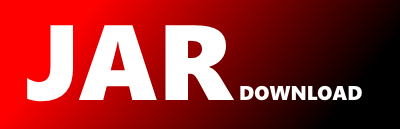
commonMain.aws.sdk.kotlin.services.configservice.model.PutOrganizationConfigRuleRequest.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of configservice-jvm Show documentation
Show all versions of configservice-jvm Show documentation
The AWS Kotlin client for Config Service
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.configservice.model
public class PutOrganizationConfigRuleRequest private constructor(builder: Builder) {
/**
* A comma-separated list of accounts that you want to exclude from an organization Config rule.
*/
public val excludedAccounts: List? = builder.excludedAccounts
/**
* The name that you assign to an organization Config rule.
*/
public val organizationConfigRuleName: kotlin.String? = builder.organizationConfigRuleName
/**
* An `OrganizationCustomPolicyRuleMetadata` object. This object specifies metadata for your organization's Config Custom Policy rule. The metadata includes the runtime system in use, which accounts have debug logging enabled, and other custom rule metadata, such as resource type, resource ID of Amazon Web Services resource, and organization trigger types that initiate Config to evaluate Amazon Web Services resources against a rule.
*/
public val organizationCustomPolicyRuleMetadata: aws.sdk.kotlin.services.configservice.model.OrganizationCustomPolicyRuleMetadata? = builder.organizationCustomPolicyRuleMetadata
/**
* An `OrganizationCustomRuleMetadata` object. This object specifies organization custom rule metadata such as resource type, resource ID of Amazon Web Services resource, Lambda function ARN, and organization trigger types that trigger Config to evaluate your Amazon Web Services resources against a rule. It also provides the frequency with which you want Config to run evaluations for the rule if the trigger type is periodic.
*/
public val organizationCustomRuleMetadata: aws.sdk.kotlin.services.configservice.model.OrganizationCustomRuleMetadata? = builder.organizationCustomRuleMetadata
/**
* An `OrganizationManagedRuleMetadata` object. This object specifies organization managed rule metadata such as resource type and ID of Amazon Web Services resource along with the rule identifier. It also provides the frequency with which you want Config to run evaluations for the rule if the trigger type is periodic.
*/
public val organizationManagedRuleMetadata: aws.sdk.kotlin.services.configservice.model.OrganizationManagedRuleMetadata? = builder.organizationManagedRuleMetadata
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.configservice.model.PutOrganizationConfigRuleRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("PutOrganizationConfigRuleRequest(")
append("excludedAccounts=$excludedAccounts,")
append("organizationConfigRuleName=$organizationConfigRuleName,")
append("organizationCustomPolicyRuleMetadata=$organizationCustomPolicyRuleMetadata,")
append("organizationCustomRuleMetadata=$organizationCustomRuleMetadata,")
append("organizationManagedRuleMetadata=$organizationManagedRuleMetadata")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = excludedAccounts?.hashCode() ?: 0
result = 31 * result + (organizationConfigRuleName?.hashCode() ?: 0)
result = 31 * result + (organizationCustomPolicyRuleMetadata?.hashCode() ?: 0)
result = 31 * result + (organizationCustomRuleMetadata?.hashCode() ?: 0)
result = 31 * result + (organizationManagedRuleMetadata?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as PutOrganizationConfigRuleRequest
if (excludedAccounts != other.excludedAccounts) return false
if (organizationConfigRuleName != other.organizationConfigRuleName) return false
if (organizationCustomPolicyRuleMetadata != other.organizationCustomPolicyRuleMetadata) return false
if (organizationCustomRuleMetadata != other.organizationCustomRuleMetadata) return false
if (organizationManagedRuleMetadata != other.organizationManagedRuleMetadata) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.configservice.model.PutOrganizationConfigRuleRequest = Builder(this).apply(block).build()
public class Builder {
/**
* A comma-separated list of accounts that you want to exclude from an organization Config rule.
*/
public var excludedAccounts: List? = null
/**
* The name that you assign to an organization Config rule.
*/
public var organizationConfigRuleName: kotlin.String? = null
/**
* An `OrganizationCustomPolicyRuleMetadata` object. This object specifies metadata for your organization's Config Custom Policy rule. The metadata includes the runtime system in use, which accounts have debug logging enabled, and other custom rule metadata, such as resource type, resource ID of Amazon Web Services resource, and organization trigger types that initiate Config to evaluate Amazon Web Services resources against a rule.
*/
public var organizationCustomPolicyRuleMetadata: aws.sdk.kotlin.services.configservice.model.OrganizationCustomPolicyRuleMetadata? = null
/**
* An `OrganizationCustomRuleMetadata` object. This object specifies organization custom rule metadata such as resource type, resource ID of Amazon Web Services resource, Lambda function ARN, and organization trigger types that trigger Config to evaluate your Amazon Web Services resources against a rule. It also provides the frequency with which you want Config to run evaluations for the rule if the trigger type is periodic.
*/
public var organizationCustomRuleMetadata: aws.sdk.kotlin.services.configservice.model.OrganizationCustomRuleMetadata? = null
/**
* An `OrganizationManagedRuleMetadata` object. This object specifies organization managed rule metadata such as resource type and ID of Amazon Web Services resource along with the rule identifier. It also provides the frequency with which you want Config to run evaluations for the rule if the trigger type is periodic.
*/
public var organizationManagedRuleMetadata: aws.sdk.kotlin.services.configservice.model.OrganizationManagedRuleMetadata? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.configservice.model.PutOrganizationConfigRuleRequest) : this() {
this.excludedAccounts = x.excludedAccounts
this.organizationConfigRuleName = x.organizationConfigRuleName
this.organizationCustomPolicyRuleMetadata = x.organizationCustomPolicyRuleMetadata
this.organizationCustomRuleMetadata = x.organizationCustomRuleMetadata
this.organizationManagedRuleMetadata = x.organizationManagedRuleMetadata
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.configservice.model.PutOrganizationConfigRuleRequest = PutOrganizationConfigRuleRequest(this)
/**
* construct an [aws.sdk.kotlin.services.configservice.model.OrganizationCustomPolicyRuleMetadata] inside the given [block]
*/
public fun organizationCustomPolicyRuleMetadata(block: aws.sdk.kotlin.services.configservice.model.OrganizationCustomPolicyRuleMetadata.Builder.() -> kotlin.Unit) {
this.organizationCustomPolicyRuleMetadata = aws.sdk.kotlin.services.configservice.model.OrganizationCustomPolicyRuleMetadata.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.configservice.model.OrganizationCustomRuleMetadata] inside the given [block]
*/
public fun organizationCustomRuleMetadata(block: aws.sdk.kotlin.services.configservice.model.OrganizationCustomRuleMetadata.Builder.() -> kotlin.Unit) {
this.organizationCustomRuleMetadata = aws.sdk.kotlin.services.configservice.model.OrganizationCustomRuleMetadata.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.configservice.model.OrganizationManagedRuleMetadata] inside the given [block]
*/
public fun organizationManagedRuleMetadata(block: aws.sdk.kotlin.services.configservice.model.OrganizationManagedRuleMetadata.Builder.() -> kotlin.Unit) {
this.organizationManagedRuleMetadata = aws.sdk.kotlin.services.configservice.model.OrganizationManagedRuleMetadata.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy