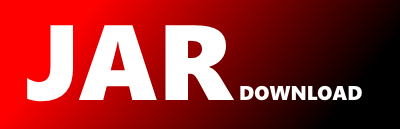
commonMain.aws.sdk.kotlin.services.databasemigrationservice.model.ElasticsearchSettings.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of databasemigrationservice-jvm Show documentation
Show all versions of databasemigrationservice-jvm Show documentation
The AWS SDK for Kotlin client for Database Migration Service
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.databasemigrationservice.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Provides information that defines an OpenSearch endpoint.
*/
public class ElasticsearchSettings private constructor(builder: Builder) {
/**
* The endpoint for the OpenSearch cluster. DMS uses HTTPS if a transport protocol (http/https) is not specified.
*/
public val endpointUri: kotlin.String = requireNotNull(builder.endpointUri) { "A non-null value must be provided for endpointUri" }
/**
* The maximum number of seconds for which DMS retries failed API requests to the OpenSearch cluster.
*/
public val errorRetryDuration: kotlin.Int? = builder.errorRetryDuration
/**
* The maximum percentage of records that can fail to be written before a full load operation stops.
*
* To avoid early failure, this counter is only effective after 1000 records are transferred. OpenSearch also has the concept of error monitoring during the last 10 minutes of an Observation Window. If transfer of all records fail in the last 10 minutes, the full load operation stops.
*/
public val fullLoadErrorPercentage: kotlin.Int? = builder.fullLoadErrorPercentage
/**
* The Amazon Resource Name (ARN) used by the service to access the IAM role. The role must allow the `iam:PassRole` action.
*/
public val serviceAccessRoleArn: kotlin.String = requireNotNull(builder.serviceAccessRoleArn) { "A non-null value must be provided for serviceAccessRoleArn" }
/**
* Set this option to `true` for DMS to migrate documentation using the documentation type `_doc`. OpenSearch and an Elasticsearch cluster only support the _doc documentation type in versions 7. x and later. The default value is `false`.
*/
public val useNewMappingType: kotlin.Boolean? = builder.useNewMappingType
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.databasemigrationservice.model.ElasticsearchSettings = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("ElasticsearchSettings(")
append("endpointUri=$endpointUri,")
append("errorRetryDuration=$errorRetryDuration,")
append("fullLoadErrorPercentage=$fullLoadErrorPercentage,")
append("serviceAccessRoleArn=$serviceAccessRoleArn,")
append("useNewMappingType=$useNewMappingType")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = endpointUri.hashCode()
result = 31 * result + (errorRetryDuration ?: 0)
result = 31 * result + (fullLoadErrorPercentage ?: 0)
result = 31 * result + (serviceAccessRoleArn.hashCode())
result = 31 * result + (useNewMappingType?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as ElasticsearchSettings
if (endpointUri != other.endpointUri) return false
if (errorRetryDuration != other.errorRetryDuration) return false
if (fullLoadErrorPercentage != other.fullLoadErrorPercentage) return false
if (serviceAccessRoleArn != other.serviceAccessRoleArn) return false
if (useNewMappingType != other.useNewMappingType) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.databasemigrationservice.model.ElasticsearchSettings = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The endpoint for the OpenSearch cluster. DMS uses HTTPS if a transport protocol (http/https) is not specified.
*/
public var endpointUri: kotlin.String? = null
/**
* The maximum number of seconds for which DMS retries failed API requests to the OpenSearch cluster.
*/
public var errorRetryDuration: kotlin.Int? = null
/**
* The maximum percentage of records that can fail to be written before a full load operation stops.
*
* To avoid early failure, this counter is only effective after 1000 records are transferred. OpenSearch also has the concept of error monitoring during the last 10 minutes of an Observation Window. If transfer of all records fail in the last 10 minutes, the full load operation stops.
*/
public var fullLoadErrorPercentage: kotlin.Int? = null
/**
* The Amazon Resource Name (ARN) used by the service to access the IAM role. The role must allow the `iam:PassRole` action.
*/
public var serviceAccessRoleArn: kotlin.String? = null
/**
* Set this option to `true` for DMS to migrate documentation using the documentation type `_doc`. OpenSearch and an Elasticsearch cluster only support the _doc documentation type in versions 7. x and later. The default value is `false`.
*/
public var useNewMappingType: kotlin.Boolean? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.databasemigrationservice.model.ElasticsearchSettings) : this() {
this.endpointUri = x.endpointUri
this.errorRetryDuration = x.errorRetryDuration
this.fullLoadErrorPercentage = x.fullLoadErrorPercentage
this.serviceAccessRoleArn = x.serviceAccessRoleArn
this.useNewMappingType = x.useNewMappingType
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.databasemigrationservice.model.ElasticsearchSettings = ElasticsearchSettings(this)
internal fun correctErrors(): Builder {
if (endpointUri == null) endpointUri = ""
if (serviceAccessRoleArn == null) serviceAccessRoleArn = ""
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy