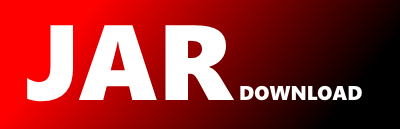
commonMain.aws.sdk.kotlin.services.configservice.model.Source.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.configservice.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Provides the CustomPolicyDetails, the rule owner (`Amazon Web Services` for managed rules, `CUSTOM_POLICY` for Custom Policy rules, and `CUSTOM_LAMBDA` for Custom Lambda rules), the rule identifier, and the events that cause the evaluation of your Amazon Web Services resources.
*/
public class Source private constructor(builder: Builder) {
/**
* Provides the runtime system, policy definition, and whether debug logging is enabled. Required when owner is set to `CUSTOM_POLICY`.
*/
public val customPolicyDetails: aws.sdk.kotlin.services.configservice.model.CustomPolicyDetails? = builder.customPolicyDetails
/**
* Indicates whether Amazon Web Services or the customer owns and manages the Config rule.
*
* Config Managed Rules are predefined rules owned by Amazon Web Services. For more information, see [Config Managed Rules](https://docs.aws.amazon.com/config/latest/developerguide/evaluate-config_use-managed-rules.html) in the *Config developer guide*.
*
* Config Custom Rules are rules that you can develop either with Guard (`CUSTOM_POLICY`) or Lambda (`CUSTOM_LAMBDA`). For more information, see [Config Custom Rules ](https://docs.aws.amazon.com/config/latest/developerguide/evaluate-config_develop-rules.html) in the *Config developer guide*.
*/
public val owner: aws.sdk.kotlin.services.configservice.model.Owner = requireNotNull(builder.owner) { "A non-null value must be provided for owner" }
/**
* Provides the source and the message types that cause Config to evaluate your Amazon Web Services resources against a rule. It also provides the frequency with which you want Config to run evaluations for the rule if the trigger type is periodic.
*
* If the owner is set to `CUSTOM_POLICY`, the only acceptable values for the Config rule trigger message type are `ConfigurationItemChangeNotification` and `OversizedConfigurationItemChangeNotification`.
*/
public val sourceDetails: List? = builder.sourceDetails
/**
* For Config Managed rules, a predefined identifier from a list. For example, `IAM_PASSWORD_POLICY` is a managed rule. To reference a managed rule, see [List of Config Managed Rules](https://docs.aws.amazon.com/config/latest/developerguide/managed-rules-by-aws-config.html).
*
* For Config Custom Lambda rules, the identifier is the Amazon Resource Name (ARN) of the rule's Lambda function, such as `arn:aws:lambda:us-east-2:123456789012:function:custom_rule_name`.
*
* For Config Custom Policy rules, this field will be ignored.
*/
public val sourceIdentifier: kotlin.String? = builder.sourceIdentifier
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.configservice.model.Source = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("Source(")
append("customPolicyDetails=$customPolicyDetails,")
append("owner=$owner,")
append("sourceDetails=$sourceDetails,")
append("sourceIdentifier=$sourceIdentifier")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = customPolicyDetails?.hashCode() ?: 0
result = 31 * result + (owner.hashCode())
result = 31 * result + (sourceDetails?.hashCode() ?: 0)
result = 31 * result + (sourceIdentifier?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as Source
if (customPolicyDetails != other.customPolicyDetails) return false
if (owner != other.owner) return false
if (sourceDetails != other.sourceDetails) return false
if (sourceIdentifier != other.sourceIdentifier) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.configservice.model.Source = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* Provides the runtime system, policy definition, and whether debug logging is enabled. Required when owner is set to `CUSTOM_POLICY`.
*/
public var customPolicyDetails: aws.sdk.kotlin.services.configservice.model.CustomPolicyDetails? = null
/**
* Indicates whether Amazon Web Services or the customer owns and manages the Config rule.
*
* Config Managed Rules are predefined rules owned by Amazon Web Services. For more information, see [Config Managed Rules](https://docs.aws.amazon.com/config/latest/developerguide/evaluate-config_use-managed-rules.html) in the *Config developer guide*.
*
* Config Custom Rules are rules that you can develop either with Guard (`CUSTOM_POLICY`) or Lambda (`CUSTOM_LAMBDA`). For more information, see [Config Custom Rules ](https://docs.aws.amazon.com/config/latest/developerguide/evaluate-config_develop-rules.html) in the *Config developer guide*.
*/
public var owner: aws.sdk.kotlin.services.configservice.model.Owner? = null
/**
* Provides the source and the message types that cause Config to evaluate your Amazon Web Services resources against a rule. It also provides the frequency with which you want Config to run evaluations for the rule if the trigger type is periodic.
*
* If the owner is set to `CUSTOM_POLICY`, the only acceptable values for the Config rule trigger message type are `ConfigurationItemChangeNotification` and `OversizedConfigurationItemChangeNotification`.
*/
public var sourceDetails: List? = null
/**
* For Config Managed rules, a predefined identifier from a list. For example, `IAM_PASSWORD_POLICY` is a managed rule. To reference a managed rule, see [List of Config Managed Rules](https://docs.aws.amazon.com/config/latest/developerguide/managed-rules-by-aws-config.html).
*
* For Config Custom Lambda rules, the identifier is the Amazon Resource Name (ARN) of the rule's Lambda function, such as `arn:aws:lambda:us-east-2:123456789012:function:custom_rule_name`.
*
* For Config Custom Policy rules, this field will be ignored.
*/
public var sourceIdentifier: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.configservice.model.Source) : this() {
this.customPolicyDetails = x.customPolicyDetails
this.owner = x.owner
this.sourceDetails = x.sourceDetails
this.sourceIdentifier = x.sourceIdentifier
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.configservice.model.Source = Source(this)
/**
* construct an [aws.sdk.kotlin.services.configservice.model.CustomPolicyDetails] inside the given [block]
*/
public fun customPolicyDetails(block: aws.sdk.kotlin.services.configservice.model.CustomPolicyDetails.Builder.() -> kotlin.Unit) {
this.customPolicyDetails = aws.sdk.kotlin.services.configservice.model.CustomPolicyDetails.invoke(block)
}
internal fun correctErrors(): Builder {
if (owner == null) owner = Owner.SdkUnknown("no value provided")
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy