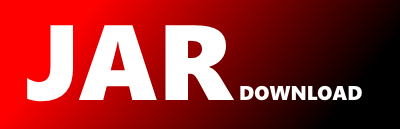
commonMain.aws.sdk.kotlin.services.customerprofiles.model.UpdateAddress.kt Maven / Gradle / Ivy
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.customerprofiles.model
/**
* Updates associated with the address properties of a customer profile.
*/
public class UpdateAddress private constructor(builder: Builder) {
/**
* The first line of a customer address.
*/
public val address1: kotlin.String? = builder.address1
/**
* The second line of a customer address.
*/
public val address2: kotlin.String? = builder.address2
/**
* The third line of a customer address.
*/
public val address3: kotlin.String? = builder.address3
/**
* The fourth line of a customer address.
*/
public val address4: kotlin.String? = builder.address4
/**
* The city in which a customer lives.
*/
public val city: kotlin.String? = builder.city
/**
* The country in which a customer lives.
*/
public val country: kotlin.String? = builder.country
/**
* The county in which a customer lives.
*/
public val county: kotlin.String? = builder.county
/**
* The postal code of a customer address.
*/
public val postalCode: kotlin.String? = builder.postalCode
/**
* The province in which a customer lives.
*/
public val province: kotlin.String? = builder.province
/**
* The state in which a customer lives.
*/
public val state: kotlin.String? = builder.state
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.customerprofiles.model.UpdateAddress = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("UpdateAddress(")
append("address1=$address1,")
append("address2=$address2,")
append("address3=$address3,")
append("address4=$address4,")
append("city=$city,")
append("country=$country,")
append("county=$county,")
append("postalCode=$postalCode,")
append("province=$province,")
append("state=$state")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = address1?.hashCode() ?: 0
result = 31 * result + (address2?.hashCode() ?: 0)
result = 31 * result + (address3?.hashCode() ?: 0)
result = 31 * result + (address4?.hashCode() ?: 0)
result = 31 * result + (city?.hashCode() ?: 0)
result = 31 * result + (country?.hashCode() ?: 0)
result = 31 * result + (county?.hashCode() ?: 0)
result = 31 * result + (postalCode?.hashCode() ?: 0)
result = 31 * result + (province?.hashCode() ?: 0)
result = 31 * result + (state?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as UpdateAddress
if (address1 != other.address1) return false
if (address2 != other.address2) return false
if (address3 != other.address3) return false
if (address4 != other.address4) return false
if (city != other.city) return false
if (country != other.country) return false
if (county != other.county) return false
if (postalCode != other.postalCode) return false
if (province != other.province) return false
if (state != other.state) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.customerprofiles.model.UpdateAddress = Builder(this).apply(block).build()
public class Builder {
/**
* The first line of a customer address.
*/
public var address1: kotlin.String? = null
/**
* The second line of a customer address.
*/
public var address2: kotlin.String? = null
/**
* The third line of a customer address.
*/
public var address3: kotlin.String? = null
/**
* The fourth line of a customer address.
*/
public var address4: kotlin.String? = null
/**
* The city in which a customer lives.
*/
public var city: kotlin.String? = null
/**
* The country in which a customer lives.
*/
public var country: kotlin.String? = null
/**
* The county in which a customer lives.
*/
public var county: kotlin.String? = null
/**
* The postal code of a customer address.
*/
public var postalCode: kotlin.String? = null
/**
* The province in which a customer lives.
*/
public var province: kotlin.String? = null
/**
* The state in which a customer lives.
*/
public var state: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.customerprofiles.model.UpdateAddress) : this() {
this.address1 = x.address1
this.address2 = x.address2
this.address3 = x.address3
this.address4 = x.address4
this.city = x.city
this.country = x.country
this.county = x.county
this.postalCode = x.postalCode
this.province = x.province
this.state = x.state
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.customerprofiles.model.UpdateAddress = UpdateAddress(this)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy