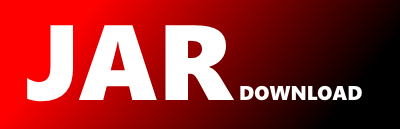
commonMain.aws.sdk.kotlin.services.databasemigrationservice.model.CollectorResponse.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of databasemigrationservice-jvm Show documentation
Show all versions of databasemigrationservice-jvm Show documentation
The AWS SDK for Kotlin client for Database Migration Service
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.databasemigrationservice.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Describes a Fleet Advisor collector.
*/
public class CollectorResponse private constructor(builder: Builder) {
/**
* Describes the last Fleet Advisor collector health check.
*/
public val collectorHealthCheck: aws.sdk.kotlin.services.databasemigrationservice.model.CollectorHealthCheck? = builder.collectorHealthCheck
/**
* The name of the Fleet Advisor collector .
*/
public val collectorName: kotlin.String? = builder.collectorName
/**
* The reference ID of the Fleet Advisor collector.
*/
public val collectorReferencedId: kotlin.String? = builder.collectorReferencedId
/**
* The version of your Fleet Advisor collector, in semantic versioning format, for example `1.0.2`
*/
public val collectorVersion: kotlin.String? = builder.collectorVersion
/**
* The timestamp when you created the collector, in the following format: `2022-01-24T19:04:02.596113Z`
*/
public val createdDate: kotlin.String? = builder.createdDate
/**
* A summary description of the Fleet Advisor collector.
*/
public val description: kotlin.String? = builder.description
/**
* Describes a Fleet Advisor collector inventory.
*/
public val inventoryData: aws.sdk.kotlin.services.databasemigrationservice.model.InventoryData? = builder.inventoryData
/**
* The timestamp of the last time the collector received data, in the following format: `2022-01-24T19:04:02.596113Z`
*/
public val lastDataReceived: kotlin.String? = builder.lastDataReceived
/**
* The timestamp when DMS last modified the collector, in the following format: `2022-01-24T19:04:02.596113Z`
*/
public val modifiedDate: kotlin.String? = builder.modifiedDate
/**
* The timestamp when DMS registered the collector, in the following format: `2022-01-24T19:04:02.596113Z`
*/
public val registeredDate: kotlin.String? = builder.registeredDate
/**
* The Amazon S3 bucket that the Fleet Advisor collector uses to store inventory metadata.
*/
public val s3BucketName: kotlin.String? = builder.s3BucketName
/**
* The IAM role that grants permissions to access the specified Amazon S3 bucket.
*/
public val serviceAccessRoleArn: kotlin.String? = builder.serviceAccessRoleArn
/**
* Whether the collector version is up to date.
*/
public val versionStatus: aws.sdk.kotlin.services.databasemigrationservice.model.VersionStatus? = builder.versionStatus
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.databasemigrationservice.model.CollectorResponse = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("CollectorResponse(")
append("collectorHealthCheck=$collectorHealthCheck,")
append("collectorName=$collectorName,")
append("collectorReferencedId=$collectorReferencedId,")
append("collectorVersion=$collectorVersion,")
append("createdDate=$createdDate,")
append("description=$description,")
append("inventoryData=$inventoryData,")
append("lastDataReceived=$lastDataReceived,")
append("modifiedDate=$modifiedDate,")
append("registeredDate=$registeredDate,")
append("s3BucketName=$s3BucketName,")
append("serviceAccessRoleArn=$serviceAccessRoleArn,")
append("versionStatus=$versionStatus")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = collectorHealthCheck?.hashCode() ?: 0
result = 31 * result + (collectorName?.hashCode() ?: 0)
result = 31 * result + (collectorReferencedId?.hashCode() ?: 0)
result = 31 * result + (collectorVersion?.hashCode() ?: 0)
result = 31 * result + (createdDate?.hashCode() ?: 0)
result = 31 * result + (description?.hashCode() ?: 0)
result = 31 * result + (inventoryData?.hashCode() ?: 0)
result = 31 * result + (lastDataReceived?.hashCode() ?: 0)
result = 31 * result + (modifiedDate?.hashCode() ?: 0)
result = 31 * result + (registeredDate?.hashCode() ?: 0)
result = 31 * result + (s3BucketName?.hashCode() ?: 0)
result = 31 * result + (serviceAccessRoleArn?.hashCode() ?: 0)
result = 31 * result + (versionStatus?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as CollectorResponse
if (collectorHealthCheck != other.collectorHealthCheck) return false
if (collectorName != other.collectorName) return false
if (collectorReferencedId != other.collectorReferencedId) return false
if (collectorVersion != other.collectorVersion) return false
if (createdDate != other.createdDate) return false
if (description != other.description) return false
if (inventoryData != other.inventoryData) return false
if (lastDataReceived != other.lastDataReceived) return false
if (modifiedDate != other.modifiedDate) return false
if (registeredDate != other.registeredDate) return false
if (s3BucketName != other.s3BucketName) return false
if (serviceAccessRoleArn != other.serviceAccessRoleArn) return false
if (versionStatus != other.versionStatus) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.databasemigrationservice.model.CollectorResponse = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* Describes the last Fleet Advisor collector health check.
*/
public var collectorHealthCheck: aws.sdk.kotlin.services.databasemigrationservice.model.CollectorHealthCheck? = null
/**
* The name of the Fleet Advisor collector .
*/
public var collectorName: kotlin.String? = null
/**
* The reference ID of the Fleet Advisor collector.
*/
public var collectorReferencedId: kotlin.String? = null
/**
* The version of your Fleet Advisor collector, in semantic versioning format, for example `1.0.2`
*/
public var collectorVersion: kotlin.String? = null
/**
* The timestamp when you created the collector, in the following format: `2022-01-24T19:04:02.596113Z`
*/
public var createdDate: kotlin.String? = null
/**
* A summary description of the Fleet Advisor collector.
*/
public var description: kotlin.String? = null
/**
* Describes a Fleet Advisor collector inventory.
*/
public var inventoryData: aws.sdk.kotlin.services.databasemigrationservice.model.InventoryData? = null
/**
* The timestamp of the last time the collector received data, in the following format: `2022-01-24T19:04:02.596113Z`
*/
public var lastDataReceived: kotlin.String? = null
/**
* The timestamp when DMS last modified the collector, in the following format: `2022-01-24T19:04:02.596113Z`
*/
public var modifiedDate: kotlin.String? = null
/**
* The timestamp when DMS registered the collector, in the following format: `2022-01-24T19:04:02.596113Z`
*/
public var registeredDate: kotlin.String? = null
/**
* The Amazon S3 bucket that the Fleet Advisor collector uses to store inventory metadata.
*/
public var s3BucketName: kotlin.String? = null
/**
* The IAM role that grants permissions to access the specified Amazon S3 bucket.
*/
public var serviceAccessRoleArn: kotlin.String? = null
/**
* Whether the collector version is up to date.
*/
public var versionStatus: aws.sdk.kotlin.services.databasemigrationservice.model.VersionStatus? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.databasemigrationservice.model.CollectorResponse) : this() {
this.collectorHealthCheck = x.collectorHealthCheck
this.collectorName = x.collectorName
this.collectorReferencedId = x.collectorReferencedId
this.collectorVersion = x.collectorVersion
this.createdDate = x.createdDate
this.description = x.description
this.inventoryData = x.inventoryData
this.lastDataReceived = x.lastDataReceived
this.modifiedDate = x.modifiedDate
this.registeredDate = x.registeredDate
this.s3BucketName = x.s3BucketName
this.serviceAccessRoleArn = x.serviceAccessRoleArn
this.versionStatus = x.versionStatus
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.databasemigrationservice.model.CollectorResponse = CollectorResponse(this)
/**
* construct an [aws.sdk.kotlin.services.databasemigrationservice.model.CollectorHealthCheck] inside the given [block]
*/
public fun collectorHealthCheck(block: aws.sdk.kotlin.services.databasemigrationservice.model.CollectorHealthCheck.Builder.() -> kotlin.Unit) {
this.collectorHealthCheck = aws.sdk.kotlin.services.databasemigrationservice.model.CollectorHealthCheck.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.databasemigrationservice.model.InventoryData] inside the given [block]
*/
public fun inventoryData(block: aws.sdk.kotlin.services.databasemigrationservice.model.InventoryData.Builder.() -> kotlin.Unit) {
this.inventoryData = aws.sdk.kotlin.services.databasemigrationservice.model.InventoryData.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy