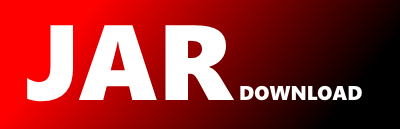
commonMain.aws.sdk.kotlin.services.configservice.model.PutConformancePackRequest.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of configservice-jvm Show documentation
Show all versions of configservice-jvm Show documentation
The AWS Kotlin client for Config Service
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.configservice.model
public class PutConformancePackRequest private constructor(builder: Builder) {
/**
* A list of `ConformancePackInputParameter` objects.
*/
public val conformancePackInputParameters: List? = builder.conformancePackInputParameters
/**
* The unique name of the conformance pack you want to deploy.
*/
public val conformancePackName: kotlin.String? = builder.conformancePackName
/**
* The name of the Amazon S3 bucket where Config stores conformance pack templates.
*
* This field is optional.
*/
public val deliveryS3Bucket: kotlin.String? = builder.deliveryS3Bucket
/**
* The prefix for the Amazon S3 bucket.
*
* This field is optional.
*/
public val deliveryS3KeyPrefix: kotlin.String? = builder.deliveryS3KeyPrefix
/**
* A string containing the full conformance pack template body. The structure containing the template body has a minimum length of 1 byte and a maximum length of 51,200 bytes.
*
* You can use a YAML template with two resource types: Config rule (`AWS::Config::ConfigRule`) and remediation action (`AWS::Config::RemediationConfiguration`).
*/
public val templateBody: kotlin.String? = builder.templateBody
/**
* The location of the file containing the template body (`s3://bucketname/prefix`). The uri must point to a conformance pack template (max size: 300 KB) that is located in an Amazon S3 bucket in the same Region as the conformance pack.
*
* You must have access to read Amazon S3 bucket. In addition, in order to ensure a successful deployment, the template object must not be in an [archived storage class](https://docs.aws.amazon.com/AmazonS3/latest/userguide/storage-class-intro.html) if this parameter is passed.
*/
public val templateS3Uri: kotlin.String? = builder.templateS3Uri
/**
* An object of type `TemplateSSMDocumentDetails`, which contains the name or the Amazon Resource Name (ARN) of the Amazon Web Services Systems Manager document (SSM document) and the version of the SSM document that is used to create a conformance pack.
*/
public val templateSsmDocumentDetails: aws.sdk.kotlin.services.configservice.model.TemplateSsmDocumentDetails? = builder.templateSsmDocumentDetails
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.configservice.model.PutConformancePackRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("PutConformancePackRequest(")
append("conformancePackInputParameters=$conformancePackInputParameters,")
append("conformancePackName=$conformancePackName,")
append("deliveryS3Bucket=$deliveryS3Bucket,")
append("deliveryS3KeyPrefix=$deliveryS3KeyPrefix,")
append("templateBody=$templateBody,")
append("templateS3Uri=$templateS3Uri,")
append("templateSsmDocumentDetails=$templateSsmDocumentDetails")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = conformancePackInputParameters?.hashCode() ?: 0
result = 31 * result + (conformancePackName?.hashCode() ?: 0)
result = 31 * result + (deliveryS3Bucket?.hashCode() ?: 0)
result = 31 * result + (deliveryS3KeyPrefix?.hashCode() ?: 0)
result = 31 * result + (templateBody?.hashCode() ?: 0)
result = 31 * result + (templateS3Uri?.hashCode() ?: 0)
result = 31 * result + (templateSsmDocumentDetails?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as PutConformancePackRequest
if (conformancePackInputParameters != other.conformancePackInputParameters) return false
if (conformancePackName != other.conformancePackName) return false
if (deliveryS3Bucket != other.deliveryS3Bucket) return false
if (deliveryS3KeyPrefix != other.deliveryS3KeyPrefix) return false
if (templateBody != other.templateBody) return false
if (templateS3Uri != other.templateS3Uri) return false
if (templateSsmDocumentDetails != other.templateSsmDocumentDetails) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.configservice.model.PutConformancePackRequest = Builder(this).apply(block).build()
public class Builder {
/**
* A list of `ConformancePackInputParameter` objects.
*/
public var conformancePackInputParameters: List? = null
/**
* The unique name of the conformance pack you want to deploy.
*/
public var conformancePackName: kotlin.String? = null
/**
* The name of the Amazon S3 bucket where Config stores conformance pack templates.
*
* This field is optional.
*/
public var deliveryS3Bucket: kotlin.String? = null
/**
* The prefix for the Amazon S3 bucket.
*
* This field is optional.
*/
public var deliveryS3KeyPrefix: kotlin.String? = null
/**
* A string containing the full conformance pack template body. The structure containing the template body has a minimum length of 1 byte and a maximum length of 51,200 bytes.
*
* You can use a YAML template with two resource types: Config rule (`AWS::Config::ConfigRule`) and remediation action (`AWS::Config::RemediationConfiguration`).
*/
public var templateBody: kotlin.String? = null
/**
* The location of the file containing the template body (`s3://bucketname/prefix`). The uri must point to a conformance pack template (max size: 300 KB) that is located in an Amazon S3 bucket in the same Region as the conformance pack.
*
* You must have access to read Amazon S3 bucket. In addition, in order to ensure a successful deployment, the template object must not be in an [archived storage class](https://docs.aws.amazon.com/AmazonS3/latest/userguide/storage-class-intro.html) if this parameter is passed.
*/
public var templateS3Uri: kotlin.String? = null
/**
* An object of type `TemplateSSMDocumentDetails`, which contains the name or the Amazon Resource Name (ARN) of the Amazon Web Services Systems Manager document (SSM document) and the version of the SSM document that is used to create a conformance pack.
*/
public var templateSsmDocumentDetails: aws.sdk.kotlin.services.configservice.model.TemplateSsmDocumentDetails? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.configservice.model.PutConformancePackRequest) : this() {
this.conformancePackInputParameters = x.conformancePackInputParameters
this.conformancePackName = x.conformancePackName
this.deliveryS3Bucket = x.deliveryS3Bucket
this.deliveryS3KeyPrefix = x.deliveryS3KeyPrefix
this.templateBody = x.templateBody
this.templateS3Uri = x.templateS3Uri
this.templateSsmDocumentDetails = x.templateSsmDocumentDetails
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.configservice.model.PutConformancePackRequest = PutConformancePackRequest(this)
/**
* construct an [aws.sdk.kotlin.services.configservice.model.TemplateSsmDocumentDetails] inside the given [block]
*/
public fun templateSsmDocumentDetails(block: aws.sdk.kotlin.services.configservice.model.TemplateSsmDocumentDetails.Builder.() -> kotlin.Unit) {
this.templateSsmDocumentDetails = aws.sdk.kotlin.services.configservice.model.TemplateSsmDocumentDetails.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy