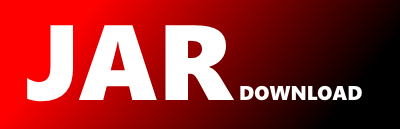
commonMain.aws.sdk.kotlin.services.configservice.model.ConformancePackDetail.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.configservice.model
import aws.smithy.kotlin.runtime.SdkDsl
import aws.smithy.kotlin.runtime.time.Instant
/**
* Returns details of a conformance pack. A conformance pack is a collection of Config rules and remediation actions that can be easily deployed in an account and a region.
*/
public class ConformancePackDetail private constructor(builder: Builder) {
/**
* Amazon Resource Name (ARN) of the conformance pack.
*/
public val conformancePackArn: kotlin.String = requireNotNull(builder.conformancePackArn) { "A non-null value must be provided for conformancePackArn" }
/**
* ID of the conformance pack.
*/
public val conformancePackId: kotlin.String = requireNotNull(builder.conformancePackId) { "A non-null value must be provided for conformancePackId" }
/**
* A list of `ConformancePackInputParameter` objects.
*/
public val conformancePackInputParameters: List? = builder.conformancePackInputParameters
/**
* Name of the conformance pack.
*/
public val conformancePackName: kotlin.String = requireNotNull(builder.conformancePackName) { "A non-null value must be provided for conformancePackName" }
/**
* The Amazon Web Services service that created the conformance pack.
*/
public val createdBy: kotlin.String? = builder.createdBy
/**
* The name of the Amazon S3 bucket where Config stores conformance pack templates.
*
* This field is optional.
*/
public val deliveryS3Bucket: kotlin.String? = builder.deliveryS3Bucket
/**
* The prefix for the Amazon S3 bucket.
*
* This field is optional.
*/
public val deliveryS3KeyPrefix: kotlin.String? = builder.deliveryS3KeyPrefix
/**
* The last time a conformation pack update was requested.
*/
public val lastUpdateRequestedTime: aws.smithy.kotlin.runtime.time.Instant? = builder.lastUpdateRequestedTime
/**
* An object that contains the name or Amazon Resource Name (ARN) of the Amazon Web Services Systems Manager document (SSM document) and the version of the SSM document that is used to create a conformance pack.
*/
public val templateSsmDocumentDetails: aws.sdk.kotlin.services.configservice.model.TemplateSsmDocumentDetails? = builder.templateSsmDocumentDetails
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.configservice.model.ConformancePackDetail = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("ConformancePackDetail(")
append("conformancePackArn=$conformancePackArn,")
append("conformancePackId=$conformancePackId,")
append("conformancePackInputParameters=$conformancePackInputParameters,")
append("conformancePackName=$conformancePackName,")
append("createdBy=$createdBy,")
append("deliveryS3Bucket=$deliveryS3Bucket,")
append("deliveryS3KeyPrefix=$deliveryS3KeyPrefix,")
append("lastUpdateRequestedTime=$lastUpdateRequestedTime,")
append("templateSsmDocumentDetails=$templateSsmDocumentDetails")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = conformancePackArn.hashCode()
result = 31 * result + (conformancePackId.hashCode())
result = 31 * result + (conformancePackInputParameters?.hashCode() ?: 0)
result = 31 * result + (conformancePackName.hashCode())
result = 31 * result + (createdBy?.hashCode() ?: 0)
result = 31 * result + (deliveryS3Bucket?.hashCode() ?: 0)
result = 31 * result + (deliveryS3KeyPrefix?.hashCode() ?: 0)
result = 31 * result + (lastUpdateRequestedTime?.hashCode() ?: 0)
result = 31 * result + (templateSsmDocumentDetails?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as ConformancePackDetail
if (conformancePackArn != other.conformancePackArn) return false
if (conformancePackId != other.conformancePackId) return false
if (conformancePackInputParameters != other.conformancePackInputParameters) return false
if (conformancePackName != other.conformancePackName) return false
if (createdBy != other.createdBy) return false
if (deliveryS3Bucket != other.deliveryS3Bucket) return false
if (deliveryS3KeyPrefix != other.deliveryS3KeyPrefix) return false
if (lastUpdateRequestedTime != other.lastUpdateRequestedTime) return false
if (templateSsmDocumentDetails != other.templateSsmDocumentDetails) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.configservice.model.ConformancePackDetail = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* Amazon Resource Name (ARN) of the conformance pack.
*/
public var conformancePackArn: kotlin.String? = null
/**
* ID of the conformance pack.
*/
public var conformancePackId: kotlin.String? = null
/**
* A list of `ConformancePackInputParameter` objects.
*/
public var conformancePackInputParameters: List? = null
/**
* Name of the conformance pack.
*/
public var conformancePackName: kotlin.String? = null
/**
* The Amazon Web Services service that created the conformance pack.
*/
public var createdBy: kotlin.String? = null
/**
* The name of the Amazon S3 bucket where Config stores conformance pack templates.
*
* This field is optional.
*/
public var deliveryS3Bucket: kotlin.String? = null
/**
* The prefix for the Amazon S3 bucket.
*
* This field is optional.
*/
public var deliveryS3KeyPrefix: kotlin.String? = null
/**
* The last time a conformation pack update was requested.
*/
public var lastUpdateRequestedTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* An object that contains the name or Amazon Resource Name (ARN) of the Amazon Web Services Systems Manager document (SSM document) and the version of the SSM document that is used to create a conformance pack.
*/
public var templateSsmDocumentDetails: aws.sdk.kotlin.services.configservice.model.TemplateSsmDocumentDetails? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.configservice.model.ConformancePackDetail) : this() {
this.conformancePackArn = x.conformancePackArn
this.conformancePackId = x.conformancePackId
this.conformancePackInputParameters = x.conformancePackInputParameters
this.conformancePackName = x.conformancePackName
this.createdBy = x.createdBy
this.deliveryS3Bucket = x.deliveryS3Bucket
this.deliveryS3KeyPrefix = x.deliveryS3KeyPrefix
this.lastUpdateRequestedTime = x.lastUpdateRequestedTime
this.templateSsmDocumentDetails = x.templateSsmDocumentDetails
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.configservice.model.ConformancePackDetail = ConformancePackDetail(this)
/**
* construct an [aws.sdk.kotlin.services.configservice.model.TemplateSsmDocumentDetails] inside the given [block]
*/
public fun templateSsmDocumentDetails(block: aws.sdk.kotlin.services.configservice.model.TemplateSsmDocumentDetails.Builder.() -> kotlin.Unit) {
this.templateSsmDocumentDetails = aws.sdk.kotlin.services.configservice.model.TemplateSsmDocumentDetails.invoke(block)
}
internal fun correctErrors(): Builder {
if (conformancePackArn == null) conformancePackArn = ""
if (conformancePackId == null) conformancePackId = ""
if (conformancePackName == null) conformancePackName = ""
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy