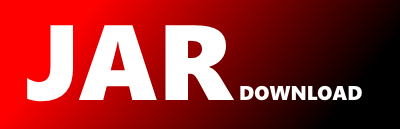
commonMain.aws.sdk.kotlin.services.databasemigrationservice.model.CreateReplicationConfigRequest.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of databasemigrationservice-jvm Show documentation
Show all versions of databasemigrationservice-jvm Show documentation
The AWS SDK for Kotlin client for Database Migration Service
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.databasemigrationservice.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
*
*/
public class CreateReplicationConfigRequest private constructor(builder: Builder) {
/**
* Configuration parameters for provisioning an DMS Serverless replication.
*/
public val computeConfig: aws.sdk.kotlin.services.databasemigrationservice.model.ComputeConfig? = builder.computeConfig
/**
* A unique identifier that you want to use to create a `ReplicationConfigArn` that is returned as part of the output from this action. You can then pass this output `ReplicationConfigArn` as the value of the `ReplicationConfigArn` option for other actions to identify both DMS Serverless replications and replication configurations that you want those actions to operate on. For some actions, you can also use either this unique identifier or a corresponding ARN in action filters to identify the specific replication and replication configuration to operate on.
*/
public val replicationConfigIdentifier: kotlin.String? = builder.replicationConfigIdentifier
/**
* Optional JSON settings for DMS Serverless replications that are provisioned using this replication configuration. For example, see [ Change processing tuning settings](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Tasks.CustomizingTasks.TaskSettings.ChangeProcessingTuning.html).
*/
public val replicationSettings: kotlin.String? = builder.replicationSettings
/**
* The type of DMS Serverless replication to provision using this replication configuration.
*
* Possible values:
* + `"full-load"`
* + `"cdc"`
* + `"full-load-and-cdc"`
*/
public val replicationType: aws.sdk.kotlin.services.databasemigrationservice.model.MigrationTypeValue? = builder.replicationType
/**
* Optional unique value or name that you set for a given resource that can be used to construct an Amazon Resource Name (ARN) for that resource. For more information, see [ Fine-grained access control using resource names and tags](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Security.html#CHAP_Security.FineGrainedAccess).
*/
public val resourceIdentifier: kotlin.String? = builder.resourceIdentifier
/**
* The Amazon Resource Name (ARN) of the source endpoint for this DMS Serverless replication configuration.
*/
public val sourceEndpointArn: kotlin.String? = builder.sourceEndpointArn
/**
* Optional JSON settings for specifying supplemental data. For more information, see [ Specifying supplemental data for task settings](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Tasks.TaskData.html).
*/
public val supplementalSettings: kotlin.String? = builder.supplementalSettings
/**
* JSON table mappings for DMS Serverless replications that are provisioned using this replication configuration. For more information, see [ Specifying table selection and transformations rules using JSON](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Tasks.CustomizingTasks.TableMapping.SelectionTransformation.html).
*/
public val tableMappings: kotlin.String? = builder.tableMappings
/**
* One or more optional tags associated with resources used by the DMS Serverless replication. For more information, see [ Tagging resources in Database Migration Service](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Tagging.html).
*/
public val tags: List? = builder.tags
/**
* The Amazon Resource Name (ARN) of the target endpoint for this DMS serverless replication configuration.
*/
public val targetEndpointArn: kotlin.String? = builder.targetEndpointArn
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.databasemigrationservice.model.CreateReplicationConfigRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("CreateReplicationConfigRequest(")
append("computeConfig=$computeConfig,")
append("replicationConfigIdentifier=$replicationConfigIdentifier,")
append("replicationSettings=$replicationSettings,")
append("replicationType=$replicationType,")
append("resourceIdentifier=$resourceIdentifier,")
append("sourceEndpointArn=$sourceEndpointArn,")
append("supplementalSettings=$supplementalSettings,")
append("tableMappings=$tableMappings,")
append("tags=$tags,")
append("targetEndpointArn=$targetEndpointArn")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = computeConfig?.hashCode() ?: 0
result = 31 * result + (replicationConfigIdentifier?.hashCode() ?: 0)
result = 31 * result + (replicationSettings?.hashCode() ?: 0)
result = 31 * result + (replicationType?.hashCode() ?: 0)
result = 31 * result + (resourceIdentifier?.hashCode() ?: 0)
result = 31 * result + (sourceEndpointArn?.hashCode() ?: 0)
result = 31 * result + (supplementalSettings?.hashCode() ?: 0)
result = 31 * result + (tableMappings?.hashCode() ?: 0)
result = 31 * result + (tags?.hashCode() ?: 0)
result = 31 * result + (targetEndpointArn?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as CreateReplicationConfigRequest
if (computeConfig != other.computeConfig) return false
if (replicationConfigIdentifier != other.replicationConfigIdentifier) return false
if (replicationSettings != other.replicationSettings) return false
if (replicationType != other.replicationType) return false
if (resourceIdentifier != other.resourceIdentifier) return false
if (sourceEndpointArn != other.sourceEndpointArn) return false
if (supplementalSettings != other.supplementalSettings) return false
if (tableMappings != other.tableMappings) return false
if (tags != other.tags) return false
if (targetEndpointArn != other.targetEndpointArn) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.databasemigrationservice.model.CreateReplicationConfigRequest = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* Configuration parameters for provisioning an DMS Serverless replication.
*/
public var computeConfig: aws.sdk.kotlin.services.databasemigrationservice.model.ComputeConfig? = null
/**
* A unique identifier that you want to use to create a `ReplicationConfigArn` that is returned as part of the output from this action. You can then pass this output `ReplicationConfigArn` as the value of the `ReplicationConfigArn` option for other actions to identify both DMS Serverless replications and replication configurations that you want those actions to operate on. For some actions, you can also use either this unique identifier or a corresponding ARN in action filters to identify the specific replication and replication configuration to operate on.
*/
public var replicationConfigIdentifier: kotlin.String? = null
/**
* Optional JSON settings for DMS Serverless replications that are provisioned using this replication configuration. For example, see [ Change processing tuning settings](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Tasks.CustomizingTasks.TaskSettings.ChangeProcessingTuning.html).
*/
public var replicationSettings: kotlin.String? = null
/**
* The type of DMS Serverless replication to provision using this replication configuration.
*
* Possible values:
* + `"full-load"`
* + `"cdc"`
* + `"full-load-and-cdc"`
*/
public var replicationType: aws.sdk.kotlin.services.databasemigrationservice.model.MigrationTypeValue? = null
/**
* Optional unique value or name that you set for a given resource that can be used to construct an Amazon Resource Name (ARN) for that resource. For more information, see [ Fine-grained access control using resource names and tags](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Security.html#CHAP_Security.FineGrainedAccess).
*/
public var resourceIdentifier: kotlin.String? = null
/**
* The Amazon Resource Name (ARN) of the source endpoint for this DMS Serverless replication configuration.
*/
public var sourceEndpointArn: kotlin.String? = null
/**
* Optional JSON settings for specifying supplemental data. For more information, see [ Specifying supplemental data for task settings](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Tasks.TaskData.html).
*/
public var supplementalSettings: kotlin.String? = null
/**
* JSON table mappings for DMS Serverless replications that are provisioned using this replication configuration. For more information, see [ Specifying table selection and transformations rules using JSON](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Tasks.CustomizingTasks.TableMapping.SelectionTransformation.html).
*/
public var tableMappings: kotlin.String? = null
/**
* One or more optional tags associated with resources used by the DMS Serverless replication. For more information, see [ Tagging resources in Database Migration Service](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_Tagging.html).
*/
public var tags: List? = null
/**
* The Amazon Resource Name (ARN) of the target endpoint for this DMS serverless replication configuration.
*/
public var targetEndpointArn: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.databasemigrationservice.model.CreateReplicationConfigRequest) : this() {
this.computeConfig = x.computeConfig
this.replicationConfigIdentifier = x.replicationConfigIdentifier
this.replicationSettings = x.replicationSettings
this.replicationType = x.replicationType
this.resourceIdentifier = x.resourceIdentifier
this.sourceEndpointArn = x.sourceEndpointArn
this.supplementalSettings = x.supplementalSettings
this.tableMappings = x.tableMappings
this.tags = x.tags
this.targetEndpointArn = x.targetEndpointArn
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.databasemigrationservice.model.CreateReplicationConfigRequest = CreateReplicationConfigRequest(this)
/**
* construct an [aws.sdk.kotlin.services.databasemigrationservice.model.ComputeConfig] inside the given [block]
*/
public fun computeConfig(block: aws.sdk.kotlin.services.databasemigrationservice.model.ComputeConfig.Builder.() -> kotlin.Unit) {
this.computeConfig = aws.sdk.kotlin.services.databasemigrationservice.model.ComputeConfig.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy