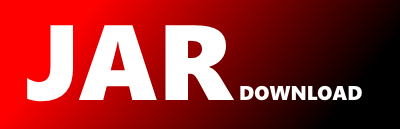
commonMain.aws.sdk.kotlin.services.databasemigrationservice.model.CreateMigrationProjectRequest.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of databasemigrationservice-jvm Show documentation
Show all versions of databasemigrationservice-jvm Show documentation
The AWS SDK for Kotlin client for Database Migration Service
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.databasemigrationservice.model
import aws.smithy.kotlin.runtime.SdkDsl
public class CreateMigrationProjectRequest private constructor(builder: Builder) {
/**
* A user-friendly description of the migration project.
*/
public val description: kotlin.String? = builder.description
/**
* The identifier of the associated instance profile. Identifiers must begin with a letter and must contain only ASCII letters, digits, and hyphens. They can't end with a hyphen, or contain two consecutive hyphens.
*/
public val instanceProfileIdentifier: kotlin.String? = builder.instanceProfileIdentifier
/**
* A user-friendly name for the migration project.
*/
public val migrationProjectName: kotlin.String? = builder.migrationProjectName
/**
* The schema conversion application attributes, including the Amazon S3 bucket name and Amazon S3 role ARN.
*/
public val schemaConversionApplicationAttributes: aws.sdk.kotlin.services.databasemigrationservice.model.ScApplicationAttributes? = builder.schemaConversionApplicationAttributes
/**
* Information about the source data provider, including the name, ARN, and Secrets Manager parameters.
*/
public val sourceDataProviderDescriptors: List? = builder.sourceDataProviderDescriptors
/**
* One or more tags to be assigned to the migration project.
*/
public val tags: List? = builder.tags
/**
* Information about the target data provider, including the name, ARN, and Amazon Web Services Secrets Manager parameters.
*/
public val targetDataProviderDescriptors: List? = builder.targetDataProviderDescriptors
/**
* The settings in JSON format for migration rules. Migration rules make it possible for you to change the object names according to the rules that you specify. For example, you can change an object name to lowercase or uppercase, add or remove a prefix or suffix, or rename objects.
*/
public val transformationRules: kotlin.String? = builder.transformationRules
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.databasemigrationservice.model.CreateMigrationProjectRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("CreateMigrationProjectRequest(")
append("description=$description,")
append("instanceProfileIdentifier=$instanceProfileIdentifier,")
append("migrationProjectName=$migrationProjectName,")
append("schemaConversionApplicationAttributes=$schemaConversionApplicationAttributes,")
append("sourceDataProviderDescriptors=$sourceDataProviderDescriptors,")
append("tags=$tags,")
append("targetDataProviderDescriptors=$targetDataProviderDescriptors,")
append("transformationRules=$transformationRules")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = description?.hashCode() ?: 0
result = 31 * result + (instanceProfileIdentifier?.hashCode() ?: 0)
result = 31 * result + (migrationProjectName?.hashCode() ?: 0)
result = 31 * result + (schemaConversionApplicationAttributes?.hashCode() ?: 0)
result = 31 * result + (sourceDataProviderDescriptors?.hashCode() ?: 0)
result = 31 * result + (tags?.hashCode() ?: 0)
result = 31 * result + (targetDataProviderDescriptors?.hashCode() ?: 0)
result = 31 * result + (transformationRules?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as CreateMigrationProjectRequest
if (description != other.description) return false
if (instanceProfileIdentifier != other.instanceProfileIdentifier) return false
if (migrationProjectName != other.migrationProjectName) return false
if (schemaConversionApplicationAttributes != other.schemaConversionApplicationAttributes) return false
if (sourceDataProviderDescriptors != other.sourceDataProviderDescriptors) return false
if (tags != other.tags) return false
if (targetDataProviderDescriptors != other.targetDataProviderDescriptors) return false
if (transformationRules != other.transformationRules) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.databasemigrationservice.model.CreateMigrationProjectRequest = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* A user-friendly description of the migration project.
*/
public var description: kotlin.String? = null
/**
* The identifier of the associated instance profile. Identifiers must begin with a letter and must contain only ASCII letters, digits, and hyphens. They can't end with a hyphen, or contain two consecutive hyphens.
*/
public var instanceProfileIdentifier: kotlin.String? = null
/**
* A user-friendly name for the migration project.
*/
public var migrationProjectName: kotlin.String? = null
/**
* The schema conversion application attributes, including the Amazon S3 bucket name and Amazon S3 role ARN.
*/
public var schemaConversionApplicationAttributes: aws.sdk.kotlin.services.databasemigrationservice.model.ScApplicationAttributes? = null
/**
* Information about the source data provider, including the name, ARN, and Secrets Manager parameters.
*/
public var sourceDataProviderDescriptors: List? = null
/**
* One or more tags to be assigned to the migration project.
*/
public var tags: List? = null
/**
* Information about the target data provider, including the name, ARN, and Amazon Web Services Secrets Manager parameters.
*/
public var targetDataProviderDescriptors: List? = null
/**
* The settings in JSON format for migration rules. Migration rules make it possible for you to change the object names according to the rules that you specify. For example, you can change an object name to lowercase or uppercase, add or remove a prefix or suffix, or rename objects.
*/
public var transformationRules: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.databasemigrationservice.model.CreateMigrationProjectRequest) : this() {
this.description = x.description
this.instanceProfileIdentifier = x.instanceProfileIdentifier
this.migrationProjectName = x.migrationProjectName
this.schemaConversionApplicationAttributes = x.schemaConversionApplicationAttributes
this.sourceDataProviderDescriptors = x.sourceDataProviderDescriptors
this.tags = x.tags
this.targetDataProviderDescriptors = x.targetDataProviderDescriptors
this.transformationRules = x.transformationRules
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.databasemigrationservice.model.CreateMigrationProjectRequest = CreateMigrationProjectRequest(this)
/**
* construct an [aws.sdk.kotlin.services.databasemigrationservice.model.ScApplicationAttributes] inside the given [block]
*/
public fun schemaConversionApplicationAttributes(block: aws.sdk.kotlin.services.databasemigrationservice.model.ScApplicationAttributes.Builder.() -> kotlin.Unit) {
this.schemaConversionApplicationAttributes = aws.sdk.kotlin.services.databasemigrationservice.model.ScApplicationAttributes.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy