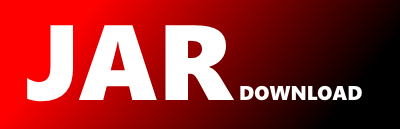
commonMain.aws.sdk.kotlin.services.databasemigrationservice.model.EndpointSetting.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of databasemigrationservice-jvm Show documentation
Show all versions of databasemigrationservice-jvm Show documentation
The AWS SDK for Kotlin client for Database Migration Service
The newest version!
// Code generated by smithy-kotlin-codegen. DO NOT EDIT!
package aws.sdk.kotlin.services.databasemigrationservice.model
import aws.smithy.kotlin.runtime.SdkDsl
/**
* Endpoint settings.
*/
public class EndpointSetting private constructor(builder: Builder) {
/**
* The relevance or validity of an endpoint setting for an engine name and its endpoint type.
*/
public val applicability: kotlin.String? = builder.applicability
/**
* The default value of the endpoint setting if no value is specified using `CreateEndpoint` or `ModifyEndpoint`.
*/
public val defaultValue: kotlin.String? = builder.defaultValue
/**
* Enumerated values to use for this endpoint.
*/
public val enumValues: List? = builder.enumValues
/**
* The maximum value of an endpoint setting that is of type `int`.
*/
public val intValueMax: kotlin.Int? = builder.intValueMax
/**
* The minimum value of an endpoint setting that is of type `int`.
*/
public val intValueMin: kotlin.Int? = builder.intValueMin
/**
* The name that you want to give the endpoint settings.
*/
public val name: kotlin.String? = builder.name
/**
* A value that marks this endpoint setting as sensitive.
*/
public val sensitive: kotlin.Boolean? = builder.sensitive
/**
* The type of endpoint. Valid values are `source` and `target`.
*/
public val type: aws.sdk.kotlin.services.databasemigrationservice.model.EndpointSettingTypeValue? = builder.type
/**
* The unit of measure for this endpoint setting.
*/
public val units: kotlin.String? = builder.units
public companion object {
public operator fun invoke(block: Builder.() -> kotlin.Unit): aws.sdk.kotlin.services.databasemigrationservice.model.EndpointSetting = Builder().apply(block).build()
}
override fun toString(): kotlin.String = buildString {
append("EndpointSetting(")
append("applicability=$applicability,")
append("defaultValue=$defaultValue,")
append("enumValues=$enumValues,")
append("intValueMax=$intValueMax,")
append("intValueMin=$intValueMin,")
append("name=$name,")
append("sensitive=$sensitive,")
append("type=$type,")
append("units=$units")
append(")")
}
override fun hashCode(): kotlin.Int {
var result = applicability?.hashCode() ?: 0
result = 31 * result + (defaultValue?.hashCode() ?: 0)
result = 31 * result + (enumValues?.hashCode() ?: 0)
result = 31 * result + (intValueMax ?: 0)
result = 31 * result + (intValueMin ?: 0)
result = 31 * result + (name?.hashCode() ?: 0)
result = 31 * result + (sensitive?.hashCode() ?: 0)
result = 31 * result + (type?.hashCode() ?: 0)
result = 31 * result + (units?.hashCode() ?: 0)
return result
}
override fun equals(other: kotlin.Any?): kotlin.Boolean {
if (this === other) return true
if (other == null || this::class != other::class) return false
other as EndpointSetting
if (applicability != other.applicability) return false
if (defaultValue != other.defaultValue) return false
if (enumValues != other.enumValues) return false
if (intValueMax != other.intValueMax) return false
if (intValueMin != other.intValueMin) return false
if (name != other.name) return false
if (sensitive != other.sensitive) return false
if (type != other.type) return false
if (units != other.units) return false
return true
}
public inline fun copy(block: Builder.() -> kotlin.Unit = {}): aws.sdk.kotlin.services.databasemigrationservice.model.EndpointSetting = Builder(this).apply(block).build()
@SdkDsl
public class Builder {
/**
* The relevance or validity of an endpoint setting for an engine name and its endpoint type.
*/
public var applicability: kotlin.String? = null
/**
* The default value of the endpoint setting if no value is specified using `CreateEndpoint` or `ModifyEndpoint`.
*/
public var defaultValue: kotlin.String? = null
/**
* Enumerated values to use for this endpoint.
*/
public var enumValues: List? = null
/**
* The maximum value of an endpoint setting that is of type `int`.
*/
public var intValueMax: kotlin.Int? = null
/**
* The minimum value of an endpoint setting that is of type `int`.
*/
public var intValueMin: kotlin.Int? = null
/**
* The name that you want to give the endpoint settings.
*/
public var name: kotlin.String? = null
/**
* A value that marks this endpoint setting as sensitive.
*/
public var sensitive: kotlin.Boolean? = null
/**
* The type of endpoint. Valid values are `source` and `target`.
*/
public var type: aws.sdk.kotlin.services.databasemigrationservice.model.EndpointSettingTypeValue? = null
/**
* The unit of measure for this endpoint setting.
*/
public var units: kotlin.String? = null
@PublishedApi
internal constructor()
@PublishedApi
internal constructor(x: aws.sdk.kotlin.services.databasemigrationservice.model.EndpointSetting) : this() {
this.applicability = x.applicability
this.defaultValue = x.defaultValue
this.enumValues = x.enumValues
this.intValueMax = x.intValueMax
this.intValueMin = x.intValueMin
this.name = x.name
this.sensitive = x.sensitive
this.type = x.type
this.units = x.units
}
@PublishedApi
internal fun build(): aws.sdk.kotlin.services.databasemigrationservice.model.EndpointSetting = EndpointSetting(this)
internal fun correctErrors(): Builder {
return this
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy