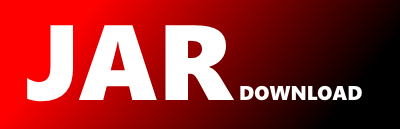
commonMain.aws.sdk.kotlin.services.configservice.model.GetResourceConfigHistoryRequest.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of configservice-jvm Show documentation
Show all versions of configservice-jvm Show documentation
The AWS Kotlin client for Config Service
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.configservice.model
import aws.smithy.kotlin.runtime.time.Instant
/**
* The input for the GetResourceConfigHistory action.
*/
public class GetResourceConfigHistoryRequest private constructor(builder: Builder) {
/**
* The chronological order for configuration items listed. By default, the results are listed in reverse chronological order.
*/
public val chronologicalOrder: aws.sdk.kotlin.services.configservice.model.ChronologicalOrder? = builder.chronologicalOrder
/**
* The chronologically earliest time in the time range for which the history requested. If not specified, the action returns paginated results that contain configuration items that start when the first configuration item was recorded.
*/
public val earlierTime: aws.smithy.kotlin.runtime.time.Instant? = builder.earlierTime
/**
* The chronologically latest time in the time range for which the history requested. If not specified, current time is taken.
*/
public val laterTime: aws.smithy.kotlin.runtime.time.Instant? = builder.laterTime
/**
* The maximum number of configuration items returned on each page. The default is 10. You cannot specify a number greater than 100. If you specify 0, Config uses the default.
*/
public val limit: kotlin.Int? = builder.limit
/**
* The `nextToken` string returned on a previous page that you use to get the next page of results in a paginated response.
*/
public val nextToken: kotlin.String? = builder.nextToken
/**
* The ID of the resource (for example., `sg-xxxxxx`).
*/
public val resourceId: kotlin.String? = builder.resourceId
/**
* The resource type.
*/
public val resourceType: aws.sdk.kotlin.services.configservice.model.ResourceType? = builder.resourceType
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.configservice.model.GetResourceConfigHistoryRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("GetResourceConfigHistoryRequest(")
append("chronologicalOrder=$chronologicalOrder,")
append("earlierTime=$earlierTime,")
append("laterTime=$laterTime,")
append("limit=$limit,")
append("nextToken=$nextToken,")
append("resourceId=$resourceId,")
append("resourceType=$resourceType")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = chronologicalOrder?.hashCode() ?: 0
result = 31 * result + (earlierTime?.hashCode() ?: 0)
result = 31 * result + (laterTime?.hashCode() ?: 0)
result = 31 * result + (limit ?: 0)
result = 31 * result + (nextToken?.hashCode() ?: 0)
result = 31 * result + (resourceId?.hashCode() ?: 0)
result = 31 * result + (resourceType?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as GetResourceConfigHistoryRequest
if (chronologicalOrder != other.chronologicalOrder) return false
if (earlierTime != other.earlierTime) return false
if (laterTime != other.laterTime) return false
if (limit != other.limit) return false
if (nextToken != other.nextToken) return false
if (resourceId != other.resourceId) return false
if (resourceType != other.resourceType) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.configservice.model.GetResourceConfigHistoryRequest = Builder(this).apply(block).build()
public class Builder {
/**
* The chronological order for configuration items listed. By default, the results are listed in reverse chronological order.
*/
public var chronologicalOrder: aws.sdk.kotlin.services.configservice.model.ChronologicalOrder? = null
/**
* The chronologically earliest time in the time range for which the history requested. If not specified, the action returns paginated results that contain configuration items that start when the first configuration item was recorded.
*/
public var earlierTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The chronologically latest time in the time range for which the history requested. If not specified, current time is taken.
*/
public var laterTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The maximum number of configuration items returned on each page. The default is 10. You cannot specify a number greater than 100. If you specify 0, Config uses the default.
*/
public var limit: kotlin.Int? = null
/**
* The `nextToken` string returned on a previous page that you use to get the next page of results in a paginated response.
*/
public var nextToken: kotlin.String? = null
/**
* The ID of the resource (for example., `sg-xxxxxx`).
*/
public var resourceId: kotlin.String? = null
/**
* The resource type.
*/
public var resourceType: aws.sdk.kotlin.services.configservice.model.ResourceType? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.configservice.model.GetResourceConfigHistoryRequest) : this() {
this.chronologicalOrder = x.chronologicalOrder
this.earlierTime = x.earlierTime
this.laterTime = x.laterTime
this.limit = x.limit
this.nextToken = x.nextToken
this.resourceId = x.resourceId
this.resourceType = x.resourceType
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.configservice.model.GetResourceConfigHistoryRequest = GetResourceConfigHistoryRequest(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy