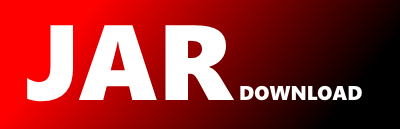
commonMain.aws.sdk.kotlin.services.databasemigrationservice.model.CreateReplicationInstanceRequest.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of databasemigrationservice-jvm Show documentation
Show all versions of databasemigrationservice-jvm Show documentation
The AWS SDK for Kotlin client for Database Migration Service
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.databasemigrationservice.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
*
*/
public class CreateReplicationInstanceRequest private constructor(builder: Builder) {
/**
* The amount of storage (in gigabytes) to be initially allocated for the replication instance.
*/
public val allocatedStorage: kotlin.Int? = builder.allocatedStorage
/**
* A value that indicates whether minor engine upgrades are applied automatically to the replication instance during the maintenance window. This parameter defaults to `true`.
*
* Default: `true`
*/
public val autoMinorVersionUpgrade: kotlin.Boolean? = builder.autoMinorVersionUpgrade
/**
* The Availability Zone where the replication instance will be created. The default value is a random, system-chosen Availability Zone in the endpoint's Amazon Web Services Region, for example: `us-east-1d`.
*/
public val availabilityZone: kotlin.String? = builder.availabilityZone
/**
* A list of custom DNS name servers supported for the replication instance to access your on-premise source or target database. This list overrides the default name servers supported by the replication instance. You can specify a comma-separated list of internet addresses for up to four on-premise DNS name servers. For example: `"1.1.1.1,2.2.2.2,3.3.3.3,4.4.4.4"`
*/
public val dnsNameServers: kotlin.String? = builder.dnsNameServers
/**
* The engine version number of the replication instance.
*
* If an engine version number is not specified when a replication instance is created, the default is the latest engine version available.
*/
public val engineVersion: kotlin.String? = builder.engineVersion
/**
* Specifies the ID of the secret that stores the key cache file required for kerberos authentication, when creating a replication instance.
*/
public val kerberosAuthenticationSettings: aws.sdk.kotlin.services.databasemigrationservice.model.KerberosAuthenticationSettings? = builder.kerberosAuthenticationSettings
/**
* An KMS key identifier that is used to encrypt the data on the replication instance.
*
* If you don't specify a value for the `KmsKeyId` parameter, then DMS uses your default encryption key.
*
* KMS creates the default encryption key for your Amazon Web Services account. Your Amazon Web Services account has a different default encryption key for each Amazon Web Services Region.
*/
public val kmsKeyId: kotlin.String? = builder.kmsKeyId
/**
* Specifies whether the replication instance is a Multi-AZ deployment. You can't set the `AvailabilityZone` parameter if the Multi-AZ parameter is set to `true`.
*/
public val multiAz: kotlin.Boolean? = builder.multiAz
/**
* The type of IP address protocol used by a replication instance, such as IPv4 only or Dual-stack that supports both IPv4 and IPv6 addressing. IPv6 only is not yet supported.
*/
public val networkType: kotlin.String? = builder.networkType
/**
* The weekly time range during which system maintenance can occur, in Universal Coordinated Time (UTC).
*
* Format: `ddd:hh24:mi-ddd:hh24:mi`
*
* Default: A 30-minute window selected at random from an 8-hour block of time per Amazon Web Services Region, occurring on a random day of the week.
*
* Valid Days: Mon, Tue, Wed, Thu, Fri, Sat, Sun
*
* Constraints: Minimum 30-minute window.
*/
public val preferredMaintenanceWindow: kotlin.String? = builder.preferredMaintenanceWindow
/**
* Specifies the accessibility options for the replication instance. A value of `true` represents an instance with a public IP address. A value of `false` represents an instance with a private IP address. The default value is `true`.
*/
public val publiclyAccessible: kotlin.Boolean? = builder.publiclyAccessible
/**
* The compute and memory capacity of the replication instance as defined for the specified replication instance class. For example to specify the instance class dms.c4.large, set this parameter to `"dms.c4.large"`.
*
* For more information on the settings and capacities for the available replication instance classes, see [ Choosing the right DMS replication instance](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_ReplicationInstance.Types.html ); and, [Selecting the best size for a replication instance](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_BestPractices.SizingReplicationInstance.html).
*/
public val replicationInstanceClass: kotlin.String? = builder.replicationInstanceClass
/**
* The replication instance identifier. This parameter is stored as a lowercase string.
*
* Constraints:
* + Must contain 1-63 alphanumeric characters or hyphens.
* + First character must be a letter.
* + Can't end with a hyphen or contain two consecutive hyphens.
*
* Example: `myrepinstance`
*/
public val replicationInstanceIdentifier: kotlin.String? = builder.replicationInstanceIdentifier
/**
* A subnet group to associate with the replication instance.
*/
public val replicationSubnetGroupIdentifier: kotlin.String? = builder.replicationSubnetGroupIdentifier
/**
* A friendly name for the resource identifier at the end of the `EndpointArn` response parameter that is returned in the created `Endpoint` object. The value for this parameter can have up to 31 characters. It can contain only ASCII letters, digits, and hyphen ('-'). Also, it can't end with a hyphen or contain two consecutive hyphens, and can only begin with a letter, such as `Example-App-ARN1`. For example, this value might result in the `EndpointArn` value `arn:aws:dms:eu-west-1:012345678901:rep:Example-App-ARN1`. If you don't specify a `ResourceIdentifier` value, DMS generates a default identifier value for the end of `EndpointArn`.
*/
public val resourceIdentifier: kotlin.String? = builder.resourceIdentifier
/**
* One or more tags to be assigned to the replication instance.
*/
public val tags: List? = builder.tags
/**
* Specifies the VPC security group to be used with the replication instance. The VPC security group must work with the VPC containing the replication instance.
*/
public val vpcSecurityGroupIds: List? = builder.vpcSecurityGroupIds
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.databasemigrationservice.model.CreateReplicationInstanceRequest = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("CreateReplicationInstanceRequest(")
append("allocatedStorage=$allocatedStorage,")
append("autoMinorVersionUpgrade=$autoMinorVersionUpgrade,")
append("availabilityZone=$availabilityZone,")
append("dnsNameServers=$dnsNameServers,")
append("engineVersion=$engineVersion,")
append("kerberosAuthenticationSettings=$kerberosAuthenticationSettings,")
append("kmsKeyId=$kmsKeyId,")
append("multiAz=$multiAz,")
append("networkType=$networkType,")
append("preferredMaintenanceWindow=$preferredMaintenanceWindow,")
append("publiclyAccessible=$publiclyAccessible,")
append("replicationInstanceClass=$replicationInstanceClass,")
append("replicationInstanceIdentifier=$replicationInstanceIdentifier,")
append("replicationSubnetGroupIdentifier=$replicationSubnetGroupIdentifier,")
append("resourceIdentifier=$resourceIdentifier,")
append("tags=$tags,")
append("vpcSecurityGroupIds=$vpcSecurityGroupIds")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = allocatedStorage ?: 0
result = 31 * result + (autoMinorVersionUpgrade?.hashCode() ?: 0)
result = 31 * result + (availabilityZone?.hashCode() ?: 0)
result = 31 * result + (dnsNameServers?.hashCode() ?: 0)
result = 31 * result + (engineVersion?.hashCode() ?: 0)
result = 31 * result + (kerberosAuthenticationSettings?.hashCode() ?: 0)
result = 31 * result + (kmsKeyId?.hashCode() ?: 0)
result = 31 * result + (multiAz?.hashCode() ?: 0)
result = 31 * result + (networkType?.hashCode() ?: 0)
result = 31 * result + (preferredMaintenanceWindow?.hashCode() ?: 0)
result = 31 * result + (publiclyAccessible?.hashCode() ?: 0)
result = 31 * result + (replicationInstanceClass?.hashCode() ?: 0)
result = 31 * result + (replicationInstanceIdentifier?.hashCode() ?: 0)
result = 31 * result + (replicationSubnetGroupIdentifier?.hashCode() ?: 0)
result = 31 * result + (resourceIdentifier?.hashCode() ?: 0)
result = 31 * result + (tags?.hashCode() ?: 0)
result = 31 * result + (vpcSecurityGroupIds?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as CreateReplicationInstanceRequest
if (allocatedStorage != other.allocatedStorage) return false
if (autoMinorVersionUpgrade != other.autoMinorVersionUpgrade) return false
if (availabilityZone != other.availabilityZone) return false
if (dnsNameServers != other.dnsNameServers) return false
if (engineVersion != other.engineVersion) return false
if (kerberosAuthenticationSettings != other.kerberosAuthenticationSettings) return false
if (kmsKeyId != other.kmsKeyId) return false
if (multiAz != other.multiAz) return false
if (networkType != other.networkType) return false
if (preferredMaintenanceWindow != other.preferredMaintenanceWindow) return false
if (publiclyAccessible != other.publiclyAccessible) return false
if (replicationInstanceClass != other.replicationInstanceClass) return false
if (replicationInstanceIdentifier != other.replicationInstanceIdentifier) return false
if (replicationSubnetGroupIdentifier != other.replicationSubnetGroupIdentifier) return false
if (resourceIdentifier != other.resourceIdentifier) return false
if (tags != other.tags) return false
if (vpcSecurityGroupIds != other.vpcSecurityGroupIds) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.databasemigrationservice.model.CreateReplicationInstanceRequest = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The amount of storage (in gigabytes) to be initially allocated for the replication instance.
*/
public var allocatedStorage: kotlin.Int? = null
/**
* A value that indicates whether minor engine upgrades are applied automatically to the replication instance during the maintenance window. This parameter defaults to `true`.
*
* Default: `true`
*/
public var autoMinorVersionUpgrade: kotlin.Boolean? = null
/**
* The Availability Zone where the replication instance will be created. The default value is a random, system-chosen Availability Zone in the endpoint's Amazon Web Services Region, for example: `us-east-1d`.
*/
public var availabilityZone: kotlin.String? = null
/**
* A list of custom DNS name servers supported for the replication instance to access your on-premise source or target database. This list overrides the default name servers supported by the replication instance. You can specify a comma-separated list of internet addresses for up to four on-premise DNS name servers. For example: `"1.1.1.1,2.2.2.2,3.3.3.3,4.4.4.4"`
*/
public var dnsNameServers: kotlin.String? = null
/**
* The engine version number of the replication instance.
*
* If an engine version number is not specified when a replication instance is created, the default is the latest engine version available.
*/
public var engineVersion: kotlin.String? = null
/**
* Specifies the ID of the secret that stores the key cache file required for kerberos authentication, when creating a replication instance.
*/
public var kerberosAuthenticationSettings: aws.sdk.kotlin.services.databasemigrationservice.model.KerberosAuthenticationSettings? = null
/**
* An KMS key identifier that is used to encrypt the data on the replication instance.
*
* If you don't specify a value for the `KmsKeyId` parameter, then DMS uses your default encryption key.
*
* KMS creates the default encryption key for your Amazon Web Services account. Your Amazon Web Services account has a different default encryption key for each Amazon Web Services Region.
*/
public var kmsKeyId: kotlin.String? = null
/**
* Specifies whether the replication instance is a Multi-AZ deployment. You can't set the `AvailabilityZone` parameter if the Multi-AZ parameter is set to `true`.
*/
public var multiAz: kotlin.Boolean? = null
/**
* The type of IP address protocol used by a replication instance, such as IPv4 only or Dual-stack that supports both IPv4 and IPv6 addressing. IPv6 only is not yet supported.
*/
public var networkType: kotlin.String? = null
/**
* The weekly time range during which system maintenance can occur, in Universal Coordinated Time (UTC).
*
* Format: `ddd:hh24:mi-ddd:hh24:mi`
*
* Default: A 30-minute window selected at random from an 8-hour block of time per Amazon Web Services Region, occurring on a random day of the week.
*
* Valid Days: Mon, Tue, Wed, Thu, Fri, Sat, Sun
*
* Constraints: Minimum 30-minute window.
*/
public var preferredMaintenanceWindow: kotlin.String? = null
/**
* Specifies the accessibility options for the replication instance. A value of `true` represents an instance with a public IP address. A value of `false` represents an instance with a private IP address. The default value is `true`.
*/
public var publiclyAccessible: kotlin.Boolean? = null
/**
* The compute and memory capacity of the replication instance as defined for the specified replication instance class. For example to specify the instance class dms.c4.large, set this parameter to `"dms.c4.large"`.
*
* For more information on the settings and capacities for the available replication instance classes, see [ Choosing the right DMS replication instance](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_ReplicationInstance.Types.html ); and, [Selecting the best size for a replication instance](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_BestPractices.SizingReplicationInstance.html).
*/
public var replicationInstanceClass: kotlin.String? = null
/**
* The replication instance identifier. This parameter is stored as a lowercase string.
*
* Constraints:
* + Must contain 1-63 alphanumeric characters or hyphens.
* + First character must be a letter.
* + Can't end with a hyphen or contain two consecutive hyphens.
*
* Example: `myrepinstance`
*/
public var replicationInstanceIdentifier: kotlin.String? = null
/**
* A subnet group to associate with the replication instance.
*/
public var replicationSubnetGroupIdentifier: kotlin.String? = null
/**
* A friendly name for the resource identifier at the end of the `EndpointArn` response parameter that is returned in the created `Endpoint` object. The value for this parameter can have up to 31 characters. It can contain only ASCII letters, digits, and hyphen ('-'). Also, it can't end with a hyphen or contain two consecutive hyphens, and can only begin with a letter, such as `Example-App-ARN1`. For example, this value might result in the `EndpointArn` value `arn:aws:dms:eu-west-1:012345678901:rep:Example-App-ARN1`. If you don't specify a `ResourceIdentifier` value, DMS generates a default identifier value for the end of `EndpointArn`.
*/
public var resourceIdentifier: kotlin.String? = null
/**
* One or more tags to be assigned to the replication instance.
*/
public var tags: List? = null
/**
* Specifies the VPC security group to be used with the replication instance. The VPC security group must work with the VPC containing the replication instance.
*/
public var vpcSecurityGroupIds: List? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.databasemigrationservice.model.CreateReplicationInstanceRequest) : this() {
this.allocatedStorage = x.allocatedStorage
this.autoMinorVersionUpgrade = x.autoMinorVersionUpgrade
this.availabilityZone = x.availabilityZone
this.dnsNameServers = x.dnsNameServers
this.engineVersion = x.engineVersion
this.kerberosAuthenticationSettings = x.kerberosAuthenticationSettings
this.kmsKeyId = x.kmsKeyId
this.multiAz = x.multiAz
this.networkType = x.networkType
this.preferredMaintenanceWindow = x.preferredMaintenanceWindow
this.publiclyAccessible = x.publiclyAccessible
this.replicationInstanceClass = x.replicationInstanceClass
this.replicationInstanceIdentifier = x.replicationInstanceIdentifier
this.replicationSubnetGroupIdentifier = x.replicationSubnetGroupIdentifier
this.resourceIdentifier = x.resourceIdentifier
this.tags = x.tags
this.vpcSecurityGroupIds = x.vpcSecurityGroupIds
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.databasemigrationservice.model.CreateReplicationInstanceRequest = CreateReplicationInstanceRequest(this)
/**
* construct an [aws.sdk.kotlin.services.databasemigrationservice.model.KerberosAuthenticationSettings] inside the given [block]
*/
public fun kerberosAuthenticationSettings(block: aws.sdk.kotlin.services.databasemigrationservice.model.KerberosAuthenticationSettings.Builder.() -> kotlin.Unit) {
this.kerberosAuthenticationSettings = aws.sdk.kotlin.services.databasemigrationservice.model.KerberosAuthenticationSettings.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy