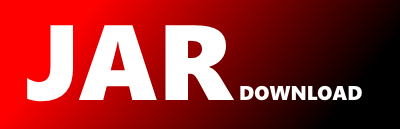
commonMain.aws.sdk.kotlin.services.databasemigrationservice.model.DataMigration.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of databasemigrationservice-jvm Show documentation
Show all versions of databasemigrationservice-jvm Show documentation
The AWS SDK for Kotlin client for Database Migration Service
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.databasemigrationservice.model
import aws.smithy.kotlin.runtime.SdkDsl
import aws.smithy.kotlin.runtime.time.Instant
/**
* This object provides information about a DMS data migration.
*/
public class DataMigration private constructor(builder: Builder) {
/**
* The Amazon Resource Name (ARN) that identifies this replication.
*/
public val dataMigrationArn: kotlin.String? = builder.dataMigrationArn
/**
* The CIDR blocks of the endpoints for the data migration.
*/
public val dataMigrationCidrBlocks: List? = builder.dataMigrationCidrBlocks
/**
* The UTC time when DMS created the data migration.
*/
public val dataMigrationCreateTime: aws.smithy.kotlin.runtime.time.Instant? = builder.dataMigrationCreateTime
/**
* The UTC time when data migration ended.
*/
public val dataMigrationEndTime: aws.smithy.kotlin.runtime.time.Instant? = builder.dataMigrationEndTime
/**
* The user-friendly name for the data migration.
*/
public val dataMigrationName: kotlin.String? = builder.dataMigrationName
/**
* Specifies CloudWatch settings and selection rules for the data migration.
*/
public val dataMigrationSettings: aws.sdk.kotlin.services.databasemigrationservice.model.DataMigrationSettings? = builder.dataMigrationSettings
/**
* The UTC time when DMS started the data migration.
*/
public val dataMigrationStartTime: aws.smithy.kotlin.runtime.time.Instant? = builder.dataMigrationStartTime
/**
* Provides information about the data migration's run, including start and stop time, latency, and data migration progress.
*/
public val dataMigrationStatistics: aws.sdk.kotlin.services.databasemigrationservice.model.DataMigrationStatistics? = builder.dataMigrationStatistics
/**
* The current status of the data migration.
*/
public val dataMigrationStatus: kotlin.String? = builder.dataMigrationStatus
/**
* Specifies whether the data migration is full-load only, change data capture (CDC) only, or full-load and CDC.
*/
public val dataMigrationType: aws.sdk.kotlin.services.databasemigrationservice.model.MigrationTypeValue? = builder.dataMigrationType
/**
* Information about the data migration's most recent error or failure.
*/
public val lastFailureMessage: kotlin.String? = builder.lastFailureMessage
/**
* The Amazon Resource Name (ARN) of the data migration's associated migration project.
*/
public val migrationProjectArn: kotlin.String? = builder.migrationProjectArn
/**
* The IP addresses of the endpoints for the data migration.
*/
public val publicIpAddresses: List? = builder.publicIpAddresses
/**
* The IAM role that the data migration uses to access Amazon Web Services resources.
*/
public val serviceAccessRoleArn: kotlin.String? = builder.serviceAccessRoleArn
/**
* Specifies information about the data migration's source data provider.
*/
public val sourceDataSettings: List? = builder.sourceDataSettings
/**
* The reason the data migration last stopped.
*/
public val stopReason: kotlin.String? = builder.stopReason
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.databasemigrationservice.model.DataMigration = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("DataMigration(")
append("dataMigrationArn=$dataMigrationArn,")
append("dataMigrationCidrBlocks=$dataMigrationCidrBlocks,")
append("dataMigrationCreateTime=$dataMigrationCreateTime,")
append("dataMigrationEndTime=$dataMigrationEndTime,")
append("dataMigrationName=$dataMigrationName,")
append("dataMigrationSettings=$dataMigrationSettings,")
append("dataMigrationStartTime=$dataMigrationStartTime,")
append("dataMigrationStatistics=$dataMigrationStatistics,")
append("dataMigrationStatus=$dataMigrationStatus,")
append("dataMigrationType=$dataMigrationType,")
append("lastFailureMessage=$lastFailureMessage,")
append("migrationProjectArn=$migrationProjectArn,")
append("publicIpAddresses=*** Sensitive Data Redacted ***,")
append("serviceAccessRoleArn=$serviceAccessRoleArn,")
append("sourceDataSettings=$sourceDataSettings,")
append("stopReason=$stopReason")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = dataMigrationArn?.hashCode() ?: 0
result = 31 * result + (dataMigrationCidrBlocks?.hashCode() ?: 0)
result = 31 * result + (dataMigrationCreateTime?.hashCode() ?: 0)
result = 31 * result + (dataMigrationEndTime?.hashCode() ?: 0)
result = 31 * result + (dataMigrationName?.hashCode() ?: 0)
result = 31 * result + (dataMigrationSettings?.hashCode() ?: 0)
result = 31 * result + (dataMigrationStartTime?.hashCode() ?: 0)
result = 31 * result + (dataMigrationStatistics?.hashCode() ?: 0)
result = 31 * result + (dataMigrationStatus?.hashCode() ?: 0)
result = 31 * result + (dataMigrationType?.hashCode() ?: 0)
result = 31 * result + (lastFailureMessage?.hashCode() ?: 0)
result = 31 * result + (migrationProjectArn?.hashCode() ?: 0)
result = 31 * result + (publicIpAddresses?.hashCode() ?: 0)
result = 31 * result + (serviceAccessRoleArn?.hashCode() ?: 0)
result = 31 * result + (sourceDataSettings?.hashCode() ?: 0)
result = 31 * result + (stopReason?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as DataMigration
if (dataMigrationArn != other.dataMigrationArn) return false
if (dataMigrationCidrBlocks != other.dataMigrationCidrBlocks) return false
if (dataMigrationCreateTime != other.dataMigrationCreateTime) return false
if (dataMigrationEndTime != other.dataMigrationEndTime) return false
if (dataMigrationName != other.dataMigrationName) return false
if (dataMigrationSettings != other.dataMigrationSettings) return false
if (dataMigrationStartTime != other.dataMigrationStartTime) return false
if (dataMigrationStatistics != other.dataMigrationStatistics) return false
if (dataMigrationStatus != other.dataMigrationStatus) return false
if (dataMigrationType != other.dataMigrationType) return false
if (lastFailureMessage != other.lastFailureMessage) return false
if (migrationProjectArn != other.migrationProjectArn) return false
if (publicIpAddresses != other.publicIpAddresses) return false
if (serviceAccessRoleArn != other.serviceAccessRoleArn) return false
if (sourceDataSettings != other.sourceDataSettings) return false
if (stopReason != other.stopReason) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.databasemigrationservice.model.DataMigration = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The Amazon Resource Name (ARN) that identifies this replication.
*/
public var dataMigrationArn: kotlin.String? = null
/**
* The CIDR blocks of the endpoints for the data migration.
*/
public var dataMigrationCidrBlocks: List? = null
/**
* The UTC time when DMS created the data migration.
*/
public var dataMigrationCreateTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The UTC time when data migration ended.
*/
public var dataMigrationEndTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* The user-friendly name for the data migration.
*/
public var dataMigrationName: kotlin.String? = null
/**
* Specifies CloudWatch settings and selection rules for the data migration.
*/
public var dataMigrationSettings: aws.sdk.kotlin.services.databasemigrationservice.model.DataMigrationSettings? = null
/**
* The UTC time when DMS started the data migration.
*/
public var dataMigrationStartTime: aws.smithy.kotlin.runtime.time.Instant? = null
/**
* Provides information about the data migration's run, including start and stop time, latency, and data migration progress.
*/
public var dataMigrationStatistics: aws.sdk.kotlin.services.databasemigrationservice.model.DataMigrationStatistics? = null
/**
* The current status of the data migration.
*/
public var dataMigrationStatus: kotlin.String? = null
/**
* Specifies whether the data migration is full-load only, change data capture (CDC) only, or full-load and CDC.
*/
public var dataMigrationType: aws.sdk.kotlin.services.databasemigrationservice.model.MigrationTypeValue? = null
/**
* Information about the data migration's most recent error or failure.
*/
public var lastFailureMessage: kotlin.String? = null
/**
* The Amazon Resource Name (ARN) of the data migration's associated migration project.
*/
public var migrationProjectArn: kotlin.String? = null
/**
* The IP addresses of the endpoints for the data migration.
*/
public var publicIpAddresses: List? = null
/**
* The IAM role that the data migration uses to access Amazon Web Services resources.
*/
public var serviceAccessRoleArn: kotlin.String? = null
/**
* Specifies information about the data migration's source data provider.
*/
public var sourceDataSettings: List? = null
/**
* The reason the data migration last stopped.
*/
public var stopReason: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.databasemigrationservice.model.DataMigration) : this() {
this.dataMigrationArn = x.dataMigrationArn
this.dataMigrationCidrBlocks = x.dataMigrationCidrBlocks
this.dataMigrationCreateTime = x.dataMigrationCreateTime
this.dataMigrationEndTime = x.dataMigrationEndTime
this.dataMigrationName = x.dataMigrationName
this.dataMigrationSettings = x.dataMigrationSettings
this.dataMigrationStartTime = x.dataMigrationStartTime
this.dataMigrationStatistics = x.dataMigrationStatistics
this.dataMigrationStatus = x.dataMigrationStatus
this.dataMigrationType = x.dataMigrationType
this.lastFailureMessage = x.lastFailureMessage
this.migrationProjectArn = x.migrationProjectArn
this.publicIpAddresses = x.publicIpAddresses
this.serviceAccessRoleArn = x.serviceAccessRoleArn
this.sourceDataSettings = x.sourceDataSettings
this.stopReason = x.stopReason
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.databasemigrationservice.model.DataMigration = DataMigration(this)
/**
* construct an [aws.sdk.kotlin.services.databasemigrationservice.model.DataMigrationSettings] inside the given [block]
*/
public fun dataMigrationSettings(block: aws.sdk.kotlin.services.databasemigrationservice.model.DataMigrationSettings.Builder.() -> kotlin.Unit) {
this.dataMigrationSettings = aws.sdk.kotlin.services.databasemigrationservice.model.DataMigrationSettings.invoke(block)
}
/**
* construct an [aws.sdk.kotlin.services.databasemigrationservice.model.DataMigrationStatistics] inside the given [block]
*/
public fun dataMigrationStatistics(block: aws.sdk.kotlin.services.databasemigrationservice.model.DataMigrationStatistics.Builder.() -> kotlin.Unit) {
this.dataMigrationStatistics = aws.sdk.kotlin.services.databasemigrationservice.model.DataMigrationStatistics.invoke(block)
}
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy