation=$annotation,")
append("complianceResourceId=$complianceResourceId,")
append("complianceResourceType=$complianceResourceType,")
append("complianceType=$complianceType,")
append("orderingTimestamp=$orderingTimestamp")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = annotation?.hashCode() ?: 0
result = 31 * result + (complianceResourceId.hashCode())
result = 31 * result + (complianceResourceType.hashCode())
result = 31 * result + (complianceType.hashCode())
result = 31 * result + (orderingTimestamp.hashCode())
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as Evaluation
if (annotation != other.annotation) return false
if (complianceResourceId != other.complianceResourceId) return false
if (complianceResourceType != other.complianceResourceType) return false
if (complianceType != other.complianceType) return false
if (orderingTimestamp != other.orderingTimestamp) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.configservice.model.Evaluation = Builder(this).apply(block).build()
public class Builder {
/**
* Supplementary information about how the evaluation determined the compliance.
*/
public var annotation: kotlin.String? = null
/**
* The ID of the Amazon Web Services resource that was evaluated.
*/
public var complianceResourceId: kotlin.String? = null
/**
* The type of Amazon Web Services resource that was evaluated.
*/
public var complianceResourceType: kotlin.String? = null
/**
* Indicates whether the Amazon Web Services resource complies with the Config rule that it was evaluated against.
*
* For the `Evaluation` data type, Config supports only the `COMPLIANT`, `NON_COMPLIANT`, and `NOT_APPLICABLE` values. Config does not support the `INSUFFICIENT_DATA` value for this data type.
*
* Similarly, Config does not accept `INSUFFICIENT_DATA` as the value for `ComplianceType` from a `PutEvaluations` request. For example, an Lambda function for a custom Config rule cannot pass an `INSUFFICIENT_DATA` value to Config.
*/
public var complianceType: aws.sdk.kotlin.services.configservice.model.ComplianceType? = null
/**
* The time of the event in Config that triggered the evaluation. For event-based evaluations, the time indicates when Config created the configuration item that triggered the evaluation. For periodic evaluations, the time indicates when Config triggered the evaluation at the frequency that you specified (for example, every 24 hours).
*/
public var orderingTimestamp: aws.smithy.kotlin.runtime.time.Instant? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.configservice.model.Evaluation) : this() {
this.annotation = x.annotation
this.complianceResourceId = x.complianceResourceId
this.complianceResourceType = x.complianceResourceType
this.complianceType = x.complianceType
this.orderingTimestamp = x.orderingTimestamp
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.configservice.model.Evaluation = Evaluation(this)
internal fun correctErrors(): Builder {
if (complianceResourceId == null) complianceResourceId = ""
if (complianceResourceType == null) complianceResourceType = ""
if (complianceType == null) complianceType = ComplianceType.SdkUnknown("no value provided")
if (orderingTimestamp == null) orderingTimestamp = Instant.fromEpochSeconds(0)
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy