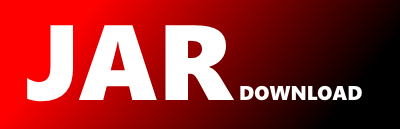
commonMain.aws.sdk.kotlin.services.connect.model.CreateVocabularyRequest.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.connect.model
public class CreateVocabularyRequest private constructor(builder: Builder) {
/**
* A unique, case-sensitive identifier that you provide to ensure the idempotency of the request. If not provided, the Amazon Web Services SDK populates this field. For more information about idempotency, see [Making retries safe with idempotent APIs](https://aws.amazon.com/builders-library/making-retries-safe-with-idempotent-APIs/). If a create request is received more than once with same client token, subsequent requests return the previous response without creating a vocabulary again.
*/
public val clientToken: kotlin.String? = builder.clientToken
/**
* The content of the custom vocabulary in plain-text format with a table of values. Each row in the table represents a word or a phrase, described with `Phrase`, `IPA`, `SoundsLike`, and `DisplayAs` fields. Separate the fields with TAB characters. The size limit is 50KB. For more information, see [Create a custom vocabulary using a table](https://docs.aws.amazon.com/transcribe/latest/dg/custom-vocabulary.html#create-vocabulary-table).
*/
public val content: kotlin.String? = builder.content
/**
* The identifier of the Amazon Connect instance. You can [find the instance ID](https://docs.aws.amazon.com/connect/latest/adminguide/find-instance-arn.html) in the Amazon Resource Name (ARN) of the instance.
*/
public val instanceId: kotlin.String? = requireNotNull(builder.instanceId) { "A non-null value must be provided for instanceId" }
/**
* The language code of the vocabulary entries. For a list of languages and their corresponding language codes, see [What is Amazon Transcribe?](https://docs.aws.amazon.com/transcribe/latest/dg/transcribe-whatis.html)
*/
public val languageCode: aws.sdk.kotlin.services.connect.model.VocabularyLanguageCode? = builder.languageCode
/**
* The tags used to organize, track, or control access for this resource. For example, { "tags": {"key1":"value1", "key2":"value2"} }.
*/
public val tags: Map? = builder.tags
/**
* A unique name of the custom vocabulary.
*/
public val vocabularyName: kotlin.String? = builder.vocabularyName
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.connect.model.CreateVocabularyRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("CreateVocabularyRequest(")
append("clientToken=$clientToken,")
append("content=$content,")
append("instanceId=$instanceId,")
append("languageCode=$languageCode,")
append("tags=$tags,")
append("vocabularyName=$vocabularyName")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = clientToken?.hashCode() ?: 0
result = 31 * result + (content?.hashCode() ?: 0)
result = 31 * result + (instanceId?.hashCode() ?: 0)
result = 31 * result + (languageCode?.hashCode() ?: 0)
result = 31 * result + (tags?.hashCode() ?: 0)
result = 31 * result + (vocabularyName?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as CreateVocabularyRequest
if (clientToken != other.clientToken) return false
if (content != other.content) return false
if (instanceId != other.instanceId) return false
if (languageCode != other.languageCode) return false
if (tags != other.tags) return false
if (vocabularyName != other.vocabularyName) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.connect.model.CreateVocabularyRequest = Builder(this).apply(block).build()
public class Builder {
/**
* A unique, case-sensitive identifier that you provide to ensure the idempotency of the request. If not provided, the Amazon Web Services SDK populates this field. For more information about idempotency, see [Making retries safe with idempotent APIs](https://aws.amazon.com/builders-library/making-retries-safe-with-idempotent-APIs/). If a create request is received more than once with same client token, subsequent requests return the previous response without creating a vocabulary again.
*/
public var clientToken: kotlin.String? = null
/**
* The content of the custom vocabulary in plain-text format with a table of values. Each row in the table represents a word or a phrase, described with `Phrase`, `IPA`, `SoundsLike`, and `DisplayAs` fields. Separate the fields with TAB characters. The size limit is 50KB. For more information, see [Create a custom vocabulary using a table](https://docs.aws.amazon.com/transcribe/latest/dg/custom-vocabulary.html#create-vocabulary-table).
*/
public var content: kotlin.String? = null
/**
* The identifier of the Amazon Connect instance. You can [find the instance ID](https://docs.aws.amazon.com/connect/latest/adminguide/find-instance-arn.html) in the Amazon Resource Name (ARN) of the instance.
*/
public var instanceId: kotlin.String? = null
/**
* The language code of the vocabulary entries. For a list of languages and their corresponding language codes, see [What is Amazon Transcribe?](https://docs.aws.amazon.com/transcribe/latest/dg/transcribe-whatis.html)
*/
public var languageCode: aws.sdk.kotlin.services.connect.model.VocabularyLanguageCode? = null
/**
* The tags used to organize, track, or control access for this resource. For example, { "tags": {"key1":"value1", "key2":"value2"} }.
*/
public var tags: Map? = null
/**
* A unique name of the custom vocabulary.
*/
public var vocabularyName: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.connect.model.CreateVocabularyRequest) : this() {
this.clientToken = x.clientToken
this.content = x.content
this.instanceId = x.instanceId
this.languageCode = x.languageCode
this.tags = x.tags
this.vocabularyName = x.vocabularyName
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.connect.model.CreateVocabularyRequest = CreateVocabularyRequest(this)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy