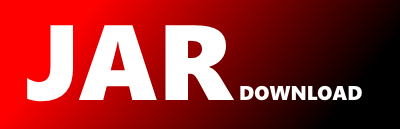
commonMain.aws.sdk.kotlin.services.configservice.model.StartResourceEvaluationRequest.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.configservice.model
import aws.smithy.kotlin.runtime.SdkDsl
public class StartResourceEvaluationRequest private constructor(builder: Builder) {
/**
* A client token is a unique, case-sensitive string of up to 64 ASCII characters. To make an idempotent API request using one of these actions, specify a client token in the request.
*
* Avoid reusing the same client token for other API requests. If you retry a request that completed successfully using the same client token and the same parameters, the retry succeeds without performing any further actions. If you retry a successful request using the same client token, but one or more of the parameters are different, other than the Region or Availability Zone, the retry fails with an IdempotentParameterMismatch error.
*/
public val clientToken: kotlin.String? = builder.clientToken
/**
* Returns an `EvaluationContext` object.
*/
public val evaluationContext: aws.sdk.kotlin.services.configservice.model.EvaluationContext? = builder.evaluationContext
/**
* The mode of an evaluation. The valid values for this API are `DETECTIVE` and `PROACTIVE`.
*/
public val evaluationMode: aws.sdk.kotlin.services.configservice.model.EvaluationMode? = builder.evaluationMode
/**
* The timeout for an evaluation. The default is 900 seconds. You cannot specify a number greater than 3600. If you specify 0, Config uses the default.
*/
public val evaluationTimeout: kotlin.Int? = builder.evaluationTimeout
/**
* Returns a `ResourceDetails` object.
*/
public val resourceDetails: aws.sdk.kotlin.services.configservice.model.ResourceDetails? = builder.resourceDetails
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.configservice.model.StartResourceEvaluationRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("StartResourceEvaluationRequest(")
append("clientToken=$clientToken,")
append("evaluationContext=$evaluationContext,")
append("evaluationMode=$evaluationMode,")
append("evaluationTimeout=$evaluationTimeout,")
append("resourceDetails=$resourceDetails")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = clientToken?.hashCode() ?: 0
result = 31 * result + (evaluationContext?.hashCode() ?: 0)
result = 31 * result + (evaluationMode?.hashCode() ?: 0)
result = 31 * result + (evaluationTimeout ?: 0)
result = 31 * result + (resourceDetails?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as StartResourceEvaluationRequest
if (clientToken != other.clientToken) return false
if (evaluationContext != other.evaluationContext) return false
if (evaluationMode != other.evaluationMode) return false
if (evaluationTimeout != other.evaluationTimeout) return false
if (resourceDetails != other.resourceDetails) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.configservice.model.StartResourceEvaluationRequest = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* A client token is a unique, case-sensitive string of up to 64 ASCII characters. To make an idempotent API request using one of these actions, specify a client token in the request.
*
* Avoid reusing the same client token for other API requests. If you retry a request that completed successfully using the same client token and the same parameters, the retry succeeds without performing any further actions. If you retry a successful request using the same client token, but one or more of the parameters are different, other than the Region or Availability Zone, the retry fails with an IdempotentParameterMismatch error.
*/
public var clientToken: kotlin.String? = null
/**
* Returns an `EvaluationContext` object.
*/
public var evaluationContext: aws.sdk.kotlin.services.configservice.model.EvaluationContext? = null
/**
* The mode of an evaluation. The valid values for this API are `DETECTIVE` and `PROACTIVE`.
*/
public var evaluationMode: aws.sdk.kotlin.services.configservice.model.EvaluationMode? = null
/**
* The timeout for an evaluation. The default is 900 seconds. You cannot specify a number greater than 3600. If you specify 0, Config uses the default.
*/
public var evaluationTimeout: kotlin.Int? = null
/**
* Returns a `ResourceDetails` object.
*/
public var resourceDetails: aws.sdk.kotlin.services.configservice.model.ResourceDetails? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.configservice.model.StartResourceEvaluationRequest) : this() {
this.clientToken = x.clientToken
this.evaluationContext = x.evaluationContext
this.evaluationMode = x.evaluationMode
this.evaluationTimeout = x.evaluationTimeout
this.resourceDetails = x.resourceDetails
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.configservice.model.StartResourceEvaluationRequest = StartResourceEvaluationRequest(this)
/**
* construct an [aws.sdk.kotlin.services.configservice.model.EvaluationContext] inside the given [block]
*/
public fun evaluationContext(block: aws.sdk.kotlin.services.configservice.model.EvaluationContext.Builder.() -> kotlin.Unit) {
this.evaluationContext = aws.sdk.kotlin.services.configservice.model.EvaluationContext.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.configservice.model.ResourceDetails] inside the given [block]
*/
public fun resourceDetails(block: aws.sdk.kotlin.services.configservice.model.ResourceDetails.Builder.() -> kotlin.Unit) {
this.resourceDetails = aws.sdk.kotlin.services.configservice.model.ResourceDetails.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy