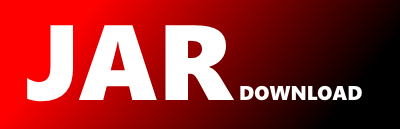
commonMain.aws.sdk.kotlin.services.databasemigrationservice.model.CreateEndpointRequest.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of databasemigrationservice-jvm Show documentation
Show all versions of databasemigrationservice-jvm Show documentation
The AWS SDK for Kotlin client for Database Migration Service
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.databasemigrationservice.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
*
*/
public class CreateEndpointRequest private constructor(builder: Builder) {
/**
* The Amazon Resource Name (ARN) for the certificate.
*/
public val certificateArn: kotlin.String? = builder.certificateArn
/**
* The name of the endpoint database. For a MySQL source or target endpoint, do not specify DatabaseName. To migrate to a specific database, use this setting and `targetDbType`.
*/
public val databaseName: kotlin.String? = builder.databaseName
/**
* The settings in JSON format for the DMS transfer type of source endpoint.
*
* Possible settings include the following:
* + `ServiceAccessRoleArn` - The Amazon Resource Name (ARN) used by the service access IAM role. The role must allow the `iam:PassRole` action.
* + `BucketName` - The name of the S3 bucket to use.
*
* Shorthand syntax for these settings is as follows: `ServiceAccessRoleArn=string,BucketName=string`
*
* JSON syntax for these settings is as follows: `{ "ServiceAccessRoleArn": "string", "BucketName": "string", } `
*/
public val dmsTransferSettings: aws.sdk.kotlin.services.databasemigrationservice.model.DmsTransferSettings? = builder.dmsTransferSettings
/**
* Provides information that defines a DocumentDB endpoint.
*/
public val docDbSettings: aws.sdk.kotlin.services.databasemigrationservice.model.DocDbSettings? = builder.docDbSettings
/**
* Settings in JSON format for the target Amazon DynamoDB endpoint. For information about other available settings, see [Using Object Mapping to Migrate Data to DynamoDB](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Target.DynamoDB.html#CHAP_Target.DynamoDB.ObjectMapping) in the *Database Migration Service User Guide.*
*/
public val dynamoDbSettings: aws.sdk.kotlin.services.databasemigrationservice.model.DynamoDbSettings? = builder.dynamoDbSettings
/**
* Settings in JSON format for the target OpenSearch endpoint. For more information about the available settings, see [Extra Connection Attributes When Using OpenSearch as a Target for DMS](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Target.Elasticsearch.html#CHAP_Target.Elasticsearch.Configuration) in the *Database Migration Service User Guide*.
*/
public val elasticsearchSettings: aws.sdk.kotlin.services.databasemigrationservice.model.ElasticsearchSettings? = builder.elasticsearchSettings
/**
* The database endpoint identifier. Identifiers must begin with a letter and must contain only ASCII letters, digits, and hyphens. They can't end with a hyphen, or contain two consecutive hyphens.
*/
public val endpointIdentifier: kotlin.String? = builder.endpointIdentifier
/**
* The type of endpoint. Valid values are `source` and `target`.
*/
public val endpointType: aws.sdk.kotlin.services.databasemigrationservice.model.ReplicationEndpointTypeValue? = builder.endpointType
/**
* The type of engine for the endpoint. Valid values, depending on the `EndpointType` value, include `"mysql"`, `"oracle"`, `"postgres"`, `"mariadb"`, `"aurora"`, `"aurora-postgresql"`, `"opensearch"`, `"redshift"`, `"s3"`, `"db2"`, `"db2-zos"`, `"azuredb"`, `"sybase"`, `"dynamodb"`, `"mongodb"`, `"kinesis"`, `"kafka"`, `"elasticsearch"`, `"docdb"`, `"sqlserver"`, `"neptune"`, `"babelfish"`, `redshift-serverless`, `aurora-serverless`, `aurora-postgresql-serverless`, `gcp-mysql`, `azure-sql-managed-instance`, `redis`, `dms-transfer`.
*/
public val engineName: kotlin.String? = builder.engineName
/**
* The external table definition.
*/
public val externalTableDefinition: kotlin.String? = builder.externalTableDefinition
/**
* Additional attributes associated with the connection. Each attribute is specified as a name-value pair associated by an equal sign (=). Multiple attributes are separated by a semicolon (;) with no additional white space. For information on the attributes available for connecting your source or target endpoint, see [Working with DMS Endpoints](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Endpoints.html) in the *Database Migration Service User Guide.*
*/
public val extraConnectionAttributes: kotlin.String? = builder.extraConnectionAttributes
/**
* Settings in JSON format for the source GCP MySQL endpoint.
*/
public val gcpMySqlSettings: aws.sdk.kotlin.services.databasemigrationservice.model.GcpMySqlSettings? = builder.gcpMySqlSettings
/**
* Settings in JSON format for the source IBM Db2 LUW endpoint. For information about other available settings, see [Extra connection attributes when using Db2 LUW as a source for DMS](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Source.DB2.html#CHAP_Source.DB2.ConnectionAttrib) in the *Database Migration Service User Guide.*
*/
public val ibmDb2Settings: aws.sdk.kotlin.services.databasemigrationservice.model.IbmDb2Settings? = builder.ibmDb2Settings
/**
* Settings in JSON format for the target Apache Kafka endpoint. For more information about the available settings, see [Using object mapping to migrate data to a Kafka topic](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Target.Kafka.html#CHAP_Target.Kafka.ObjectMapping) in the *Database Migration Service User Guide.*
*/
public val kafkaSettings: aws.sdk.kotlin.services.databasemigrationservice.model.KafkaSettings? = builder.kafkaSettings
/**
* Settings in JSON format for the target endpoint for Amazon Kinesis Data Streams. For more information about the available settings, see [Using object mapping to migrate data to a Kinesis data stream](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Target.Kinesis.html#CHAP_Target.Kinesis.ObjectMapping) in the *Database Migration Service User Guide.*
*/
public val kinesisSettings: aws.sdk.kotlin.services.databasemigrationservice.model.KinesisSettings? = builder.kinesisSettings
/**
* An KMS key identifier that is used to encrypt the connection parameters for the endpoint.
*
* If you don't specify a value for the `KmsKeyId` parameter, then DMS uses your default encryption key.
*
* KMS creates the default encryption key for your Amazon Web Services account. Your Amazon Web Services account has a different default encryption key for each Amazon Web Services Region.
*/
public val kmsKeyId: kotlin.String? = builder.kmsKeyId
/**
* Settings in JSON format for the source and target Microsoft SQL Server endpoint. For information about other available settings, see [Extra connection attributes when using SQL Server as a source for DMS](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Source.SQLServer.html#CHAP_Source.SQLServer.ConnectionAttrib) and [ Extra connection attributes when using SQL Server as a target for DMS](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Target.SQLServer.html#CHAP_Target.SQLServer.ConnectionAttrib) in the *Database Migration Service User Guide.*
*/
public val microsoftSqlServerSettings: aws.sdk.kotlin.services.databasemigrationservice.model.MicrosoftSqlServerSettings? = builder.microsoftSqlServerSettings
/**
* Settings in JSON format for the source MongoDB endpoint. For more information about the available settings, see [Endpoint configuration settings when using MongoDB as a source for Database Migration Service](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Source.MongoDB.html#CHAP_Source.MongoDB.Configuration) in the *Database Migration Service User Guide.*
*/
public val mongoDbSettings: aws.sdk.kotlin.services.databasemigrationservice.model.MongoDbSettings? = builder.mongoDbSettings
/**
* Settings in JSON format for the source and target MySQL endpoint. For information about other available settings, see [Extra connection attributes when using MySQL as a source for DMS](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Source.MySQL.html#CHAP_Source.MySQL.ConnectionAttrib) and [Extra connection attributes when using a MySQL-compatible database as a target for DMS](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Target.MySQL.html#CHAP_Target.MySQL.ConnectionAttrib) in the *Database Migration Service User Guide.*
*/
public val mySqlSettings: aws.sdk.kotlin.services.databasemigrationservice.model.MySqlSettings? = builder.mySqlSettings
/**
* Settings in JSON format for the target Amazon Neptune endpoint. For more information about the available settings, see [Specifying graph-mapping rules using Gremlin and R2RML for Amazon Neptune as a target](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Target.Neptune.html#CHAP_Target.Neptune.EndpointSettings) in the *Database Migration Service User Guide.*
*/
public val neptuneSettings: aws.sdk.kotlin.services.databasemigrationservice.model.NeptuneSettings? = builder.neptuneSettings
/**
* Settings in JSON format for the source and target Oracle endpoint. For information about other available settings, see [Extra connection attributes when using Oracle as a source for DMS](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Source.Oracle.html#CHAP_Source.Oracle.ConnectionAttrib) and [ Extra connection attributes when using Oracle as a target for DMS](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Target.Oracle.html#CHAP_Target.Oracle.ConnectionAttrib) in the *Database Migration Service User Guide.*
*/
public val oracleSettings: aws.sdk.kotlin.services.databasemigrationservice.model.OracleSettings? = builder.oracleSettings
/**
* The password to be used to log in to the endpoint database.
*/
public val password: kotlin.String? = builder.password
/**
* The port used by the endpoint database.
*/
public val port: kotlin.Int? = builder.port
/**
* Settings in JSON format for the source and target PostgreSQL endpoint. For information about other available settings, see [Extra connection attributes when using PostgreSQL as a source for DMS](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Source.PostgreSQL.html#CHAP_Source.PostgreSQL.ConnectionAttrib) and [ Extra connection attributes when using PostgreSQL as a target for DMS](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Target.PostgreSQL.html#CHAP_Target.PostgreSQL.ConnectionAttrib) in the *Database Migration Service User Guide.*
*/
public val postgreSqlSettings: aws.sdk.kotlin.services.databasemigrationservice.model.PostgreSqlSettings? = builder.postgreSqlSettings
/**
* Settings in JSON format for the target Redis endpoint.
*/
public val redisSettings: aws.sdk.kotlin.services.databasemigrationservice.model.RedisSettings? = builder.redisSettings
/**
* Provides information that defines an Amazon Redshift endpoint.
*/
public val redshiftSettings: aws.sdk.kotlin.services.databasemigrationservice.model.RedshiftSettings? = builder.redshiftSettings
/**
* A friendly name for the resource identifier at the end of the `EndpointArn` response parameter that is returned in the created `Endpoint` object. The value for this parameter can have up to 31 characters. It can contain only ASCII letters, digits, and hyphen ('-'). Also, it can't end with a hyphen or contain two consecutive hyphens, and can only begin with a letter, such as `Example-App-ARN1`. For example, this value might result in the `EndpointArn` value `arn:aws:dms:eu-west-1:012345678901:rep:Example-App-ARN1`. If you don't specify a `ResourceIdentifier` value, DMS generates a default identifier value for the end of `EndpointArn`.
*/
public val resourceIdentifier: kotlin.String? = builder.resourceIdentifier
/**
* Settings in JSON format for the target Amazon S3 endpoint. For more information about the available settings, see [Extra Connection Attributes When Using Amazon S3 as a Target for DMS](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Target.S3.html#CHAP_Target.S3.Configuring) in the *Database Migration Service User Guide.*
*/
public val s3Settings: aws.sdk.kotlin.services.databasemigrationservice.model.S3Settings? = builder.s3Settings
/**
* The name of the server where the endpoint database resides.
*/
public val serverName: kotlin.String? = builder.serverName
/**
* The Amazon Resource Name (ARN) for the service access role that you want to use to create the endpoint. The role must allow the `iam:PassRole` action.
*/
public val serviceAccessRoleArn: kotlin.String? = builder.serviceAccessRoleArn
/**
* The Secure Sockets Layer (SSL) mode to use for the SSL connection. The default is `none`
*/
public val sslMode: aws.sdk.kotlin.services.databasemigrationservice.model.DmsSslModeValue? = builder.sslMode
/**
* Settings in JSON format for the source and target SAP ASE endpoint. For information about other available settings, see [Extra connection attributes when using SAP ASE as a source for DMS](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Source.SAP.html#CHAP_Source.SAP.ConnectionAttrib) and [Extra connection attributes when using SAP ASE as a target for DMS](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Target.SAP.html#CHAP_Target.SAP.ConnectionAttrib) in the *Database Migration Service User Guide.*
*/
public val sybaseSettings: aws.sdk.kotlin.services.databasemigrationservice.model.SybaseSettings? = builder.sybaseSettings
/**
* One or more tags to be assigned to the endpoint.
*/
public val tags: List? = builder.tags
/**
* Settings in JSON format for the target Amazon Timestream endpoint.
*/
public val timestreamSettings: aws.sdk.kotlin.services.databasemigrationservice.model.TimestreamSettings? = builder.timestreamSettings
/**
* The user name to be used to log in to the endpoint database.
*/
public val username: kotlin.String? = builder.username
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.databasemigrationservice.model.CreateEndpointRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("CreateEndpointRequest(")
append("certificateArn=$certificateArn,")
append("databaseName=$databaseName,")
append("dmsTransferSettings=$dmsTransferSettings,")
append("docDbSettings=$docDbSettings,")
append("dynamoDbSettings=$dynamoDbSettings,")
append("elasticsearchSettings=$elasticsearchSettings,")
append("endpointIdentifier=$endpointIdentifier,")
append("endpointType=$endpointType,")
append("engineName=$engineName,")
append("externalTableDefinition=$externalTableDefinition,")
append("extraConnectionAttributes=$extraConnectionAttributes,")
append("gcpMySqlSettings=$gcpMySqlSettings,")
append("ibmDb2Settings=$ibmDb2Settings,")
append("kafkaSettings=$kafkaSettings,")
append("kinesisSettings=$kinesisSettings,")
append("kmsKeyId=$kmsKeyId,")
append("microsoftSqlServerSettings=$microsoftSqlServerSettings,")
append("mongoDbSettings=$mongoDbSettings,")
append("mySqlSettings=$mySqlSettings,")
append("neptuneSettings=$neptuneSettings,")
append("oracleSettings=$oracleSettings,")
append("password=*** Sensitive Data Redacted ***,")
append("port=$port,")
append("postgreSqlSettings=$postgreSqlSettings,")
append("redisSettings=$redisSettings,")
append("redshiftSettings=$redshiftSettings,")
append("resourceIdentifier=$resourceIdentifier,")
append("s3Settings=$s3Settings,")
append("serverName=$serverName,")
append("serviceAccessRoleArn=$serviceAccessRoleArn,")
append("sslMode=$sslMode,")
append("sybaseSettings=$sybaseSettings,")
append("tags=$tags,")
append("timestreamSettings=$timestreamSettings,")
append("username=$username")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = certificateArn?.hashCode() ?: 0
result = 31 * result + (databaseName?.hashCode() ?: 0)
result = 31 * result + (dmsTransferSettings?.hashCode() ?: 0)
result = 31 * result + (docDbSettings?.hashCode() ?: 0)
result = 31 * result + (dynamoDbSettings?.hashCode() ?: 0)
result = 31 * result + (elasticsearchSettings?.hashCode() ?: 0)
result = 31 * result + (endpointIdentifier?.hashCode() ?: 0)
result = 31 * result + (endpointType?.hashCode() ?: 0)
result = 31 * result + (engineName?.hashCode() ?: 0)
result = 31 * result + (externalTableDefinition?.hashCode() ?: 0)
result = 31 * result + (extraConnectionAttributes?.hashCode() ?: 0)
result = 31 * result + (gcpMySqlSettings?.hashCode() ?: 0)
result = 31 * result + (ibmDb2Settings?.hashCode() ?: 0)
result = 31 * result + (kafkaSettings?.hashCode() ?: 0)
result = 31 * result + (kinesisSettings?.hashCode() ?: 0)
result = 31 * result + (kmsKeyId?.hashCode() ?: 0)
result = 31 * result + (microsoftSqlServerSettings?.hashCode() ?: 0)
result = 31 * result + (mongoDbSettings?.hashCode() ?: 0)
result = 31 * result + (mySqlSettings?.hashCode() ?: 0)
result = 31 * result + (neptuneSettings?.hashCode() ?: 0)
result = 31 * result + (oracleSettings?.hashCode() ?: 0)
result = 31 * result + (password?.hashCode() ?: 0)
result = 31 * result + (port ?: 0)
result = 31 * result + (postgreSqlSettings?.hashCode() ?: 0)
result = 31 * result + (redisSettings?.hashCode() ?: 0)
result = 31 * result + (redshiftSettings?.hashCode() ?: 0)
result = 31 * result + (resourceIdentifier?.hashCode() ?: 0)
result = 31 * result + (s3Settings?.hashCode() ?: 0)
result = 31 * result + (serverName?.hashCode() ?: 0)
result = 31 * result + (serviceAccessRoleArn?.hashCode() ?: 0)
result = 31 * result + (sslMode?.hashCode() ?: 0)
result = 31 * result + (sybaseSettings?.hashCode() ?: 0)
result = 31 * result + (tags?.hashCode() ?: 0)
result = 31 * result + (timestreamSettings?.hashCode() ?: 0)
result = 31 * result + (username?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as CreateEndpointRequest
if (certificateArn != other.certificateArn) return false
if (databaseName != other.databaseName) return false
if (dmsTransferSettings != other.dmsTransferSettings) return false
if (docDbSettings != other.docDbSettings) return false
if (dynamoDbSettings != other.dynamoDbSettings) return false
if (elasticsearchSettings != other.elasticsearchSettings) return false
if (endpointIdentifier != other.endpointIdentifier) return false
if (endpointType != other.endpointType) return false
if (engineName != other.engineName) return false
if (externalTableDefinition != other.externalTableDefinition) return false
if (extraConnectionAttributes != other.extraConnectionAttributes) return false
if (gcpMySqlSettings != other.gcpMySqlSettings) return false
if (ibmDb2Settings != other.ibmDb2Settings) return false
if (kafkaSettings != other.kafkaSettings) return false
if (kinesisSettings != other.kinesisSettings) return false
if (kmsKeyId != other.kmsKeyId) return false
if (microsoftSqlServerSettings != other.microsoftSqlServerSettings) return false
if (mongoDbSettings != other.mongoDbSettings) return false
if (mySqlSettings != other.mySqlSettings) return false
if (neptuneSettings != other.neptuneSettings) return false
if (oracleSettings != other.oracleSettings) return false
if (password != other.password) return false
if (port != other.port) return false
if (postgreSqlSettings != other.postgreSqlSettings) return false
if (redisSettings != other.redisSettings) return false
if (redshiftSettings != other.redshiftSettings) return false
if (resourceIdentifier != other.resourceIdentifier) return false
if (s3Settings != other.s3Settings) return false
if (serverName != other.serverName) return false
if (serviceAccessRoleArn != other.serviceAccessRoleArn) return false
if (sslMode != other.sslMode) return false
if (sybaseSettings != other.sybaseSettings) return false
if (tags != other.tags) return false
if (timestreamSettings != other.timestreamSettings) return false
if (username != other.username) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.databasemigrationservice.model.CreateEndpointRequest = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The Amazon Resource Name (ARN) for the certificate.
*/
public var certificateArn: kotlin.String? = null
/**
* The name of the endpoint database. For a MySQL source or target endpoint, do not specify DatabaseName. To migrate to a specific database, use this setting and `targetDbType`.
*/
public var databaseName: kotlin.String? = null
/**
* The settings in JSON format for the DMS transfer type of source endpoint.
*
* Possible settings include the following:
* + `ServiceAccessRoleArn` - The Amazon Resource Name (ARN) used by the service access IAM role. The role must allow the `iam:PassRole` action.
* + `BucketName` - The name of the S3 bucket to use.
*
* Shorthand syntax for these settings is as follows: `ServiceAccessRoleArn=string,BucketName=string`
*
* JSON syntax for these settings is as follows: `{ "ServiceAccessRoleArn": "string", "BucketName": "string", } `
*/
public var dmsTransferSettings: aws.sdk.kotlin.services.databasemigrationservice.model.DmsTransferSettings? = null
/**
* Provides information that defines a DocumentDB endpoint.
*/
public var docDbSettings: aws.sdk.kotlin.services.databasemigrationservice.model.DocDbSettings? = null
/**
* Settings in JSON format for the target Amazon DynamoDB endpoint. For information about other available settings, see [Using Object Mapping to Migrate Data to DynamoDB](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Target.DynamoDB.html#CHAP_Target.DynamoDB.ObjectMapping) in the *Database Migration Service User Guide.*
*/
public var dynamoDbSettings: aws.sdk.kotlin.services.databasemigrationservice.model.DynamoDbSettings? = null
/**
* Settings in JSON format for the target OpenSearch endpoint. For more information about the available settings, see [Extra Connection Attributes When Using OpenSearch as a Target for DMS](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Target.Elasticsearch.html#CHAP_Target.Elasticsearch.Configuration) in the *Database Migration Service User Guide*.
*/
public var elasticsearchSettings: aws.sdk.kotlin.services.databasemigrationservice.model.ElasticsearchSettings? = null
/**
* The database endpoint identifier. Identifiers must begin with a letter and must contain only ASCII letters, digits, and hyphens. They can't end with a hyphen, or contain two consecutive hyphens.
*/
public var endpointIdentifier: kotlin.String? = null
/**
* The type of endpoint. Valid values are `source` and `target`.
*/
public var endpointType: aws.sdk.kotlin.services.databasemigrationservice.model.ReplicationEndpointTypeValue? = null
/**
* The type of engine for the endpoint. Valid values, depending on the `EndpointType` value, include `"mysql"`, `"oracle"`, `"postgres"`, `"mariadb"`, `"aurora"`, `"aurora-postgresql"`, `"opensearch"`, `"redshift"`, `"s3"`, `"db2"`, `"db2-zos"`, `"azuredb"`, `"sybase"`, `"dynamodb"`, `"mongodb"`, `"kinesis"`, `"kafka"`, `"elasticsearch"`, `"docdb"`, `"sqlserver"`, `"neptune"`, `"babelfish"`, `redshift-serverless`, `aurora-serverless`, `aurora-postgresql-serverless`, `gcp-mysql`, `azure-sql-managed-instance`, `redis`, `dms-transfer`.
*/
public var engineName: kotlin.String? = null
/**
* The external table definition.
*/
public var externalTableDefinition: kotlin.String? = null
/**
* Additional attributes associated with the connection. Each attribute is specified as a name-value pair associated by an equal sign (=). Multiple attributes are separated by a semicolon (;) with no additional white space. For information on the attributes available for connecting your source or target endpoint, see [Working with DMS Endpoints](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Endpoints.html) in the *Database Migration Service User Guide.*
*/
public var extraConnectionAttributes: kotlin.String? = null
/**
* Settings in JSON format for the source GCP MySQL endpoint.
*/
public var gcpMySqlSettings: aws.sdk.kotlin.services.databasemigrationservice.model.GcpMySqlSettings? = null
/**
* Settings in JSON format for the source IBM Db2 LUW endpoint. For information about other available settings, see [Extra connection attributes when using Db2 LUW as a source for DMS](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Source.DB2.html#CHAP_Source.DB2.ConnectionAttrib) in the *Database Migration Service User Guide.*
*/
public var ibmDb2Settings: aws.sdk.kotlin.services.databasemigrationservice.model.IbmDb2Settings? = null
/**
* Settings in JSON format for the target Apache Kafka endpoint. For more information about the available settings, see [Using object mapping to migrate data to a Kafka topic](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Target.Kafka.html#CHAP_Target.Kafka.ObjectMapping) in the *Database Migration Service User Guide.*
*/
public var kafkaSettings: aws.sdk.kotlin.services.databasemigrationservice.model.KafkaSettings? = null
/**
* Settings in JSON format for the target endpoint for Amazon Kinesis Data Streams. For more information about the available settings, see [Using object mapping to migrate data to a Kinesis data stream](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Target.Kinesis.html#CHAP_Target.Kinesis.ObjectMapping) in the *Database Migration Service User Guide.*
*/
public var kinesisSettings: aws.sdk.kotlin.services.databasemigrationservice.model.KinesisSettings? = null
/**
* An KMS key identifier that is used to encrypt the connection parameters for the endpoint.
*
* If you don't specify a value for the `KmsKeyId` parameter, then DMS uses your default encryption key.
*
* KMS creates the default encryption key for your Amazon Web Services account. Your Amazon Web Services account has a different default encryption key for each Amazon Web Services Region.
*/
public var kmsKeyId: kotlin.String? = null
/**
* Settings in JSON format for the source and target Microsoft SQL Server endpoint. For information about other available settings, see [Extra connection attributes when using SQL Server as a source for DMS](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Source.SQLServer.html#CHAP_Source.SQLServer.ConnectionAttrib) and [ Extra connection attributes when using SQL Server as a target for DMS](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Target.SQLServer.html#CHAP_Target.SQLServer.ConnectionAttrib) in the *Database Migration Service User Guide.*
*/
public var microsoftSqlServerSettings: aws.sdk.kotlin.services.databasemigrationservice.model.MicrosoftSqlServerSettings? = null
/**
* Settings in JSON format for the source MongoDB endpoint. For more information about the available settings, see [Endpoint configuration settings when using MongoDB as a source for Database Migration Service](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Source.MongoDB.html#CHAP_Source.MongoDB.Configuration) in the *Database Migration Service User Guide.*
*/
public var mongoDbSettings: aws.sdk.kotlin.services.databasemigrationservice.model.MongoDbSettings? = null
/**
* Settings in JSON format for the source and target MySQL endpoint. For information about other available settings, see [Extra connection attributes when using MySQL as a source for DMS](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Source.MySQL.html#CHAP_Source.MySQL.ConnectionAttrib) and [Extra connection attributes when using a MySQL-compatible database as a target for DMS](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Target.MySQL.html#CHAP_Target.MySQL.ConnectionAttrib) in the *Database Migration Service User Guide.*
*/
public var mySqlSettings: aws.sdk.kotlin.services.databasemigrationservice.model.MySqlSettings? = null
/**
* Settings in JSON format for the target Amazon Neptune endpoint. For more information about the available settings, see [Specifying graph-mapping rules using Gremlin and R2RML for Amazon Neptune as a target](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Target.Neptune.html#CHAP_Target.Neptune.EndpointSettings) in the *Database Migration Service User Guide.*
*/
public var neptuneSettings: aws.sdk.kotlin.services.databasemigrationservice.model.NeptuneSettings? = null
/**
* Settings in JSON format for the source and target Oracle endpoint. For information about other available settings, see [Extra connection attributes when using Oracle as a source for DMS](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Source.Oracle.html#CHAP_Source.Oracle.ConnectionAttrib) and [ Extra connection attributes when using Oracle as a target for DMS](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Target.Oracle.html#CHAP_Target.Oracle.ConnectionAttrib) in the *Database Migration Service User Guide.*
*/
public var oracleSettings: aws.sdk.kotlin.services.databasemigrationservice.model.OracleSettings? = null
/**
* The password to be used to log in to the endpoint database.
*/
public var password: kotlin.String? = null
/**
* The port used by the endpoint database.
*/
public var port: kotlin.Int? = null
/**
* Settings in JSON format for the source and target PostgreSQL endpoint. For information about other available settings, see [Extra connection attributes when using PostgreSQL as a source for DMS](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Source.PostgreSQL.html#CHAP_Source.PostgreSQL.ConnectionAttrib) and [ Extra connection attributes when using PostgreSQL as a target for DMS](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Target.PostgreSQL.html#CHAP_Target.PostgreSQL.ConnectionAttrib) in the *Database Migration Service User Guide.*
*/
public var postgreSqlSettings: aws.sdk.kotlin.services.databasemigrationservice.model.PostgreSqlSettings? = null
/**
* Settings in JSON format for the target Redis endpoint.
*/
public var redisSettings: aws.sdk.kotlin.services.databasemigrationservice.model.RedisSettings? = null
/**
* Provides information that defines an Amazon Redshift endpoint.
*/
public var redshiftSettings: aws.sdk.kotlin.services.databasemigrationservice.model.RedshiftSettings? = null
/**
* A friendly name for the resource identifier at the end of the `EndpointArn` response parameter that is returned in the created `Endpoint` object. The value for this parameter can have up to 31 characters. It can contain only ASCII letters, digits, and hyphen ('-'). Also, it can't end with a hyphen or contain two consecutive hyphens, and can only begin with a letter, such as `Example-App-ARN1`. For example, this value might result in the `EndpointArn` value `arn:aws:dms:eu-west-1:012345678901:rep:Example-App-ARN1`. If you don't specify a `ResourceIdentifier` value, DMS generates a default identifier value for the end of `EndpointArn`.
*/
public var resourceIdentifier: kotlin.String? = null
/**
* Settings in JSON format for the target Amazon S3 endpoint. For more information about the available settings, see [Extra Connection Attributes When Using Amazon S3 as a Target for DMS](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Target.S3.html#CHAP_Target.S3.Configuring) in the *Database Migration Service User Guide.*
*/
public var s3Settings: aws.sdk.kotlin.services.databasemigrationservice.model.S3Settings? = null
/**
* The name of the server where the endpoint database resides.
*/
public var serverName: kotlin.String? = null
/**
* The Amazon Resource Name (ARN) for the service access role that you want to use to create the endpoint. The role must allow the `iam:PassRole` action.
*/
public var serviceAccessRoleArn: kotlin.String? = null
/**
* The Secure Sockets Layer (SSL) mode to use for the SSL connection. The default is `none`
*/
public var sslMode: aws.sdk.kotlin.services.databasemigrationservice.model.DmsSslModeValue? = null
/**
* Settings in JSON format for the source and target SAP ASE endpoint. For information about other available settings, see [Extra connection attributes when using SAP ASE as a source for DMS](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Source.SAP.html#CHAP_Source.SAP.ConnectionAttrib) and [Extra connection attributes when using SAP ASE as a target for DMS](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Target.SAP.html#CHAP_Target.SAP.ConnectionAttrib) in the *Database Migration Service User Guide.*
*/
public var sybaseSettings: aws.sdk.kotlin.services.databasemigrationservice.model.SybaseSettings? = null
/**
* One or more tags to be assigned to the endpoint.
*/
public var tags: List? = null
/**
* Settings in JSON format for the target Amazon Timestream endpoint.
*/
public var timestreamSettings: aws.sdk.kotlin.services.databasemigrationservice.model.TimestreamSettings? = null
/**
* The user name to be used to log in to the endpoint database.
*/
public var username: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.databasemigrationservice.model.CreateEndpointRequest) : this() {
this.certificateArn = x.certificateArn
this.databaseName = x.databaseName
this.dmsTransferSettings = x.dmsTransferSettings
this.docDbSettings = x.docDbSettings
this.dynamoDbSettings = x.dynamoDbSettings
this.elasticsearchSettings = x.elasticsearchSettings
this.endpointIdentifier = x.endpointIdentifier
this.endpointType = x.endpointType
this.engineName = x.engineName
this.externalTableDefinition = x.externalTableDefinition
this.extraConnectionAttributes = x.extraConnectionAttributes
this.gcpMySqlSettings = x.gcpMySqlSettings
this.ibmDb2Settings = x.ibmDb2Settings
this.kafkaSettings = x.kafkaSettings
this.kinesisSettings = x.kinesisSettings
this.kmsKeyId = x.kmsKeyId
this.microsoftSqlServerSettings = x.microsoftSqlServerSettings
this.mongoDbSettings = x.mongoDbSettings
this.mySqlSettings = x.mySqlSettings
this.neptuneSettings = x.neptuneSettings
this.oracleSettings = x.oracleSettings
this.password = x.password
this.port = x.port
this.postgreSqlSettings = x.postgreSqlSettings
this.redisSettings = x.redisSettings
this.redshiftSettings = x.redshiftSettings
this.resourceIdentifier = x.resourceIdentifier
this.s3Settings = x.s3Settings
this.serverName = x.serverName
this.serviceAccessRoleArn = x.serviceAccessRoleArn
this.sslMode = x.sslMode
this.sybaseSettings = x.sybaseSettings
this.tags = x.tags
this.timestreamSettings = x.timestreamSettings
this.username = x.username
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.databasemigrationservice.model.CreateEndpointRequest = CreateEndpointRequest(this)
/**
* construct an [aws.sdk.kotlin.services.databasemigrationservice.model.DmsTransferSettings] inside the given [block]
*/
public fun dmsTransferSettings(block: aws.sdk.kotlin.services.databasemigrationservice.model.DmsTransferSettings.Builder.() -> kotlin.Unit) {
this.dmsTransferSettings = aws.sdk.kotlin.services.databasemigrationservice.model.DmsTransferSettings.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.databasemigrationservice.model.DocDbSettings] inside the given [block]
*/
public fun docDbSettings(block: aws.sdk.kotlin.services.databasemigrationservice.model.DocDbSettings.Builder.() -> kotlin.Unit) {
this.docDbSettings = aws.sdk.kotlin.services.databasemigrationservice.model.DocDbSettings.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.databasemigrationservice.model.DynamoDbSettings] inside the given [block]
*/
public fun dynamoDbSettings(block: aws.sdk.kotlin.services.databasemigrationservice.model.DynamoDbSettings.Builder.() -> kotlin.Unit) {
this.dynamoDbSettings = aws.sdk.kotlin.services.databasemigrationservice.model.DynamoDbSettings.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.databasemigrationservice.model.ElasticsearchSettings] inside the given [block]
*/
public fun elasticsearchSettings(block: aws.sdk.kotlin.services.databasemigrationservice.model.ElasticsearchSettings.Builder.() -> kotlin.Unit) {
this.elasticsearchSettings = aws.sdk.kotlin.services.databasemigrationservice.model.ElasticsearchSettings.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.databasemigrationservice.model.GcpMySqlSettings] inside the given [block]
*/
public fun gcpMySqlSettings(block: aws.sdk.kotlin.services.databasemigrationservice.model.GcpMySqlSettings.Builder.() -> kotlin.Unit) {
this.gcpMySqlSettings = aws.sdk.kotlin.services.databasemigrationservice.model.GcpMySqlSettings.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.databasemigrationservice.model.IbmDb2Settings] inside the given [block]
*/
public fun ibmDb2Settings(block: aws.sdk.kotlin.services.databasemigrationservice.model.IbmDb2Settings.Builder.() -> kotlin.Unit) {
this.ibmDb2Settings = aws.sdk.kotlin.services.databasemigrationservice.model.IbmDb2Settings.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.databasemigrationservice.model.KafkaSettings] inside the given [block]
*/
public fun kafkaSettings(block: aws.sdk.kotlin.services.databasemigrationservice.model.KafkaSettings.Builder.() -> kotlin.Unit) {
this.kafkaSettings = aws.sdk.kotlin.services.databasemigrationservice.model.KafkaSettings.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.databasemigrationservice.model.KinesisSettings] inside the given [block]
*/
public fun kinesisSettings(block: aws.sdk.kotlin.services.databasemigrationservice.model.KinesisSettings.Builder.() -> kotlin.Unit) {
this.kinesisSettings = aws.sdk.kotlin.services.databasemigrationservice.model.KinesisSettings.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.databasemigrationservice.model.MicrosoftSqlServerSettings] inside the given [block]
*/
public fun microsoftSqlServerSettings(block: aws.sdk.kotlin.services.databasemigrationservice.model.MicrosoftSqlServerSettings.Builder.() -> kotlin.Unit) {
this.microsoftSqlServerSettings = aws.sdk.kotlin.services.databasemigrationservice.model.MicrosoftSqlServerSettings.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.databasemigrationservice.model.MongoDbSettings] inside the given [block]
*/
public fun mongoDbSettings(block: aws.sdk.kotlin.services.databasemigrationservice.model.MongoDbSettings.Builder.() -> kotlin.Unit) {
this.mongoDbSettings = aws.sdk.kotlin.services.databasemigrationservice.model.MongoDbSettings.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.databasemigrationservice.model.MySqlSettings] inside the given [block]
*/
public fun mySqlSettings(block: aws.sdk.kotlin.services.databasemigrationservice.model.MySqlSettings.Builder.() -> kotlin.Unit) {
this.mySqlSettings = aws.sdk.kotlin.services.databasemigrationservice.model.MySqlSettings.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.databasemigrationservice.model.NeptuneSettings] inside the given [block]
*/
public fun neptuneSettings(block: aws.sdk.kotlin.services.databasemigrationservice.model.NeptuneSettings.Builder.() -> kotlin.Unit) {
this.neptuneSettings = aws.sdk.kotlin.services.databasemigrationservice.model.NeptuneSettings.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.databasemigrationservice.model.OracleSettings] inside the given [block]
*/
public fun oracleSettings(block: aws.sdk.kotlin.services.databasemigrationservice.model.OracleSettings.Builder.() -> kotlin.Unit) {
this.oracleSettings = aws.sdk.kotlin.services.databasemigrationservice.model.OracleSettings.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.databasemigrationservice.model.PostgreSqlSettings] inside the given [block]
*/
public fun postgreSqlSettings(block: aws.sdk.kotlin.services.databasemigrationservice.model.PostgreSqlSettings.Builder.() -> kotlin.Unit) {
this.postgreSqlSettings = aws.sdk.kotlin.services.databasemigrationservice.model.PostgreSqlSettings.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.databasemigrationservice.model.RedisSettings] inside the given [block]
*/
public fun redisSettings(block: aws.sdk.kotlin.services.databasemigrationservice.model.RedisSettings.Builder.() -> kotlin.Unit) {
this.redisSettings = aws.sdk.kotlin.services.databasemigrationservice.model.RedisSettings.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.databasemigrationservice.model.RedshiftSettings] inside the given [block]
*/
public fun redshiftSettings(block: aws.sdk.kotlin.services.databasemigrationservice.model.RedshiftSettings.Builder.() -> kotlin.Unit) {
this.redshiftSettings = aws.sdk.kotlin.services.databasemigrationservice.model.RedshiftSettings.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.databasemigrationservice.model.S3Settings] inside the given [block]
*/
public fun s3Settings(block: aws.sdk.kotlin.services.databasemigrationservice.model.S3Settings.Builder.() -> kotlin.Unit) {
this.s3Settings = aws.sdk.kotlin.services.databasemigrationservice.model.S3Settings.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.databasemigrationservice.model.SybaseSettings] inside the given [block]
*/
public fun sybaseSettings(block: aws.sdk.kotlin.services.databasemigrationservice.model.SybaseSettings.Builder.() -> kotlin.Unit) {
this.sybaseSettings = aws.sdk.kotlin.services.databasemigrationservice.model.SybaseSettings.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.databasemigrationservice.model.TimestreamSettings] inside the given [block]
*/
public fun timestreamSettings(block: aws.sdk.kotlin.services.databasemigrationservice.model.TimestreamSettings.Builder.() -> kotlin.Unit) {
this.timestreamSettings = aws.sdk.kotlin.services.databasemigrationservice.model.TimestreamSettings.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy