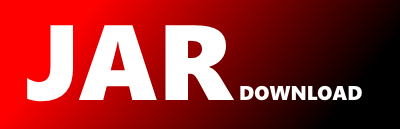
aws.sdk.kotlin.services.configservice.model.OrganizationConfigRule.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.configservice.model
import aws.smithy.kotlin.runtime.time.Instant
/**
* An organization Config rule that has information about Config rules that Config creates in member accounts.
*/
class OrganizationConfigRule private constructor(builder: Builder) {
/**
* A comma-separated list of accounts excluded from organization Config rule.
*/
val excludedAccounts: List? = builder.excludedAccounts
/**
* The timestamp of the last update.
*/
val lastUpdateTime: aws.smithy.kotlin.runtime.time.Instant? = builder.lastUpdateTime
/**
* Amazon Resource Name (ARN) of organization Config rule.
*/
val organizationConfigRuleArn: kotlin.String? = builder.organizationConfigRuleArn
/**
* The name that you assign to organization Config rule.
*/
val organizationConfigRuleName: kotlin.String? = builder.organizationConfigRuleName
/**
* An
* object that specifies metadata for your organization's Config Custom Policy rule. The metadata includes the runtime system in use, which accounts have
* debug logging enabled, and other custom rule metadata, such as resource type, resource
* ID of Amazon Web Services resource, and organization trigger types that initiate Config to evaluate Amazon Web Services resources against a rule.
*/
val organizationCustomPolicyRuleMetadata: aws.sdk.kotlin.services.configservice.model.OrganizationCustomPolicyRuleMetadataNoPolicy? = builder.organizationCustomPolicyRuleMetadata
/**
* An OrganizationCustomRuleMetadata object.
*/
val organizationCustomRuleMetadata: aws.sdk.kotlin.services.configservice.model.OrganizationCustomRuleMetadata? = builder.organizationCustomRuleMetadata
/**
* An OrganizationManagedRuleMetadata object.
*/
val organizationManagedRuleMetadata: aws.sdk.kotlin.services.configservice.model.OrganizationManagedRuleMetadata? = builder.organizationManagedRuleMetadata
companion object {
operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.configservice.model.OrganizationConfigRule = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("OrganizationConfigRule(")
append("excludedAccounts=$excludedAccounts,")
append("lastUpdateTime=$lastUpdateTime,")
append("organizationConfigRuleArn=$organizationConfigRuleArn,")
append("organizationConfigRuleName=$organizationConfigRuleName,")
append("organizationCustomPolicyRuleMetadata=$organizationCustomPolicyRuleMetadata,")
append("organizationCustomRuleMetadata=$organizationCustomRuleMetadata,")
append("organizationManagedRuleMetadata=$organizationManagedRuleMetadata)")
}
override fun hashCode(): kotlin.Int {
var result = excludedAccounts?.hashCode() ?: 0
result = 31 * result + (lastUpdateTime?.hashCode() ?: 0)
result = 31 * result + (organizationConfigRuleArn?.hashCode() ?: 0)
result = 31 * result + (organizationConfigRuleName?.hashCode() ?: 0)
result = 31 * result + (organizationCustomPolicyRuleMetadata?.hashCode() ?: 0)
result = 31 * result + (organizationCustomRuleMetadata?.hashCode() ?: 0)
result = 31 * result + (organizationManagedRuleMetadata?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as OrganizationConfigRule
if (excludedAccounts != other.excludedAccounts) return false
if (lastUpdateTime != other.lastUpdateTime) return false
if (organizationConfigRuleArn != other.organizationConfigRuleArn) return false
if (organizationConfigRuleName != other.organizationConfigRuleName) return false
if (organizationCustomPolicyRuleMetadata != other.organizationCustomPolicyRuleMetadata) return false
if (organizationCustomRuleMetadata != other.organizationCustomRuleMetadata) return false
if (organizationManagedRuleMetadata != other.organizationManagedRuleMetadata) return false
return true
}
inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.configservice.model.OrganizationConfigRule = Builder(this).apply(block).build()
class Builder {
/**
* A comma-separated list of accounts excluded from organization Config rule.
*/
var excludedAccounts: List? = null
/**
* The timestamp of the last update.
*/
var lastUpdateTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* Amazon Resource Name (ARN) of organization Config rule.
*/
var organizationConfigRuleArn: kotlin.String? = null
/**
* The name that you assign to organization Config rule.
*/
var organizationConfigRuleName: kotlin.String? = null
/**
* An
* object that specifies metadata for your organization's Config Custom Policy rule. The metadata includes the runtime system in use, which accounts have
* debug logging enabled, and other custom rule metadata, such as resource type, resource
* ID of Amazon Web Services resource, and organization trigger types that initiate Config to evaluate Amazon Web Services resources against a rule.
*/
var organizationCustomPolicyRuleMetadata: aws.sdk.kotlin.services.configservice.model.OrganizationCustomPolicyRuleMetadataNoPolicy? = null
/**
* An OrganizationCustomRuleMetadata object.
*/
var organizationCustomRuleMetadata: aws.sdk.kotlin.services.configservice.model.OrganizationCustomRuleMetadata? = null
/**
* An OrganizationManagedRuleMetadata object.
*/
var organizationManagedRuleMetadata: aws.sdk.kotlin.services.configservice.model.OrganizationManagedRuleMetadata? = null
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.configservice.model.OrganizationConfigRule) : this() {
this.excludedAccounts = x.excludedAccounts
this.lastUpdateTime = x.lastUpdateTime
this.organizationConfigRuleArn = x.organizationConfigRuleArn
this.organizationConfigRuleName = x.organizationConfigRuleName
this.organizationCustomPolicyRuleMetadata = x.organizationCustomPolicyRuleMetadata
this.organizationCustomRuleMetadata = x.organizationCustomRuleMetadata
this.organizationManagedRuleMetadata = x.organizationManagedRuleMetadata
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.configservice.model.OrganizationConfigRule = OrganizationConfigRule(this)
/**
* construct an [aws.sdk.kotlin.services.configservice.model.OrganizationCustomPolicyRuleMetadataNoPolicy] inside the given [block]
*/
fun organizationCustomPolicyRuleMetadata(block: aws.sdk.kotlin.services.configservice.model.OrganizationCustomPolicyRuleMetadataNoPolicy.Builder.() -> kotlin.Unit) {
this.organizationCustomPolicyRuleMetadata = aws.sdk.kotlin.services.configservice.model.OrganizationCustomPolicyRuleMetadataNoPolicy.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.configservice.model.OrganizationCustomRuleMetadata] inside the given [block]
*/
fun organizationCustomRuleMetadata(block: aws.sdk.kotlin.services.configservice.model.OrganizationCustomRuleMetadata.Builder.() -> kotlin.Unit) {
this.organizationCustomRuleMetadata = aws.sdk.kotlin.services.configservice.model.OrganizationCustomRuleMetadata.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.configservice.model.OrganizationManagedRuleMetadata] inside the given [block]
*/
fun organizationManagedRuleMetadata(block: aws.sdk.kotlin.services.configservice.model.OrganizationManagedRuleMetadata.Builder.() -> kotlin.Unit) {
this.organizationManagedRuleMetadata = aws.sdk.kotlin.services.configservice.model.OrganizationManagedRuleMetadata.invoke(block)
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy