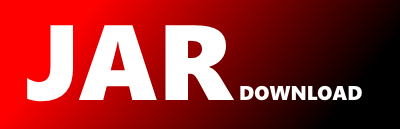
commonMain.aws.sdk.kotlin.services.databasemigrationservice.model.DatabaseInstanceSoftwareDetailsResponse.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of databasemigrationservice-jvm Show documentation
Show all versions of databasemigrationservice-jvm Show documentation
The AWS SDK for Kotlin client for Database Migration Service
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.databasemigrationservice.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Describes an inventory database instance for a Fleet Advisor collector.
*/
public class DatabaseInstanceSoftwareDetailsResponse private constructor(builder: Builder) {
/**
* The database engine of a database in a Fleet Advisor collector inventory, for example `Microsoft SQL Server`.
*/
public val engine: kotlin.String? = builder.engine
/**
* The database engine edition of a database in a Fleet Advisor collector inventory, for example `Express`.
*/
public val engineEdition: kotlin.String? = builder.engineEdition
/**
* The database engine version of a database in a Fleet Advisor collector inventory, for example `2019`.
*/
public val engineVersion: kotlin.String? = builder.engineVersion
/**
* The operating system architecture of the database.
*/
public val osArchitecture: kotlin.Int? = builder.osArchitecture
/**
* The service pack level of the database.
*/
public val servicePack: kotlin.String? = builder.servicePack
/**
* The support level of the database, for example `Mainstream support`.
*/
public val supportLevel: kotlin.String? = builder.supportLevel
/**
* Information about the database engine software, for example `Mainstream support ends on November 14th, 2024`.
*/
public val tooltip: kotlin.String? = builder.tooltip
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.databasemigrationservice.model.DatabaseInstanceSoftwareDetailsResponse = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("DatabaseInstanceSoftwareDetailsResponse(")
append("engine=$engine,")
append("engineEdition=$engineEdition,")
append("engineVersion=$engineVersion,")
append("osArchitecture=$osArchitecture,")
append("servicePack=$servicePack,")
append("supportLevel=$supportLevel,")
append("tooltip=$tooltip")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = engine?.hashCode() ?: 0
result = 31 * result + (engineEdition?.hashCode() ?: 0)
result = 31 * result + (engineVersion?.hashCode() ?: 0)
result = 31 * result + (osArchitecture ?: 0)
result = 31 * result + (servicePack?.hashCode() ?: 0)
result = 31 * result + (supportLevel?.hashCode() ?: 0)
result = 31 * result + (tooltip?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as DatabaseInstanceSoftwareDetailsResponse
if (engine != other.engine) return false
if (engineEdition != other.engineEdition) return false
if (engineVersion != other.engineVersion) return false
if (osArchitecture != other.osArchitecture) return false
if (servicePack != other.servicePack) return false
if (supportLevel != other.supportLevel) return false
if (tooltip != other.tooltip) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.databasemigrationservice.model.DatabaseInstanceSoftwareDetailsResponse = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The database engine of a database in a Fleet Advisor collector inventory, for example `Microsoft SQL Server`.
*/
public var engine: kotlin.String? = null
/**
* The database engine edition of a database in a Fleet Advisor collector inventory, for example `Express`.
*/
public var engineEdition: kotlin.String? = null
/**
* The database engine version of a database in a Fleet Advisor collector inventory, for example `2019`.
*/
public var engineVersion: kotlin.String? = null
/**
* The operating system architecture of the database.
*/
public var osArchitecture: kotlin.Int? = null
/**
* The service pack level of the database.
*/
public var servicePack: kotlin.String? = null
/**
* The support level of the database, for example `Mainstream support`.
*/
public var supportLevel: kotlin.String? = null
/**
* Information about the database engine software, for example `Mainstream support ends on November 14th, 2024`.
*/
public var tooltip: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.databasemigrationservice.model.DatabaseInstanceSoftwareDetailsResponse) : this() {
this.engine = x.engine
this.engineEdition = x.engineEdition
this.engineVersion = x.engineVersion
this.osArchitecture = x.osArchitecture
this.servicePack = x.servicePack
this.supportLevel = x.supportLevel
this.tooltip = x.tooltip
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.databasemigrationservice.model.DatabaseInstanceSoftwareDetailsResponse = DatabaseInstanceSoftwareDetailsResponse(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy