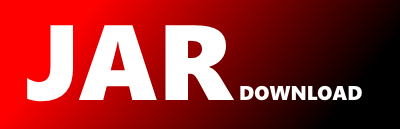
aws.sdk.kotlin.services.configservice.model.ExternalEvaluation.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of configservice Show documentation
Show all versions of configservice Show documentation
The AWS Kotlin client for Config Service
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.configservice.model
import aws.smithy.kotlin.runtime.time.Instant
/**
* Identifies an Amazon Web Services resource and indicates whether it complies with the Config rule that it was evaluated against.
*/
class ExternalEvaluation private constructor(builder: Builder) {
/**
* Supplementary information about the reason of compliance. For example, this task was completed on a specific date.
*/
val annotation: kotlin.String? = builder.annotation
/**
* The evaluated compliance resource ID. Config accepts only Amazon Web Services account ID.
*/
val complianceResourceId: kotlin.String? = builder.complianceResourceId
/**
* The evaluated compliance resource type. Config accepts AWS::::Account resource type.
*/
val complianceResourceType: kotlin.String? = builder.complianceResourceType
/**
* The compliance of the Amazon Web Services resource. The valid values are COMPLIANT, NON_COMPLIANT, and NOT_APPLICABLE.
*/
val complianceType: aws.sdk.kotlin.services.configservice.model.ComplianceType? = builder.complianceType
/**
* The time when the compliance was recorded.
*/
val orderingTimestamp: aws.smithy.kotlin.runtime.time.Instant? = builder.orderingTimestamp
companion object {
operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.configservice.model.ExternalEvaluation = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("ExternalEvaluation(")
append("annotation=$annotation,")
append("complianceResourceId=$complianceResourceId,")
append("complianceResourceType=$complianceResourceType,")
append("complianceType=$complianceType,")
append("orderingTimestamp=$orderingTimestamp)")
}
override fun hashCode(): kotlin.Int {
var result = annotation?.hashCode() ?: 0
result = 31 * result + (complianceResourceId?.hashCode() ?: 0)
result = 31 * result + (complianceResourceType?.hashCode() ?: 0)
result = 31 * result + (complianceType?.hashCode() ?: 0)
result = 31 * result + (orderingTimestamp?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as ExternalEvaluation
if (annotation != other.annotation) return false
if (complianceResourceId != other.complianceResourceId) return false
if (complianceResourceType != other.complianceResourceType) return false
if (complianceType != other.complianceType) return false
if (orderingTimestamp != other.orderingTimestamp) return false
return true
}
inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.configservice.model.ExternalEvaluation = Builder(this).apply(block).build()
class Builder {
/**
* Supplementary information about the reason of compliance. For example, this task was completed on a specific date.
*/
var annotation: kotlin.String? = null
/**
* The evaluated compliance resource ID. Config accepts only Amazon Web Services account ID.
*/
var complianceResourceId: kotlin.String? = null
/**
* The evaluated compliance resource type. Config accepts AWS::::Account resource type.
*/
var complianceResourceType: kotlin.String? = null
/**
* The compliance of the Amazon Web Services resource. The valid values are COMPLIANT, NON_COMPLIANT, and NOT_APPLICABLE.
*/
var complianceType: aws.sdk.kotlin.services.configservice.model.ComplianceType? = null
/**
* The time when the compliance was recorded.
*/
var orderingTimestamp: aws.smithy.kotlin.runtime.time.Instant? = null
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.configservice.model.ExternalEvaluation) : this() {
this.annotation = x.annotation
this.complianceResourceId = x.complianceResourceId
this.complianceResourceType = x.complianceResourceType
this.complianceType = x.complianceType
this.orderingTimestamp = x.orderingTimestamp
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.configservice.model.ExternalEvaluation = ExternalEvaluation(this)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy