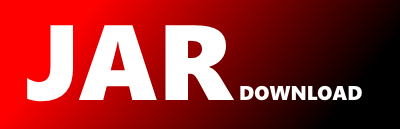
commonMain.aws.sdk.kotlin.services.databasemigrationservice.model.Certificate.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of databasemigrationservice-jvm Show documentation
Show all versions of databasemigrationservice-jvm Show documentation
The AWS SDK for Kotlin client for Database Migration Service
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.databasemigrationservice.model
import aws.smithy.kotlin.runtime.SdkDsl
import aws.smithy.kotlin.runtime.time.Instant
/**
* The SSL certificate that can be used to encrypt connections between the endpoints and the replication instance.
*/
public class Certificate private constructor(builder: Builder) {
/**
* The Amazon Resource Name (ARN) for the certificate.
*/
public val certificateArn: kotlin.String? = builder.certificateArn
/**
* The date that the certificate was created.
*/
public val certificateCreationDate: aws.smithy.kotlin.runtime.time.Instant? = builder.certificateCreationDate
/**
* A customer-assigned name for the certificate. Identifiers must begin with a letter and must contain only ASCII letters, digits, and hyphens. They can't end with a hyphen or contain two consecutive hyphens.
*/
public val certificateIdentifier: kotlin.String? = builder.certificateIdentifier
/**
* The owner of the certificate.
*/
public val certificateOwner: kotlin.String? = builder.certificateOwner
/**
* The contents of a `.pem` file, which contains an X.509 certificate.
*/
public val certificatePem: kotlin.String? = builder.certificatePem
/**
* The location of an imported Oracle Wallet certificate for use with SSL. Example: `filebase64("${path.root}/rds-ca-2019-root.sso")`
*/
public val certificateWallet: kotlin.ByteArray? = builder.certificateWallet
/**
* The key length of the cryptographic algorithm being used.
*/
public val keyLength: kotlin.Int? = builder.keyLength
/**
* The signing algorithm for the certificate.
*/
public val signingAlgorithm: kotlin.String? = builder.signingAlgorithm
/**
* The beginning date that the certificate is valid.
*/
public val validFromDate: aws.smithy.kotlin.runtime.time.Instant? = builder.validFromDate
/**
* The final date that the certificate is valid.
*/
public val validToDate: aws.smithy.kotlin.runtime.time.Instant? = builder.validToDate
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.databasemigrationservice.model.Certificate = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("Certificate(")
append("certificateArn=$certificateArn,")
append("certificateCreationDate=$certificateCreationDate,")
append("certificateIdentifier=$certificateIdentifier,")
append("certificateOwner=$certificateOwner,")
append("certificatePem=$certificatePem,")
append("certificateWallet=$certificateWallet,")
append("keyLength=$keyLength,")
append("signingAlgorithm=$signingAlgorithm,")
append("validFromDate=$validFromDate,")
append("validToDate=$validToDate")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = certificateArn?.hashCode() ?: 0
result = 31 * result + (certificateCreationDate?.hashCode() ?: 0)
result = 31 * result + (certificateIdentifier?.hashCode() ?: 0)
result = 31 * result + (certificateOwner?.hashCode() ?: 0)
result = 31 * result + (certificatePem?.hashCode() ?: 0)
result = 31 * result + (certificateWallet?.contentHashCode() ?: 0)
result = 31 * result + (keyLength ?: 0)
result = 31 * result + (signingAlgorithm?.hashCode() ?: 0)
result = 31 * result + (validFromDate?.hashCode() ?: 0)
result = 31 * result + (validToDate?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as Certificate
if (certificateArn != other.certificateArn) return false
if (certificateCreationDate != other.certificateCreationDate) return false
if (certificateIdentifier != other.certificateIdentifier) return false
if (certificateOwner != other.certificateOwner) return false
if (certificatePem != other.certificatePem) return false
if (certificateWallet != null) {
if (other.certificateWallet == null) return false
if (!certificateWallet.contentEquals(other.certificateWallet)) return false
} else if (other.certificateWallet != null) return false
if (keyLength != other.keyLength) return false
if (signingAlgorithm != other.signingAlgorithm) return false
if (validFromDate != other.validFromDate) return false
if (validToDate != other.validToDate) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.databasemigrationservice.model.Certificate = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The Amazon Resource Name (ARN) for the certificate.
*/
public var certificateArn: kotlin.String? = null
/**
* The date that the certificate was created.
*/
public var certificateCreationDate: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* A customer-assigned name for the certificate. Identifiers must begin with a letter and must contain only ASCII letters, digits, and hyphens. They can't end with a hyphen or contain two consecutive hyphens.
*/
public var certificateIdentifier: kotlin.String? = null
/**
* The owner of the certificate.
*/
public var certificateOwner: kotlin.String? = null
/**
* The contents of a `.pem` file, which contains an X.509 certificate.
*/
public var certificatePem: kotlin.String? = null
/**
* The location of an imported Oracle Wallet certificate for use with SSL. Example: `filebase64("${path.root}/rds-ca-2019-root.sso")`
*/
public var certificateWallet: kotlin.ByteArray? = null
/**
* The key length of the cryptographic algorithm being used.
*/
public var keyLength: kotlin.Int? = null
/**
* The signing algorithm for the certificate.
*/
public var signingAlgorithm: kotlin.String? = null
/**
* The beginning date that the certificate is valid.
*/
public var validFromDate: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The final date that the certificate is valid.
*/
public var validToDate: aws.smithy.kotlin.runtime.time.Instant? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.databasemigrationservice.model.Certificate) : this() {
this.certificateArn = x.certificateArn
this.certificateCreationDate = x.certificateCreationDate
this.certificateIdentifier = x.certificateIdentifier
this.certificateOwner = x.certificateOwner
this.certificatePem = x.certificatePem
this.certificateWallet = x.certificateWallet
this.keyLength = x.keyLength
this.signingAlgorithm = x.signingAlgorithm
this.validFromDate = x.validFromDate
this.validToDate = x.validToDate
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.databasemigrationservice.model.Certificate = Certificate(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy