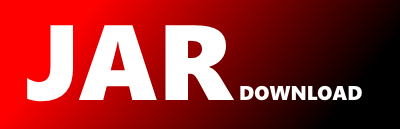
commonMain.aws.sdk.kotlin.services.connect.model.Instance.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.connect.model
import aws.smithy.kotlin.runtime.time.Instant
/**
* The Amazon Connect instance.
*/
public class Instance private constructor(builder: Builder) {
/**
* The Amazon Resource Name (ARN) of the instance.
*/
public val arn: kotlin.String? = builder.arn
/**
* When the instance was created.
*/
public val createdTime: aws.smithy.kotlin.runtime.time.Instant? = builder.createdTime
/**
* The identifier of the Amazon Connect instance. You can [find the instance ID](https://docs.aws.amazon.com/connect/latest/adminguide/find-instance-arn.html) in the Amazon Resource Name (ARN) of the instance.
*/
public val id: kotlin.String? = builder.id
/**
* The identity management type.
*/
public val identityManagementType: aws.sdk.kotlin.services.connect.model.DirectoryType? = builder.identityManagementType
/**
* Whether inbound calls are enabled.
*/
public val inboundCallsEnabled: kotlin.Boolean? = builder.inboundCallsEnabled
/**
* The alias of instance.
*/
public val instanceAlias: kotlin.String? = builder.instanceAlias
/**
* The state of the instance.
*/
public val instanceStatus: aws.sdk.kotlin.services.connect.model.InstanceStatus? = builder.instanceStatus
/**
* Whether outbound calls are enabled.
*/
public val outboundCallsEnabled: kotlin.Boolean? = builder.outboundCallsEnabled
/**
* The service role of the instance.
*/
public val serviceRole: kotlin.String? = builder.serviceRole
/**
* Relevant details why the instance was not successfully created.
*/
public val statusReason: aws.sdk.kotlin.services.connect.model.InstanceStatusReason? = builder.statusReason
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.connect.model.Instance = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("Instance(")
append("arn=$arn,")
append("createdTime=$createdTime,")
append("id=$id,")
append("identityManagementType=$identityManagementType,")
append("inboundCallsEnabled=$inboundCallsEnabled,")
append("instanceAlias=*** Sensitive Data Redacted ***,")
append("instanceStatus=$instanceStatus,")
append("outboundCallsEnabled=$outboundCallsEnabled,")
append("serviceRole=$serviceRole,")
append("statusReason=$statusReason")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = arn?.hashCode() ?: 0
result = 31 * result + (createdTime?.hashCode() ?: 0)
result = 31 * result + (id?.hashCode() ?: 0)
result = 31 * result + (identityManagementType?.hashCode() ?: 0)
result = 31 * result + (inboundCallsEnabled?.hashCode() ?: 0)
result = 31 * result + (instanceAlias?.hashCode() ?: 0)
result = 31 * result + (instanceStatus?.hashCode() ?: 0)
result = 31 * result + (outboundCallsEnabled?.hashCode() ?: 0)
result = 31 * result + (serviceRole?.hashCode() ?: 0)
result = 31 * result + (statusReason?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as Instance
if (arn != other.arn) return false
if (createdTime != other.createdTime) return false
if (id != other.id) return false
if (identityManagementType != other.identityManagementType) return false
if (inboundCallsEnabled != other.inboundCallsEnabled) return false
if (instanceAlias != other.instanceAlias) return false
if (instanceStatus != other.instanceStatus) return false
if (outboundCallsEnabled != other.outboundCallsEnabled) return false
if (serviceRole != other.serviceRole) return false
if (statusReason != other.statusReason) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.connect.model.Instance = Builder(this).apply(block).build()
public class Builder {
/**
* The Amazon Resource Name (ARN) of the instance.
*/
public var arn: kotlin.String? = null
/**
* When the instance was created.
*/
public var createdTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The identifier of the Amazon Connect instance. You can [find the instance ID](https://docs.aws.amazon.com/connect/latest/adminguide/find-instance-arn.html) in the Amazon Resource Name (ARN) of the instance.
*/
public var id: kotlin.String? = null
/**
* The identity management type.
*/
public var identityManagementType: aws.sdk.kotlin.services.connect.model.DirectoryType? = null
/**
* Whether inbound calls are enabled.
*/
public var inboundCallsEnabled: kotlin.Boolean? = null
/**
* The alias of instance.
*/
public var instanceAlias: kotlin.String? = null
/**
* The state of the instance.
*/
public var instanceStatus: aws.sdk.kotlin.services.connect.model.InstanceStatus? = null
/**
* Whether outbound calls are enabled.
*/
public var outboundCallsEnabled: kotlin.Boolean? = null
/**
* The service role of the instance.
*/
public var serviceRole: kotlin.String? = null
/**
* Relevant details why the instance was not successfully created.
*/
public var statusReason: aws.sdk.kotlin.services.connect.model.InstanceStatusReason? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.connect.model.Instance) : this() {
this.arn = x.arn
this.createdTime = x.createdTime
this.id = x.id
this.identityManagementType = x.identityManagementType
this.inboundCallsEnabled = x.inboundCallsEnabled
this.instanceAlias = x.instanceAlias
this.instanceStatus = x.instanceStatus
this.outboundCallsEnabled = x.outboundCallsEnabled
this.serviceRole = x.serviceRole
this.statusReason = x.statusReason
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.connect.model.Instance = Instance(this)
/**
* construct an [aws.sdk.kotlin.services.connect.model.InstanceStatusReason] inside the given [block]
*/
public fun statusReason(block: aws.sdk.kotlin.services.connect.model.InstanceStatusReason.Builder.() -> kotlin.Unit) {
this.statusReason = aws.sdk.kotlin.services.connect.model.InstanceStatusReason.invoke(block)
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy