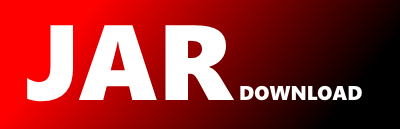
commonMain.aws.sdk.kotlin.services.configservice.model.MemberAccountStatus.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.configservice.model
import aws.smithy.kotlin.runtime.SdkDsl
import aws.smithy.kotlin.runtime.time.Instant
/**
* Organization Config rule creation or deletion status in each member account. This includes the name of the rule, the status, error code and error message when the rule creation or deletion failed.
*/
public class MemberAccountStatus private constructor(builder: Builder) {
/**
* The 12-digit account ID of a member account.
*/
public val accountId: kotlin.String = requireNotNull(builder.accountId) { "A non-null value must be provided for accountId" }
/**
* The name of Config rule deployed in the member account.
*/
public val configRuleName: kotlin.String = requireNotNull(builder.configRuleName) { "A non-null value must be provided for configRuleName" }
/**
* An error code that is returned when Config rule creation or deletion failed in the member account.
*/
public val errorCode: kotlin.String? = builder.errorCode
/**
* An error message indicating that Config rule account creation or deletion has failed due to an error in the member account.
*/
public val errorMessage: kotlin.String? = builder.errorMessage
/**
* The timestamp of the last status update.
*/
public val lastUpdateTime: aws.smithy.kotlin.runtime.time.Instant? = builder.lastUpdateTime
/**
* Indicates deployment status for Config rule in the member account. When management account calls `PutOrganizationConfigRule` action for the first time, Config rule status is created in the member account. When management account calls `PutOrganizationConfigRule` action for the second time, Config rule status is updated in the member account. Config rule status is deleted when the management account deletes `OrganizationConfigRule` and disables service access for `config-multiaccountsetup.amazonaws.com`.
*
* Config sets the state of the rule to:
* + `CREATE_SUCCESSFUL` when Config rule has been created in the member account.
* + `CREATE_IN_PROGRESS` when Config rule is being created in the member account.
* + `CREATE_FAILED` when Config rule creation has failed in the member account.
* + `DELETE_FAILED` when Config rule deletion has failed in the member account.
* + `DELETE_IN_PROGRESS` when Config rule is being deleted in the member account.
* + `DELETE_SUCCESSFUL` when Config rule has been deleted in the member account.
* + `UPDATE_SUCCESSFUL` when Config rule has been updated in the member account.
* + `UPDATE_IN_PROGRESS` when Config rule is being updated in the member account.
* + `UPDATE_FAILED` when Config rule deletion has failed in the member account.
*/
public val memberAccountRuleStatus: aws.sdk.kotlin.services.configservice.model.MemberAccountRuleStatus = requireNotNull(builder.memberAccountRuleStatus) { "A non-null value must be provided for memberAccountRuleStatus" }
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.configservice.model.MemberAccountStatus = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("MemberAccountStatus(")
append("accountId=$accountId,")
append("configRuleName=$configRuleName,")
append("errorCode=$errorCode,")
append("errorMessage=$errorMessage,")
append("lastUpdateTime=$lastUpdateTime,")
append("memberAccountRuleStatus=$memberAccountRuleStatus")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = accountId.hashCode()
result = 31 * result + (configRuleName.hashCode())
result = 31 * result + (errorCode?.hashCode() ?: 0)
result = 31 * result + (errorMessage?.hashCode() ?: 0)
result = 31 * result + (lastUpdateTime?.hashCode() ?: 0)
result = 31 * result + (memberAccountRuleStatus.hashCode())
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as MemberAccountStatus
if (accountId != other.accountId) return false
if (configRuleName != other.configRuleName) return false
if (errorCode != other.errorCode) return false
if (errorMessage != other.errorMessage) return false
if (lastUpdateTime != other.lastUpdateTime) return false
if (memberAccountRuleStatus != other.memberAccountRuleStatus) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.configservice.model.MemberAccountStatus = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The 12-digit account ID of a member account.
*/
public var accountId: kotlin.String? = null
/**
* The name of Config rule deployed in the member account.
*/
public var configRuleName: kotlin.String? = null
/**
* An error code that is returned when Config rule creation or deletion failed in the member account.
*/
public var errorCode: kotlin.String? = null
/**
* An error message indicating that Config rule account creation or deletion has failed due to an error in the member account.
*/
public var errorMessage: kotlin.String? = null
/**
* The timestamp of the last status update.
*/
public var lastUpdateTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* Indicates deployment status for Config rule in the member account. When management account calls `PutOrganizationConfigRule` action for the first time, Config rule status is created in the member account. When management account calls `PutOrganizationConfigRule` action for the second time, Config rule status is updated in the member account. Config rule status is deleted when the management account deletes `OrganizationConfigRule` and disables service access for `config-multiaccountsetup.amazonaws.com`.
*
* Config sets the state of the rule to:
* + `CREATE_SUCCESSFUL` when Config rule has been created in the member account.
* + `CREATE_IN_PROGRESS` when Config rule is being created in the member account.
* + `CREATE_FAILED` when Config rule creation has failed in the member account.
* + `DELETE_FAILED` when Config rule deletion has failed in the member account.
* + `DELETE_IN_PROGRESS` when Config rule is being deleted in the member account.
* + `DELETE_SUCCESSFUL` when Config rule has been deleted in the member account.
* + `UPDATE_SUCCESSFUL` when Config rule has been updated in the member account.
* + `UPDATE_IN_PROGRESS` when Config rule is being updated in the member account.
* + `UPDATE_FAILED` when Config rule deletion has failed in the member account.
*/
public var memberAccountRuleStatus: aws.sdk.kotlin.services.configservice.model.MemberAccountRuleStatus? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.configservice.model.MemberAccountStatus) : this() {
this.accountId = x.accountId
this.configRuleName = x.configRuleName
this.errorCode = x.errorCode
this.errorMessage = x.errorMessage
this.lastUpdateTime = x.lastUpdateTime
this.memberAccountRuleStatus = x.memberAccountRuleStatus
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.configservice.model.MemberAccountStatus = MemberAccountStatus(this)
internal fun correctErrors(): Builder {
if (accountId == null) accountId = ""
if (configRuleName == null) configRuleName = ""
if (memberAccountRuleStatus == null) memberAccountRuleStatus = MemberAccountRuleStatus.SdkUnknown("no value provided")
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy